Introduction
Part 1
Part 2
MSDN Reference
Just like C, C++ and Java, C# also provides some escape characters as shown below.
(i) \' : Inserts a single quote into a string literal.
(ii) \" : Inserts a double quote into a string literal.
(iii) \\ : Inserts a backslash into a string literal. This can be quite helpful when defining file paths.
(iv) \a : Triggers a system alert (beep). For console applications, this can be an audio clue to the user.
(v) \b : Triggers a backspace.
(vi) \f : Triggers from feed.
(vii) \n : Inserts a new line on Win32 platforms.
(viii) \r : Inserts a carriage return.
(ix) \t : Inserts a horizontal tab into the string literal.
(x) \u : Inserts a Unicode character into the string literal.
(xi) \v : Inserts a vertical tab into the string literal.
(xii) \0 : Represents NULL character.
In addition to escape characters, C# provides the @ quote string literal notation called as 'verbatim string'. Using this notation, we can bypass the use of escape characters and define our literals.
At compile time, verbatim strings are converted to ordinary strings with all the same escape sequences. Therefore, if you view a verbatim string in the debugger watch window, you will see the escape characters that were added by the compiler, not the verbatim version from your source code. For example, the verbatim string @"C:\inetpub\wwwroot\" will appear in the watch window as "C:\\inetpub\\wwwroot\\".
Let's look at a program and its output to understand this well.
using System;
using System.Collections.Generic;
using System.Linq;
namespace ConsoleApplication1
{
class Program
{
static void Main(string[] args)
{
string str1 = "\"C#\" Language is very easy, isn't this?";
//if you use just "C#" will pop error
Console.WriteLine(str1);
string str2 = "C:\\inetpub\\wwwroot\\";
//if you use just \ will pop error
Console.WriteLine(str2);
string str3 = @"C:\inetpub\wwwroot\";
//using verbatim string
Console.WriteLine(str3);
string str4 = @"<script language='javascript'>";
//using verbatim string
Console.WriteLine(str4);
string str5 = "Hello \aAbhimanyu";
//beep
Console.WriteLine(str5);
Console.ReadKey();
}
}
}
The above code will produce the following output:
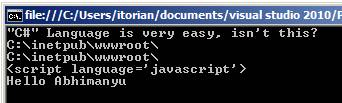
Thank you for reading.
HAVE A HAPPY CODING!!