Today there are a large number of books covering .NET and Windows Forms. While most of these books discuss the essentials of working with Windows Forms and guide you well on your way to becoming proficient in developing Windows Forms applications, very few books cover a vital and much needed topic. And this topic is: the sequence of events that are triggered for a Form. Knowing the lifecycle of a Form can help you place important bits of code in relevant events.
If you look at ASP.NET tutorials and books, you will find many references to the Web Page lifecyle but what about Windows Forms lifecycle? Sadly, there's not much concrete information about this. The aim of this article is, therefore, to delve into this topic and provide insightful knowledge about Form events.
The events in the lifecycle of a Form from the time it is launched to the time it is closed are listed below:
- Move: This event occurs when the form is moved. Although by default, when a form is instantiated and launched, the user does not move it, yet this event is triggered before the Load event occurs.
- Load: This event occurs before a form is displayed for the first time.
- VisibleChanged: This event occurs when the Visible property value changes.
- Activated: This event occurs when the form is activated in code or by the user.
- Shown: This event occurs whenever the form is first displayed.
- Paint: This event occurs when the control is redrawn.
- Deactivate: This event occurs when the form loses focus and is not the active form.
- Closing: This event occurs when the form is closing.
- Closed: This event occurs when the form is being closed.
Let us view this through an example.
1. First, launch Visual Studio IDE (2005 or 2008) and create a Windows Forms application.
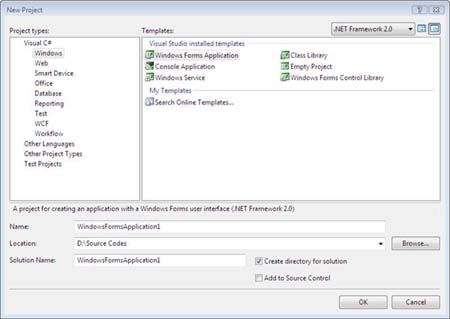
Figure 1: New Project Dialog Box
2. Give a suitable name and click OK. This will create the aplication and open it in Design view.
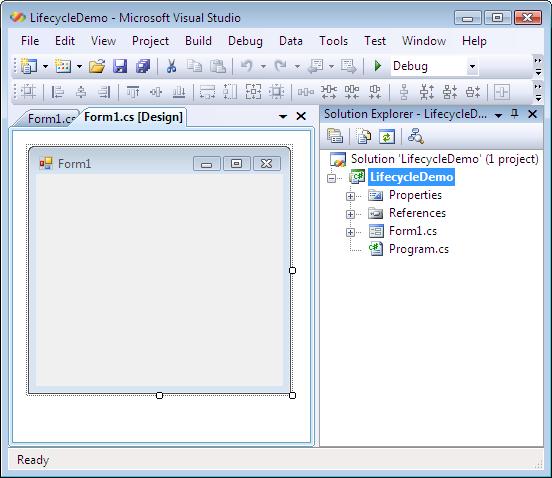
Figure 2: Application in the Design view
3. Open the Form properties window. The easiest way to do this is: select the Form in the design view and press the F4 key.
4. Click the Events tab and select the Move event.
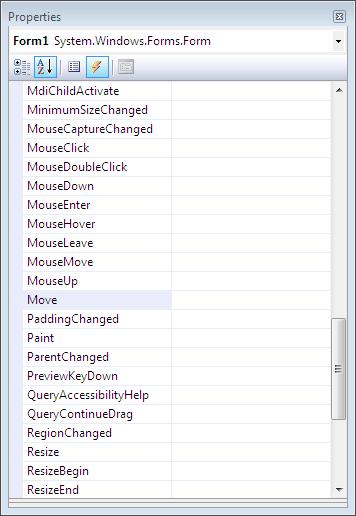
Figure 3: Form events
5. Double click on it. This will cause the event handler to be auto-generated in the Code View.
6. Switch back to the Design View and in the Form properties window, double click the Load event.
7. Likewise, repeat this procedure for all the events that were listed earlier.
8. Open the Code View of Form1.Designer.cs and add the code marked in bold.
using System.IO;
namespace LifecycleDemo
{
partial class Form1
{
StreamWriter sr = new treamWriter("D:\\formevents.txt");
/// <summary>
/// Required designer variable.
/// </summary>
private System.ComponentModel.IContainer components = null;
/// <summary>
/// Clean up any resources being used.
/// </summary>
/// <param name="disposing">true if managed resources should be disposed; otherwise, false.</param>
protected override void Dispose(bool disposing)
{
if (disposing && (components != null))
{
components.Dispose();
}
base.Dispose(disposing);
sr.Close();
}
#region Windows Form Designer generated code
#endregion
}
}
9. Open the Code View of Form 1 and add the code marked in bold.
public partial class Form1 : Form
{
public Form1()
{
InitializeComponent();
}
private void Form1_Move(object sender, EventArgs e)
{
sr.WriteLine("1 - Move event");
}
private void Form1_Load(object sender, EventArgs e)
{
sr.WriteLine("2 - Load event");
}
private void Form1_Activated(object sender, EventArgs e)
{
sr.WriteLine("3 - Activated event");
}
private void Form1_VisibleChanged(object sender, EventArgs e)
{
sr.WriteLine("4 - VisibleChanged event");
}
private void Form1_Shown(object sender, EventArgs e)
{
sr.WriteLine("5 -Shown event");
}
private void Form1_Paint(object sender, PaintEventArgs e)
{
sr.WriteLine("6 - Paint event");
}
private void Form1_FormClosed(object sender, FormClosedEventArgs e)
{
sr.WriteLine("7 - FormClosed event");
}
private void Form1_FormClosing(object sender, FormClosingEventArgs e)
{
sr.WriteLine("8 - FormClosing");
}
private void Form1_Deactivate(object sender, EventArgs e)
{
sr.WriteLine("9 - Deactivate");
}
}
10. Next, you save, build and execute the application. (File->Save is used for the save operation and Build->Build Solution is used to build the application. To execute, click Debug->Start Debugging.
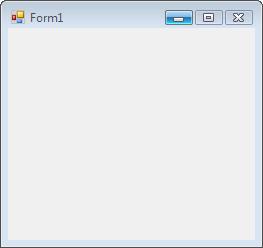
Figure 4: Form shown during execution of the application
11. Switch to some other application, such that the form is no longer in focus.
12. Switch back to the Windows Form so that it regains focus.
13. Exit the Windows Form application.
14. Open the text file, formevents.txt. You will observe the output similar to the one shown in Figure 5. (Output may vary if you perform some other actions in between causing additional events to be raised).
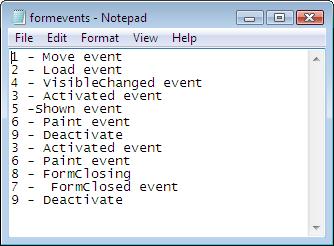
Figure 5: Text file contents showing event lifecycle
The Move, Load, VisibleChanged and Activated events are raised even before the Form is shown. Then the Shown event takes place. This is followed by the Paint event. These events are common for any application and are always standard.
When you switch the focus to some other application, the Deactivate event occurs. When the form regains focus, the Activated event is raised. Then the form is painted again because it has regained focus.
When you attempt to close the form, the FormClosing and FormClosed events are raised. Finally, after the form is closed, Deactivate is raised once more. If you had inserted a WriteLine for Dispose as well (which has not been written as of now) you would see that statement appearing after the Deactivate.
Conclusion: Thus, you learned about the lifecycle of events in a Windows Form.