This code is written using LINQ Preview May 2006 CTP.
To run this sample code, you must have Visual Studio 2005 Express or other Visual Studio 2005 versions.
After that, install LINQ Preview May 2006 CTP version from MSDN website.
After installation of LINQ Preview May 2006 CTP, click on New Project in the File menu of Visual Studio 2005, select LINQ Preview in the Project Types and create a LINQ Console Application from the selected templates.
Note:
If you want to write your sample code in VB.NET, Select Visual Basic in the Project types and LINQ in the Templates pane.
See Figure 1.
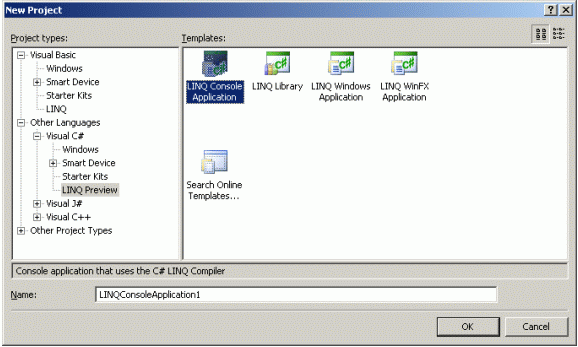
Figure 1.
As name suggests, LINQ (.NET Integrated Query Language) provides from..where..select syntax to select data from collections.
Understanding from..where..select
The from..where..select syntax in LINQ is similar to SELECT..FROM..WHERE clause of SQL. Here is a simple from..select.
from day in daysArray
select day;
In the above statement, I select day in daysArray. It returns an object of "var" type, which is a generic type and can store any type. For example, daysArray can be an array of numbers, strings, or even objects. Here is an example of daysArray.
string
[] daysArray = { "Mon", "Tue", "Wed", "Thur", "Fri", "Sat", "Sun" };
This is how return value is stored from a from..select statement.
var days = from day in daysArray select day;
Now where clause is used to filter the results. For example, the following syntax gets retuslts from daysArray where value of day is "Wed" only.
var days = from day in daysArray where day =="Wed" select day;
Filtering using where
The following code selects items from an array that have value below 20.
int[] IntArray = { 12, 5, 24, 10, 9, 8, 4, 87, 23, 7, 11, 43 };
var under20 = from u20 in IntArray where u20 < 20 select u20;
Console.WriteLine("Numbers below 20 are: ");
Console.WriteLine("=====================");
foreach (var numU20 in under20)
{
Console.WriteLine(numU20);
}
Console.WriteLine("======================");
Console.ReadLine();
The output looks like Figure 2.
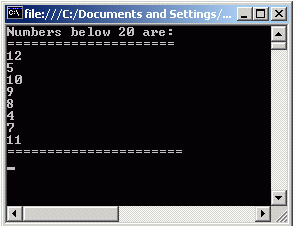
Figure 2.
The following query is used to get items between two values, greater than 8 and less than 20.
var under20 = from u20 in IntArray where u20 < 20 && u20 > 8 select u20;
The output looks like Figure 3.
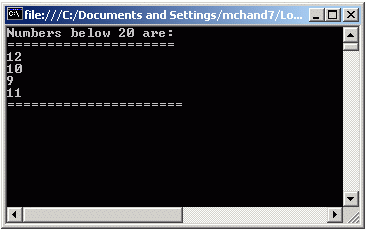
Figure 3.
The exact match items can be retrieved using == operator. For example, the following syntax selects items that have value 23.
var under20 = from u20 in IntArray where u20 == 23 select u20;
The output looks like Figure 4.
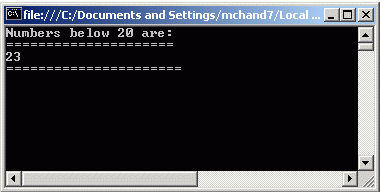
Figure 4.
Check out More Fun with LINQ Technology by Mike Gold