Silverlight Line Control
This article demonstrates how to create and use a Line control in Silverlight using XAML and C#.
The Line object represents a line shape and draws a line between two defined points. The X1 and Y1 properties of the Line represent the start point and X2 and Y2 properties represent the end point of the line. The Stroke property sets the color of the line and StrokeThickness represents the width of the line. A line shape does not have an interior so Fill property has no affect on a line.
Creating a Line
The Line element in XAML creates a line shape. The code snippet in Listing 1 creates a Line by setting its start point (X1, Y1) to (50, 50) and end point (X2, Y2) to (200, 200). That means a line is drawn from point (50, 50) to (200, 200). The code also sets the color of the line to red and width 4.
<Line
X1="50" Y1="50"
X2="200" Y2="200"
Stroke="Red"
StrokeThickness="4" />
Listing 1
The output looks like Figure 1.
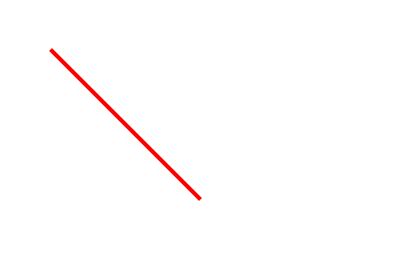
Figure 1
Creating a Line Dynamically
The CreateALine method listed in Listing 2 draws same line in Figure 1 dynamically. The code first creates a Line object, sets its X1, Y1, X2, and Y2 properties and after that sets the Stroke and StrokeThickness of the line.
/// <summary>
/// Creates a line at run-time
/// </summary>
public void CreateALine()
{
// Create a Line
Line redLine = new Line();
redLine.X1 = 50;
redLine.Y1 = 50;
redLine.X2 = 200;
redLine.Y2 = 200;
// Create a red Brush
SolidColorBrush redBrush = new SolidColorBrush();
redBrush.Color = Colors.Red;
// Set Line's width and color
redLine.StrokeThickness = 4;
redLine.Stroke = redBrush;
// Add line to the Grid.
LayoutRoot.Children.Add(redLine);
}
Listing 2
Summary
In this article, I discussed how we can create a Line control in Silverlight and C#. We also saw how to create a Line dynamically.