This article will display how to use AutoComplete control in Windows Phone 7. AutoComplete is used for when you type something into a textbox a popup window opens with a list of words that begin with the prefix typed into the textbox.
If you are looking for AutoComple in JQuery and Ajax then refer to my previous articles:
AutoComplete using JQuery
Auto Complete in ASP.NET using Ajax
Getting Started
Creating a Windows Phone Application:
- Open Visual Studio 2010.
- Go to File => New => Project
- Select Silverlight for Windows Phone from the Installed templates and choose Windows Phone Application
- Enter the Name and choose the location.
- Click OK.
Image 1.
First of all add a reference of System.Windows.Control.Input.dll. You will find all DLLs in Silverlight for Windows Phone toolkit.
Let's add a class
Image 2.
Technologies.cs
using System;
using System.Net;
using System.Windows;
using System.Windows.Controls;
using System.Windows.Documents;
using System.Windows.Ink;
using System.Windows.Input;
using System.Windows.Media;
using System.Windows.Media.Animation;
using System.Windows.Shapes;
using System.Collections.ObjectModel;
using System.ComponentModel;
namespace MCMobile
{
public class Technologies : INotifyPropertyChanged
{
public event PropertyChangedEventHandler PropertyChanged;
private ObservableCollection<Technology> _listOftechnologies;
public ObservableCollection<Technology> ListOfTechnologies
{
get { return _listOftechnologies; }
set
{
_listOftechnologies = value;
if (PropertyChanged != null)
PropertyChanged(this, new PropertyChangedEventArgs("ListOfTechnologies"));
}
}
public Technologies()
{
ListOfTechnologies = new ObservableCollection<Technology>();
ListOfTechnologies.Add(new Technology() { Name = "C#" });
ListOfTechnologies.Add(new Technology() { Name = "VB.NET" });
ListOfTechnologies.Add(new Technology() { Name = "ASP.NET" });
ListOfTechnologies.Add(new Technology() { Name = "Java" });
ListOfTechnologies.Add(new Technology() { Name = "Win Forms" });
ListOfTechnologies.Add(new Technology() { Name = "Web Forms" });
ListOfTechnologies.Add(new Technology() { Name = "Ajax" });
ListOfTechnologies.Add(new Technology() { Name = "DirectX" });
ListOfTechnologies.Add(new Technology() { Name = "Exception Handling" });
ListOfTechnologies.Add(new Technology() { Name = "HTML" });
ListOfTechnologies.Add(new Technology() { Name = "JQuery" });
ListOfTechnologies.Add(new Technology() { Name = "Printing" });
ListOfTechnologies.Add(new Technology() { Name = "Networking" });
ListOfTechnologies.Add(new Technology() { Name = "Remoting" });
ListOfTechnologies.Add(new Technology() { Name = "Project Management" });
ListOfTechnologies.Add(new Technology() { Name = "Silverlight" });
ListOfTechnologies.Add(new Technology() { Name = "Sharepoint" });
ListOfTechnologies.Add(new Technology() { Name = "Threading" });
ListOfTechnologies.Add(new Technology() { Name = "WPF" });
ListOfTechnologies.Add(new Technology() { Name = "XNA" });
ListOfTechnologies.Add(new Technology() { Name = "Testing" });
ListOfTechnologies.Add(new Technology() { Name = "Cloud Computing" });
ListOfTechnologies.Add(new Technology() { Name = "Windows Services" });
ListOfTechnologies.Add(new Technology() { Name = "Web Services" });
}
}
public class Technology
{
public string Name { get; set; }
public override string ToString()
{
return Name;
}
}
}
Now drag and drop AutoComplete control on windows phone page. Now add these two references on page.
xmlns:toolkit="clr-namespace:Microsoft.Phone.Controls;assembly=Microsoft.Phone.Controls.Toolkit"
xmlns:MCMobile="clr-namespace:MCMobile"
<phone:PhoneApplicationPage.Resources>
<MCMobile:Technologies x:Key="Technologies"></MCMobile:Technologies>
</phone:PhoneApplicationPage.Resources>
<!--LayoutRoot is the root grid where all page content is placed-->
<Grid x:Name="LayoutRoot" Background="Transparent" DataContext="{StaticResource Technologies}">
<Grid.RowDefinitions>
<RowDefinition Height="Auto"/>
<RowDefinition Height="*"/>
</Grid.RowDefinitions>
<!--TitlePanel contains the name of the application and page title-->
<StackPanel x:Name="TitlePanel" Grid.Row="0" Margin="12,17,0,28">
<TextBlock x:Name="PageTitle" Text="AutoComplete Sample" Margin="9,-7,0,0" Style="{StaticResource PhoneTextTitle1Style}" FontSize="32"/>
</StackPanel>
<!--ContentPanel - place additional content here-->
<Grid x:Name="ContentPanel" Grid.Row="1" Margin="12,0,12,0">
<toolkit:AutoCompleteBox HorizontalAlignment="Left" Margin="12,76,0,0"
Name="autoCompleteBox1"
VerticalAlignment="Top"
Width="326"
ValueMemberBinding="{Binding Name}"
ItemsSource="{ Binding ListOfTechnologies}" >
<toolkit:AutoCompleteBox.ItemTemplate>
<DataTemplate>
<TextBlock Text="{Binding Name}"></TextBlock>
</DataTemplate>
</toolkit:AutoCompleteBox.ItemTemplate>
</toolkit:AutoCompleteBox>
<TextBlock Height="30" HorizontalAlignment="Left" Margin="24,6,0,0" Name="textBlock1" Text="Enter technology name" VerticalAlignment="Top" Width="298" />
</Grid>
</Grid>
Now build and run the application to see the result.
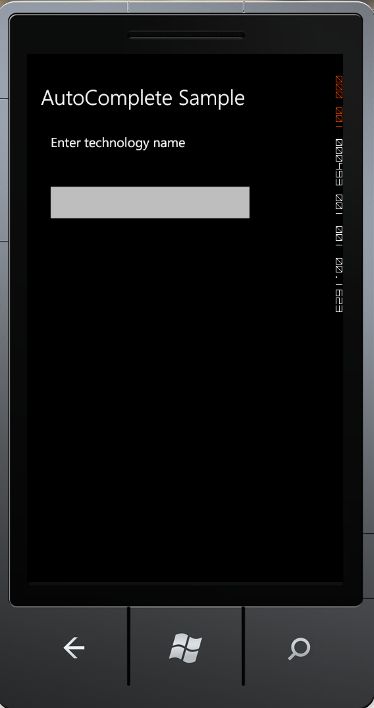
Image 3.
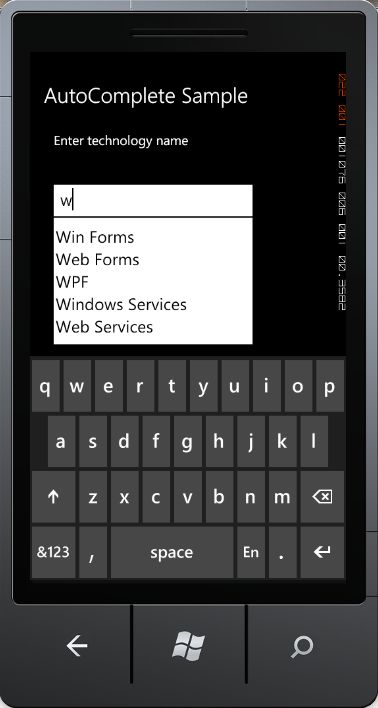
Image 4.
Image 5.
We are done here with windows phone auto complete. If you have questions or comments, drop me a line in c-sharpcorner comments section.