If you have been lucky enough to get the Compact Framework or Smart devices extension beta for April 2002 you may be wondering what you can do with it. After previous articles showing some simple C# code I though why not do something with the Internet and getting stock quotes is a good idea.
However before delving into this article ask yourself why, bearing in mind that Pocket PC comes with a pretty good web browser would you write an application to access a web site directly? The simple reason is that most web sites do not consider that they may be accessed by a small device like a Pocket PC and so write web pages assuming everyone is running on a large screen. Of course a pocket PC screen is typically one quarter the size of a VGA screen so you can see the problem.
Even so if you accessed nasdaq.com using the Pocket PC browser it would do a fairly good job of trying to fit all the information on screen. Of course all this takes time and as web sites get more complicated the browser on the Pocket PC does a lot of work working out how to present information designed for a large screen on to the small Pocket PC screen.
That is why being able to write programs like this and bypass a web browser alltogether can be useful.
Here is what the application runs like on my Pocket PC emulator:
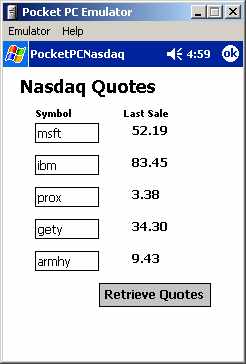
As you can see from the code I am using the WebRequest and WebResponse classes to talk to www.nasdaq.com. I read the response into a string and then search through it using the IndexOf methd of the string class. Using this simple technique allows me to search through the returned HTML code and extract the data I need. Of course if Nasdaq.com change their pages then its likely this code will break.
Once again I tested this code on the Pocket PC emulator and it worked fine if slightly slowly. Some things to note where this program could be improved.
- Program will fail if an invalid symbol is entered
- I create a WebRequest for each symbol rather than grouping them all into one request. This was purely done to make the code simpler to read.
Lastly, to show that this program is waiting for web responses I display an hourglass using Pocket PC APIs to inform the user the program is actually busy.
When I get more time later versions of this program will gather more Nasdaq information and allow you to do things like symbol lookups etc
//PocketPCNasdaq.cs
//Lets you check stock quotes over internet on a Pocket PC
//Written using Compact Framework Beta 1
//Written May 21st 2002 by John O'Donnell - [email protected]
using System;
using System.Windows.Forms;
using System.Net;
using System.IO;
using System.Text;
using System.Runtime.InteropServices;
namespace PocketPCNasdaq
{
public class Form1 : System.Windows.Forms.Form
{
private const int hourGlassCursorID = 32514;
//Pocket PC API's to show and hide waitcursor
[DllImport("coredll.dll")]
private static extern int LoadCursor(int zeroValue, int cursorID);
[DllImport("coredll.dll")]
private static extern int SetCursor(int cursorHandle);
private System.Windows.Forms.Label label1;
private System.Windows.Forms.Label label5;
private System.Windows.Forms.Label label4;
private System.Windows.Forms.Label label3;
private System.Windows.Forms.Label label2;
private System.Windows.Forms.TextBox textBox1;
private System.Windows.Forms.TextBox textBox2;
private System.Windows.Forms.TextBox textBox3;
private System.Windows.Forms.TextBox textBox4;
private System.Windows.Forms.TextBox textBox5;
private System.Windows.Forms.Button button1;
private System.Windows.Forms.Label label7;
private System.Windows.Forms.Label label8;
private System.Windows.Forms.Panel panel1;
public Form1()
{
InitializeComponent();
}
protected override void Dispose( bool disposing )
{
base.Dispose( disposing );
}
#region Windows Form Designer generated code
// Some code here
#endregion
static void Main()
{
Application.Run(new Form1());
}
private void button1_Click(object sender, System.EventArgs e)
{
this.ShowWaitCursor(true);
if (textBox1.Text!="")
label2.Text=GetQuote(textBox1.Text);
if (textBox2.Text!="")
label3.Text=GetQuote(textBox2.Text);
if (textBox3.Text!="")
label4.Text=GetQuote(textBox3.Text);
if (textBox4.Text!="")
label5.Text=GetQuote(textBox4.Text);
if (textBox5.Text!="")
label6.Text=GetQuote(textBox5.Text);
this.ShowWaitCursor(false);
}
//Returns the current value for a particular stock
public string GetQuote(string symbol)
{
string a;
string b;
string c;
string d="";
string query=http://quotes.nasdaq.com/Quote.dll?mode=stock&symbol=;
query=query+symbol+"&&quick.x=0&quick.y=0";
WebRequest wrq = WebRequest.Create(query);
// Return the response.
WebResponse wr= wrq.GetResponse();
StreamReader sr = new StreamReader(wr.GetResponseStream(),Encoding.ASCII);
a=sr.ReadToEnd().ToString();
if(a.IndexOf("data")>-1)
{
int pos1=a.IndexOf("[");
int pos2=a.IndexOf("]");
b=a.Substring(pos1+1,pos2-pos1);
pos1=b.IndexOf(";");
c=b.Substring(pos1,10);
pos1=c.IndexOf(",");
d=c.Substring(1,pos1-2);
}
//close resources between calls
wr.Close();
sr.Close();
return(d);
}
// Shows or Hides the wait cursor on the PPC
public void ShowWaitCursor(bool ShowCursor)
{
int cursorHandle = 0;
if (ShowCursor)
{
cursorHandle = LoadCursor(0, hourGlassCursorID);
}
SetCursor(cursorHandle);
}
}
}