In this article I am going to share some tips on building a user interface to display hierarchical data in an ASP.NET application without using server side controls. This type of functionality can be easily built by using HTML, Ajax, jQuery and ASP.NET MVC framework. We will have to build our own controls for rendering the hierarchical data.
Here are the steps for doing so.
1) Install MVC framework and create a new project selecting ASP.NET MVC Web application.
2) Let's consider following screen shots as requirements. Here assume that Top level table contains the data related to Types of Exams that a person has to take. On selecting a particular type the list of available exams of that type are displayed under it. Then you can drill down further and find out list of people who have cleared that exam in our organization or division.
- Tables having 2 row and hyperlinks on items in each row. On click of hyperlink a new child table will be injected under the selected parent.

Image 1
- On Click of Business Functions hyperlink you get the screen shot as below
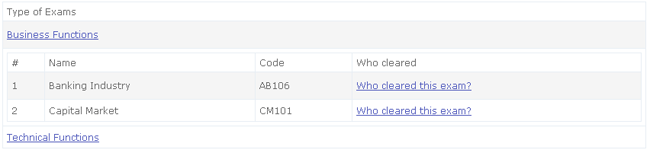
Image 2
- On Click of "Who cleared this exam?" hyperlink you got the following screen shot
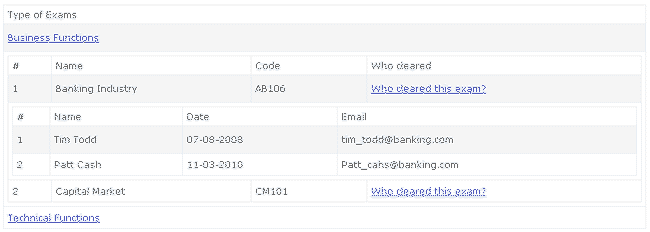
Image 3
- Similar functionality will be seen on Click of the hyperlink on "Technical Functions"
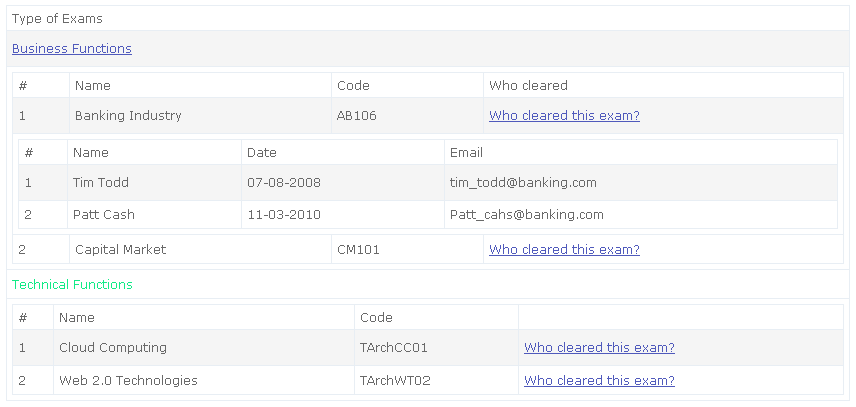
Image 4
- Now that we have defined goal to build the above screen layout without using server side controls let's see how it can be achieved.
3) In the new ASP.NET MVC project you will get a
- Default Controller (in Controllers folder) --> HomeController.cs
- Couple of views (In Views/Home folder) --> Index.aspx
- A Master page for the site (in Views/Shared folder) --> Site.Master
- Files required for jQuery framework in the Scripts folder. (jquery-1.3.2.min.js)
- CSS file in the Contents folder --> Site.css
If we run the project we can set a running website with ASP.NET MVC Framework and jQuery framework being integrated into it by default. We can see the welcome to ASP.NET MVC message on the Index page when we run the project. There are few other controllers and views added to the project by default which I have not mentioned here for simplicity.
4) Here, I am assuming that reader is able to understand how an ASP.NET MVC application works. Also he knows how to write HTML and JavaScript. Also the reader should be able to understand the concepts of Ajax technologies. So with all this we are now equipped to build the required user interface to generate the above user interface.
5) I shall provide the steps that you should follow to get the required output.
- We shall add the views first.
- TypeOfExams.aspx
- BusinessExams.ascx
- TechnicalExams.ascx
- WhoClearedThisExam.ascx
- We shall add the controller Action methods in the HomeController.cs
- public ActionResult TypeOfExams()
- public ActionResult BusinessExams()
- public ActionResult TechnicalExams()
- public ActionResult WhoClearedThisExam()
6) Now we provide a menu for Invoking the TypeOfExams.aspx page in the master page.
- Here I have removed the default About menu and added a new menu for our View.
<ul id="menu">
<li><%= Html.ActionLink("Home", "Index", "Home")%></li>
<li><%= Html.ActionLink("My Exams", "TypeOfExams", "Home")%></li>
</ul>
7) Now in the views we need to build the required layout using the plain HTML tags. To keep the example simple I have hardcoded the data in the view. But in real time the data can come from the database. The application can use the layers architecture and enterprise library to get the data from the database and bind it to view.
8) Now that we have got our Views ready we need to use Ajax and jQuery to put them all together and achieve the desired layout as per Image 4. So let's check out how we have done this.
- Check the HTML code in all aspx/ascx pages that we have added. You will notice following things
- Css classes have been used in the HTML tables to get the required look and feel for the pages.
- Css classes have been added to table, tbody, tr
- These Css classes can be using from jQuery to achieve any functional requirements.
- IDs are given to different elements in the table. These IDs will be used in jQuery and Ajax calls for injecting the sub tables into the main tables when user clicks on any of the hyperlinks.
- IDs have been added to TR and Anchor elements.
- Observe that styles have not been hardcoded. These have been applied using Css. The new css classes have been added in the style.css file in the Contents folder.
- JavaScript/jQuery code has not been written on any of the aspx/ascx pages.
- Now that all HTML pages and ASP.NET MVC code is ready, we shall use jQuery and Ajax to build the hierarchical data in the View.
- For every hyperlink we need to make an Ajax call for injecting the next level of data in the hierarchical display.
- While injecting data we need to make sure that we inject it in the correct place i.e. under the correct parent.
- Also if the data already exists under the selected parent we should not make another server side call but show the already existing data.
- To check if the data already exist under a given node we have added a css class HasChild. Presence of this class indicates that the data already exists.
- Another css class HideColumn is used to toggle the visibility of the child grid/table.
- The jQuery code has been written in a new js file. This file has been added in the scripts folder with the name Demo.js.
- jQuery Code in Demo.js file
- When the document is ready jQuery fires an event. Following function gets called automatically
- $(function() {} – In this function we have registered different event that we need to handle for our UI. The click event of the Anchor tags on following links
- Business Functions,
- Technical Functions and
- Who Cleared this Exam
links have been registered. Please note that .live based jQuery syntax has been used.
For above registration we have used following functions in .js file
- function initDemo() {
- function initExpandCollapseGroup() {
- function initExpandCollapseTechnicalGroup() {
- function initExpandCollapseDomainGroup() {
Actual logic on for onclick event is provided in following function.
- function onHeaderClick(event) {
- The onHeaderClick function calls following function.
function headerClick(source, callBackMethod) {
In the above function following actions are taken
- Identify from where the call has come.
- Identify the URL where we need to post the Ajax call to get the next level of data.
- Identify the Node under which we need to inject the response that we get from the Ajax call.
- Make an Ajax call to the ASP.NET MVC application URL (controller) and pass the response received to the callback function.
For identifying correct place holder we make use of the Css classes that we have added and the ID's that we have provided to different elements in the HTML code in the aspx/ascx pages.
- The Ajax call- jQuery provides multiple ways of making Ajax calls. For this above example we have extracted the logic of making Ajax calls into a generic function and reused it for all Ajax call. Following function contains the code for making the Ajax calls.
function processRequest(sender, url, requestInfo, callBackMethod) {
- Guard functionality in Ajax call – When the user clicks to expand a particular node we make an Ajax call. To guard the user from making next Ajax calls before the first call returns we need to implement the Guard function. This is achieved by following line in the above function for Ajax call.
if ($(sender).hasClass('inProgress')) {
After successful or failed completion of the ajax call the above guard has to be removed. This is done by following code.
$(sender).removeClass('inProgress');
- Callback function. This function is used to inject the response data received from the Ajax call under the corresponding parent. We have extracted this reusable logic in injecting the data under the selected parent into a common function as given below.
function insertHTML(sender, requestInfo, data) {
- Toggle Visibility: The feature of expand and collapse is achieved by the following function. To expand a particular node we check if the child exists, if not we make the Ajax call, get the required data and inject it under correct node. To collapse the selected node we just hide that node. So that it looks like its collapsed.
function toggleClass(sender) {
- The URL to which the Ajax call will be posted is derived using following function
function getURL(groupAnchorID) {
- Now we have completed the Controllers, Views, Css, jQuery code and Ajax related functionality we should be in a position to run the sample code in the attached solution and check it working.
In this article I have just tried to explain that it's possible to build the complex looking User interface easily without using the server side controls. The in solution we have successfully implemented grid functionality without using Viewstate, session or post-back features which are offered by ASP.NET. Here we have relied on Plain HTML, ASP.NET MVC, jQuery and Ajax. Our UI is light weight and have no Viewstate or any additional framework generated ID for the screen elements.
As we have seen, with the above approach we have complete control on the way we can build our user interface. One point I would like to make here is that the above sample by no means is a completely functional hierarchical grid. This is just a starting point for you to explore and take it forward. To make this grid control fully functional, add features like pagination, sorting, search etc. A lot more work needs to be done. Possibilities are infinite. jQuery Grid plug-in is one such control that comes out of box from jQuery itself. It offers many features similar to the traditional server side controls. There are other providers available in the market that use similar approach and are offering a fully functional grid controls which can be used with ASP.NET MVC applications.