Introduction
LinkedList<T> represents the doubly linked list, where T is any specific datatype. It represents the collection of nodes, and each node contains an element. Each node is linked to the preceding node and the following node. The doubly linked list only knows the first and the last node. There are various operations that we can perform in the LinkedList.
In this article, I explain how to create a LinkedList and how we perform the operations on a LinkedList. For this, the following steps are used.
Step 1. Open Visual Studio 2010 and click on File->New->Project
click on the Console application. Give the name of the project as LinkedList.
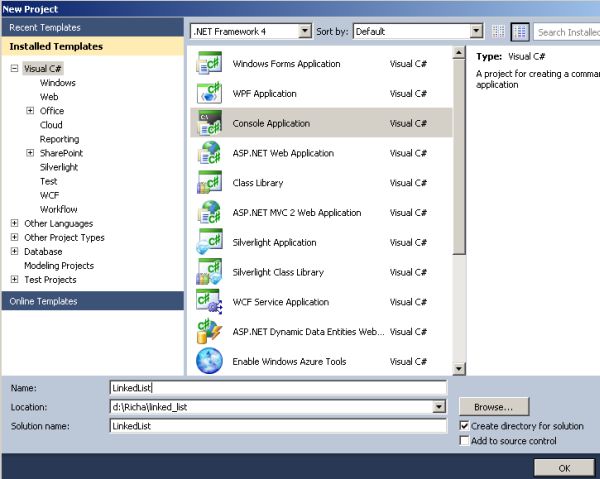
Step 2. Creation of the LinkedList
The LinkedList can be created as.
using System;
using System.Collections.Generic;
namespace LinkedListExample
{
class Program
{
static void Main(string[] args)
{
// Creation of an empty linked list
LinkedList<string> linkedList = new LinkedList<string>();
string[] courses = { "MCA", "BCA", "MBA", "BBA", "BTech", "MTech" };
// Creation of a linked list with initial elements
LinkedList<string> linkedlist1 = new LinkedList<string>(courses);
// Your code here
}
}
}
Some of the methods that we use for adding the elements to the list are.
- AddFirst(): It adds the node at the first position in the LinkedList.
- AddLast(): It adds the node at the last position in the LinkedList.
- AddBefore(): It adds the node before the given node.
- AddAfter(): It adds the node after the given node.
Now to add the nodes on the empty list that I created, named LinkedList, write the following code.
using System;
using System.Collections.Generic;
namespace LinkedListExample
{
class Program
{
static void Main(string[] args)
{
// Creation of an empty linked list
LinkedList<string> linkedList = new LinkedList<string>();
// Add an element at the first position
linkedList.AddFirst("one");
// Add a node at the last position
var nodeThree = linkedList.AddLast("three");
// Add a node before the given element
linkedList.AddBefore(nodeThree, "two");
// Add a node after the given node
linkedList.AddAfter(nodeThree, "four");
// Your code here
}
}
}
Display both the list
To display both lists, write the code as.
using System;
using System.Collections.Generic;
namespace LinkedListExample
{
class Program
{
static void Main(string[] args)
{
// Creation of an empty linked list
LinkedList<string> linkedList = new LinkedList<string>();
string[] courses = { "MCA", "BCA", "MBA", "BBA", "BTech", "MTech" };
// Creation of a linked list with initial elements
LinkedList<string> linkedlist1 = new LinkedList<string>(courses);
// Add an element at the first position
linkedList.AddFirst("one");
// Add a node at the last position
var nodeThree = linkedList.AddLast("three");
// Add a node before the given element
linkedList.AddBefore(nodeThree, "two");
// Add a node after the given node
linkedList.AddAfter(nodeThree, "four");
// Display the first list (linkedList)
Console.WriteLine("The first list is as:");
foreach (string value in linkedList)
{
Console.WriteLine(value);
}
// Display the second list (linkedlist1)
Console.WriteLine("The second list is as:");
foreach (string list in linkedlist1)
{
Console.WriteLine(list);
}
}
}
}
Output
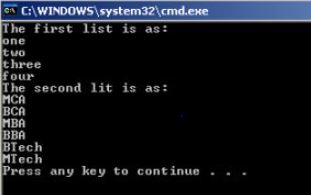
Step 3. Perform various operations on the linked list
- Count(): Count () is used to count the number of nodes/or elements in the linked list.
Example
using System;
using System.Collections.Generic;
using System.Linq; // Added to use the Count() method
namespace LinkedListExample
{
class Program
{
static void Main(string[] args)
{
// Creation of an empty linked list
LinkedList<string> linkedList = new LinkedList<string>();
// Add an element at the first position
linkedList.AddFirst("one");
// Add a node at the last position
var nodeThree = linkedList.AddLast("three");
// Add a node before the given element
linkedList.AddBefore(nodeThree, "two");
// Add a node after the given node
linkedList.AddAfter(nodeThree, "four");
// Display the list (linkedList)
Console.WriteLine("The first list is as:");
foreach (string value in linkedList)
{
Console.WriteLine(value);
}
// Count the number of elements in the list
int i = linkedList.Count();
Console.WriteLine("The elements in the list are: " + i);
}
}
}
Output
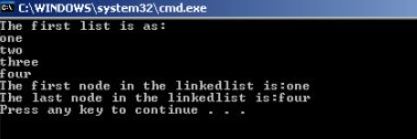
- First(): The First() method is used to get the first node in the LinkedList.
- Last(): The Last() method is used to get the last node in the LinkedList.
Example
using System;
using System.Collections.Generic;
namespace LinkedListExample
{
class Program
{
static void Main(string[] args)
{
// Creation of an empty linked list
LinkedList<string> linkedList = new LinkedList<string>();
// Add an element at the first position
linkedList.AddFirst("one");
// Add a node at the last position
var nodeThree = linkedList.AddLast("three");
// Add a node before the given element
linkedList.AddBefore(nodeThree, "two");
// Add a node after the given node
linkedList.AddAfter(nodeThree, "four");
// Display the list (linkedList)
Console.WriteLine("The first list is as:");
foreach (string value in linkedList)
{
Console.WriteLine(value);
}
// Display the first node in the linked list
Console.WriteLine("The first node in the linked list is: " + linkedList.First());
// Display the last node in the linked list
Console.WriteLine("The last node in the linked list is: " + linkedList.Last());
}
}
}
Output
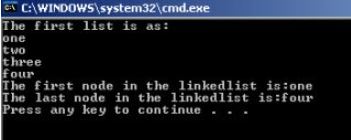
- Contains(): It determines whether the node is contained in the list or not. If yes, then it gives true otherwise false.
- Remove(): Removes the first occurrence of the specified value from the LinkedList<T>.
- RemoveFirst(): Removes the node at the start of the LinkedList<T>.
- RemoveLast(): Removes the node at the end of the LinkedList<T>.
- Clear(): It clears the list.
Example
using System;
using System.Collections.Generic;
namespace LinkedListExample
{
class Program
{
static void Main(string[] args)
{
// Creation of an empty linked list
LinkedList<string> linkedList = new LinkedList<string>();
// Add an element at the first position
linkedList.AddFirst("one");
// Add a node at the last position
var nodeThree = linkedList.AddLast("three");
// Add a node before the given element
linkedList.AddBefore(nodeThree, "two");
// Add a node after the given node
linkedList.AddAfter(nodeThree, "four");
// Display the list (linkedList)
Console.WriteLine("The first list is as follows:");
foreach (string value in linkedList)
{
Console.WriteLine(value);
}
// Use the Contains() method to check if nodes are present in the list
Console.WriteLine("The node 'one' is in the list: " + linkedList.Contains("one"));
Console.WriteLine("The node 'six' is in the list: " + linkedList.Contains("six"));
// Remove a specific node from the list
linkedList.Remove("three");
// Remove the first node from the list
linkedList.RemoveFirst();
// Remove the last node from the list
linkedList.RemoveLast();
// Display the modified list
Console.WriteLine("The list after removal is as follows:");
foreach (string value in linkedList)
{
Console.WriteLine(value);
}
// Clear the list
linkedList.Clear();
Console.WriteLine("The list is now empty.");
foreach (string value in linkedList)
{
Console.WriteLine(value);
}
}
}
}
Output
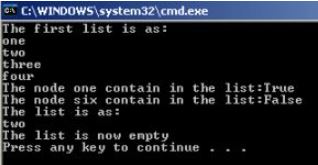
Summary
In this article, I explained how to create a LinkedList and the various operations on the LinkedList.