Overview
I have noticed that in many articles, I read, people use a longer method of clearing many textboxes, as well as handling more than one control at the same time. In this article Ill try to show you how to do that in a better way.
Description
Lets imagine a situation in which you have many textboxes in your form, and you have to clear them at the end of the operation. In several articles I saw that people do it this way:
textBox1.Text = ;
textBox2.Text = ;
textBoxn.Text = ;
A better way to do that is to do
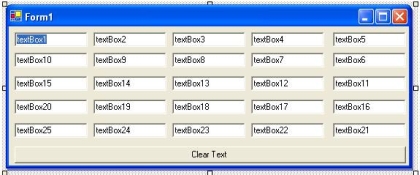
in
this example in the button event:
private void button1_Click(object sender, System.EventArgs e)
{
for (int i = 0; i < this.Controls.Count; i++)
if (this.Controls[i] is TextBox)
this.Controls[i].Text = "";
}
The second thing is the possibility of handling more than one control at the same time. For example, when the mouse enters the textbox and I want to change the BackColor property of the cell. Then the mouse leaves the textbox and I want to restore the previous situation.
Can you imagine rewriting (in this case) the same routine 21 times??? Here is a very easy way to do that.
First, lets start with the MouseEnter event on textBox1:
and write that:
private void textBox1_MouseEnter(object sender, System.EventArgs e)
{
((TextBox)sender).BackColor = Color.Red;
}
and now the same in the MouseLeave event
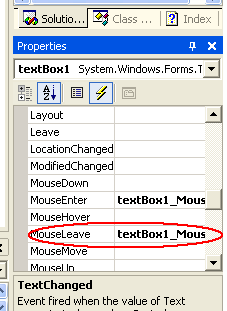
and write that:
private void textBox1_MouseLeave(object sender, System.EventArgs e)
{
((TextBox)sender).BackColor = Color.White;
}
now the only thing you have to do is to select the textBox2 (and so on) and to associate in the MouseEnter event (and in the MouseLeave event) the event generated in the textBox1. Here is the code:
this.textBox2.MouseEnter += new System.EventHandler(this.textBox1_MouseEnter);
this.textBox2.MouseLeave += new System.EventHandler(this.textBox1_MouseLeave);
this.textBox3.MouseEnter += new System.EventHandler(this.textBox1_MouseEnter);
this.textBox3.MouseLeave += new System.EventHandler(this.textBox1_MouseLeave);
And at design time from VS.NET (for MouseEnter):
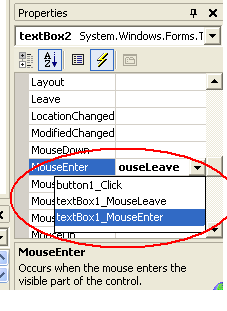
and (MouseLeave):
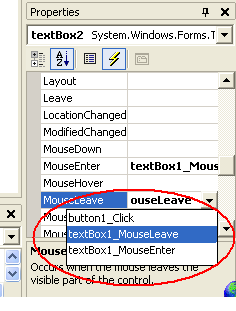
thats it
Enjoy !!!