In every system, there may be some important files to make system work properly. Suppose, you shared such important folder and you went to some other place. Suddenly, your friend calls you and tell that system is not working properly. The most possible reason may be he deleted some important files. How can you know, which files he deleted and at what time. You are away from your system, so you can't even find files which are deleted. So to overcome such situation, I created an application which will send a mail to you, whenever any important files are deleted, renamed or its contents changed. The message sent to you, will clearly tell files update status.
I developed this service in C# in VS 2003.
Create a Windows Service in C# and name it as filesystemwatcher as shown below:
Add reference to System.Web.dll by going to Solution Explorer, clicking on References(->Add Reference) as shown below:
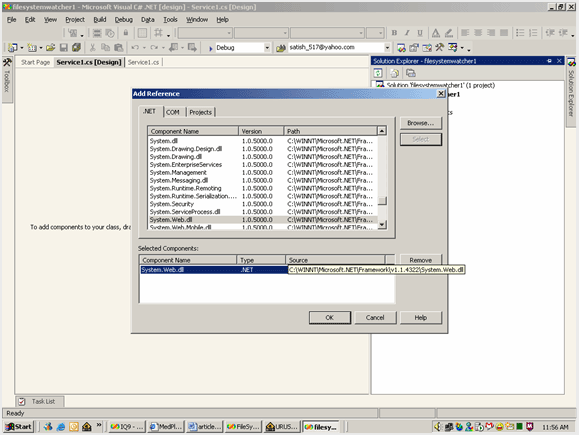
Next go to code window, find "Service1" and replace with filesystemwatcher in all places. Next go to design view, select properties and set Autolog to false and ServiceName to filesystemwatcher.
Next keep a Timer control by dragging it from Components tab of Toolbar.
Next add Installer, by right clicking on design window as shown in figure:
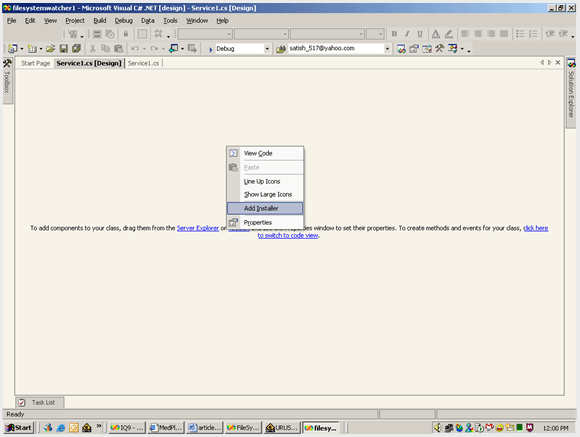
Next go to properties of ServiceInstaller1, and set StartType to Automatic and DisplayName and ServiceName to filesystemwatcher.
Next goto properties of ServiceProcessInstaller1, and set Account to LocalSystem.
Next goto Timer Properties window, and set enabled to true, Interval to 60000.(1 Minute).
Next goto code window and add this statements there:
using System.IO;
using System.Web;
Paste the below code in the timer1_Elapsed event of the Timer control:
DirectoryInfo dir=new DirectoryInfo(@"c:\satish1");
foreach(FileInfo f in dir.GetFiles())
{
TimeSpan diff=(DateTime.Now-f.LastWriteTime);
if(diff.Hours==0 && diff.Minutes<=1)
{
System.Web.Mail.MailMessage message=new System.Web.Mail.MailMessage();
message.To ="[email protected]";
message.From ="[email protected]";
message.Subject ="File Contents Changed "+f.FullName.ToString();
message.Body ="The Following File Contents are Changed " + Path.GetFileName(f.FullName.ToString())+ " of size " + f.Length.ToString()+" at "+DateTime.Now.ToLongTimeString()+" by " +System.Environment.UserName.ToString();
System.Web.Mail.SmtpMail.Send(message);
}
}
The above code will send a mail, whenever a file content is changed in c:\satish1 folder.
This code will check the specified folder for every minute and send a mail, if it contents are changed within that timespan.
Next go to OnStart event and paste this code:
string dirname=@"c:\satish1";
//string dirname=@"c:\"; to monitor entire C: drive....
watch=new System.IO.FileSystemWatcher(dirname,"*.*");
watch.Created +=new FileSystemEventHandler(this.FileCreated);
watch.Deleted +=new FileSystemEventHandler(this.FileDeleted);
watch.Renamed +=new RenamedEventHandler(this.FileRenamed);
watch.IncludeSubdirectories=true;
watch.EnableRaisingEvents=true;
This will create a FileSystemWatcherobject for monitoring folder. I created 3 methods to handle create, delete and rename of a file. I specified that handlers in OnStart event.
Next paste this methods in code window:
public void FileCreated(object sender, FileSystemEventArgs e1)
{
System.Web.Mail.MailMessage message=new System.Web.Mail.MailMessage();
message.To ="[email protected]";
message.From ="[email protected]";
message.Subject ="File Created "+e1.FullPath.ToString();
message.Body ="The Following File is Created "+Path.GetFileName(e1.FullPath.ToString())+" in "+Path.GetDirectoryName(e1.FullPath.ToString())+ " at "+DateTime.Now.ToLongTimeString()+" by " +System.Environment.UserName.ToString();
System.Web.Mail.SmtpMail.Send(message);
}
public void FileDeleted(object sender, FileSystemEventArgs e1)
{
System.Web.Mail.MailMessage message=new System.Web.Mail.MailMessage();
message.To ="[email protected]";
message.From ="[email protected]";
message.Subject ="File Deleted "+e1.FullPath.ToString();
message.Body ="The Following File is Deleted "+Path.GetFileName(e1.FullPath.ToString())+" in "+Path.GetDirectoryName(e1.FullPath.ToString()) + " at "+DateTime.Now.ToLongTimeString()+" by " +System.Environment.UserName.ToString();
System.Web.Mail.SmtpMail.Send(message);
}
public void FileRenamed(object sender, RenamedEventArgs e1)
{
System.Web.Mail.MailMessage message=new System.Web.Mail.MailMessage();
message.To ="[email protected]";
message.From ="[email protected]";
message.Subject ="File Renamed "+e1.OldFullPath.ToString();
message.Body ="The Following file is Renamed from " + e1.OldName.ToString()+ " "+ "To " + e1.Name.ToString()+" in "+Path.GetFileName(e1.FullPath.ToString())+ " at "+DateTime.Now.ToLongTimeString()+" by " +System.Environment.UserName.ToString();
System.Web.Mail.SmtpMail.Send(message);
}
Change the name of folder according to your requirement in OnStart and in timer1_elapsed event.
Each method handle creation, deletion and rename of a file and send a mail to [email protected] with required information of file.
Next, paste this code in OnStop event:
watch.Dispose();
timer1.Enabled=false;
Next build solution by pressing Ctrl+Shift+B. If build is succeeded, then go to command prompt of VS Tools.
Go to debug folder of your solution and type
InstallUtil filesystemwatcher.exe
If commit phase is succeeded, then type this to start service.
net start filesystemwatcher.
Change the folder contents, you specified and see whether a mail will come or not.
I hope this code will be useful to all. I am attaching code for further assistance.