The lock keyword in C# provides good control over a multi-threaded application.
But if we are using it in an unwanted scenario it could impact performance. Sometimes, the usage of threading itself would not be beneficial.
I am trying to explain a valid scenario where lock is advised in a multi-threaded application.
Lock while writing to a file - Advisable
In a multithreaded environment, if a particular method is writing to a file, it is advisable to use lock – otherwise an exception will occur.
private void WriteToFile_Locked()
{
lock (this)
{
StreamWriter streamWriter = File.AppendText("File.txt");
streamWriter.WriteLine("A line of text..");
streamWriter.Close();
}
}
Lock while fetching a network resource – Not Advisable
Suppose a method relys on a network resource such as copying a file over a network or downloading a file from the internet. In this case the lock would degrade the performance since all the threads will be waiting to enter the critical section.
If we use a lock in the above scenario we will not be benefiting from the threading advantage.
private void ReadNetworkResource()
{
WebClient client = new WebClient();
var result = client.DownloadData("http://www.google.com");
}
If we implement a lock in the method above then it will degrade performance.
Test Application
A test application is provided to check the performance in both the above scenarios.
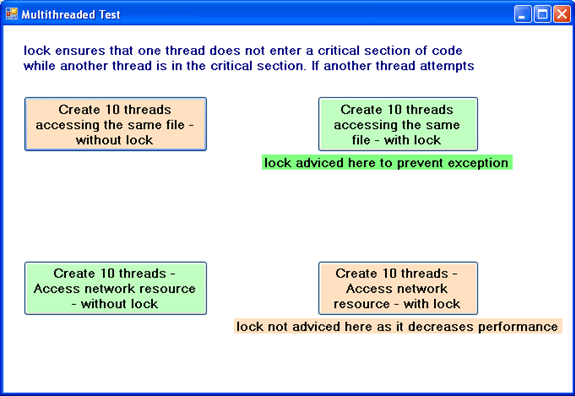
The application contains buttons which are self explanatory. The first row of buttons deals with the file issue and it is solved by implementing the lock keyword.
The second row of buttons deal with the network resource issue where lock is implemented and performance was degraded. On clicking the buttons we can see the time difference it is taking to perform the actions.
In the test machine it took 10 seconds for the first one and 25 seconds for the second.
This shows that if a lock is not wisely used it could impact the performance in a multi threaded application.
Without Lock |
With Lock |
10 seconds |
25 seconds |
About the Source
The source for the above application is attached with the article.