Introduction
This is the first "Web API with AJAX" article series presentation. This article provides a basic understanding of the Web API and concepts of AJAX in web applications. We can say that the Web API is the latest communication technology of the Microsoft platform. If we look back at Microsoft's communication technology, we will find that communication started by holding the hand of remoting techniques. Then the concept of Web Services was introduced, and SOAP messages began to send messages back and forth.
Web API with AJAX
It has a few limitations, such as only being able to be consumed by HTTP and being able to host on IIS. Then WCF took entries with valuable features. The limitation of IIS has gone, and people started to host their applications in various environments. Then new problems began. It's a programmer's nightmare to configure endpoints and consume them through a proper endpoint (If you are a good developer, you may ignore the line you have read just now). Then Microsoft introduced the concept of the Web API in MVC platforms on top of the traditional ASP.NET.
The Web API is a Swiss knife with many features and capabilities. It has simplified the concept of communication. And supports true RESTful features. The most beautiful advantage of the Web API is (almost) any client can consume it. Whether your client is built using C#, JavaScript, or another language running on various platforms and devices does not matter. We will not continue our explanation much more because this is not core to a Web API article. So, let's start our coding session by assuming you have a basic understanding of HTTP POST, GET, PUT, and DELETE verbs to talk about Web API applications.
Per the title, we will understand how to consume the Web API service using a POST method. If you are new in this environment (read Web API and RESTful service) and still want to read, the following paragraph is for you.
When discussing RESTful services, we need to consider every object a resource. For example, we want to read the employee information whose id is 5, so the information is nothing but a resource. If I want to get the picture whose name is "flower", then I think of the picture as nothing but a resource that a few HTTP verbs can consume. In a broad sense, consume implies save, retrieve, update, and delete. Now, our beloved CRUD operation can match with the HTTP verb. Here is the mapping sequence:
- POST: Create
- GET: Read
- PUT: Update
- DELETE: delete
So, the idea is that when we perform a create operation, we will use the POST verb with respect to the RESTful service, and in this article, we will use the jQuery ajax() function for the post-operation.
As per the concept of any service application, we must implement both a client and a server. Here we will implement the client using JavaScript and JQuey. So, let's start to write the code.
Implement client code to consume Web API
This is the client-side implementation to consume the Web API service. We are loading jQuery from the local server and using the ajax() function.
<%@ Page Language="C#" AutoEventWireup="true" CodeBehind="APICall.aspx.cs" Inherits="WebApplication1.APICall" %>
<!DOCTYPE html>
<html xmlns="http://www.w3.org/1999/xhtml">
<head runat="server">
<title></title>
<script src="jquery-1.7.1.js" type="text/javascript"></script>
<script>
$(document).ready(function () {
$("#Save").click(function () {
var person = new Object();
person.name = $('#name').val();
person.surname = $('#surname').val();
$.ajax({
url: 'http://localhost:3413/api/person',
type: 'POST',
dataType: 'json',
data: person,
success: function (data, textStatus, xhr) {
console.log(data);
},
error: function (xhr, textStatus, errorThrown) {
console.log('Error in Operation');
}
});
});
});
</script>
</head>
<body>
<form id="form1">
Name :- <input type="text" name="name" id="name" />
Surname:- <input type="text" name="surname" id="surname" />
<input type="button" id="Save" value="Save Data" />
</form>
</body>
</html>
Please note the formation of the data we collect from the HTML form. Since we have configured the ajax() method to communicate with the JSON data, we need to form the data in object format. So, this is the implementation of the client-side code.
Create Web API to serve RESTful service
In this section, we will create the Web API application. We are skipping the basic Web API creation part, hoping you know. Here is the implementation of Web API.
using System;
using System.Collections.Generic;
using System.Linq;
using System.Net;
using System.Net.Http;
using System.Web.Http;
namespace WebApplication1.WebAPI
{
public class person
{
public string name { get; set; }
public string surname { get; set; }
}
public class personController : ApiController
{
[HttpPost]
public string Post( person obj)
{
return obj.name + obj.surname;
}
}
}
At first, we created a model class (person) very similar to the client object we will send from the client application. The person controller contains the Posr() method specified with the HttpPost attribute. This implies that the POST verb can consume this action/method. We are halting the execution flow and checking the form data to the Web API.
In the Web API, we are getting the value we sent from the client end.
In the successful callback function, we just print this value to the console. Here is a sample output.
Use the post() function of the jQuery library
Here is another way to perform the POST operation. We can use the post() function to perform the post-operation. The post() function is nothing but a shorthand implementation of the ajax() function.
Have a look at the following code.
<%@ Page Language="C#" AutoEventWireup="true" CodeBehind="APICall.aspx.cs" Inherits="WebApplication1.APICall" %>
<head runat="server">
<script src="jquery-1.7.1.js" type="text/javascript"></script>
<script>
$(document).ready(function () {
$("#Save").click(function () {
var person = new Object();
person.name = $('#name').val();
person.surname = $('#surname').val();
$.post('http://localhost:3413/api/person', person, function (data) {
console.log(data);
});
});
});
</script>
</head>
<body>
<form id="form1">
Name :- <input type="text" name="name" id="name" />
Surname:- <input type="text" name="surname" id="surname" />
<input type="button" id="Save" value="Save Data" />
</form>
</body>
</html>
Here is the output of the post() method.
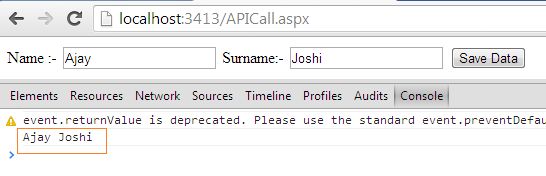
Conclusion
This is the first lap of our Web API Ajax journey. Today we have learned the POST method and its practical implementation of that. In a future article, we will learn all types of HTTP verbs and a few concepts of Web API. Happy learning.