Recently I needed to develop code for reading RSS feeds from a blog and load into another site using Ajax. The top most feeds are already available through the feedburner url. But the requirement was to load the feed asynchronously so that the loading time of the site would not be affected by the feed loading time.
So to do that I wrote the simple JavaScript code here like:
var xhr;
function getRSS()
{
var method="GET";
var url="http://feeds.feedburner.com/lfsblogger?format=sigpro";
//call the right constructor for the browser being used
if (window.ActiveXObject){
xhr = new ActiveXObject("Microsoft.XMLHTTP");
xhr.open(method, url, true);
}
else{
xhr = new XMLHttpRequest();
}
xhr.onreadystatechange = function() {
if (xhr.readyState == 4)
{
if (xhr.status == 200 || xhr.status == 0)
{
if (xhr.responseText != null)
{
processRSS(xhr.responseXML);
}
else
{
alert("Failed to receive RSS file from the server - file not found.");
return false;
}
}
else
alert("Error code " + xhr.status + " received: " + xhr.statusText);
}
}
//send the request
xhr.send(null);
}
This was working good in IE6 but in firefox 3.5+ and IE7+ the statement xhr.responseText was returning blank. I was wondering why it's happening. Then I searched Google and found that there is a new protocol introduced for cross domain requests.
The Cross-Origin Resource Sharing (CORS) protocol is a W3C Working Draft that defines how the browser and server must communicate when accessing sources across origins. The basic idea behind CORS is to use custom HTTP headers to allow both the browser and the server to know enough about each other to determine if the request or response should succeed or fail. You can found more about it from the Firefox site: Source: https://developer.mozilla.org/en/Same_origin_policy_for_JavaScript
Then I did more research and I found the solution using JQuery to deal with it and to make a cross domain Ajax request. Thanks to James Padolsey to write the JQuery plugin. What you have to do is just add the below JQuery files in your project:
<script src="jquery-1.6.min.js" type='text/javascript'></script>
<script src="xAjaxJquery.js" type='text/javascript'></script>
I've attached both the files in the attachment. Now what you have to do is just make an Ajax request just like:
function getRSS()
{
this.txt;
$.ajax({
url: 'http://feeds.feedburner.com/lfsblogger?format=sigpro',
type: 'GET',
success: function(res) {
var headline = res.responseText;
var rssxml=parseHtml(headline);
tmpElement = rssxml.getElementsByTagName("p")[0];
if (tmpElement != null)
eval("feed=tmpElement.childNodes[0].nodeValue");
//Removing the newline characters from the response
/***** Firefox only needs this line *****/
feed=feed.replace(/\n/g,' ');
/***** IE and Opera need this line also *****/
feed=feed.replace(/\s/g,' ').replace(/ ,/g,' ');
//Custom formatting of feeds to convert it to HTML from the Feed burner's format
feed = feed.replace(/document.write\("/g,'').replace(/"\);/g,'');
feed=unescape(feed.replace(/\\x/g,'%'));
showFeeds();
}
});
}
I've to write some custom code for parsing the result as it returns the complete HTML having the response inside the body so I wrote the method parseHtml to get the exact response. Also it embedded some new line characters in the response text. So cleaning the result is some extra work you have to do.
But I wrote the complete code for you so there is no need to panic.
var xmlDoc;
function parseHtml(_html)
{
if (window.DOMParser)
{
parser=new DOMParser();
xmlDoc=parser.parseFromString(_html,"text/xml");
}
else // Internet Explorer
{
xmlDoc=new ActiveXObject("Microsoft.XMLDOM");
xmlDoc.async="false";
xmlDoc.loadXML(_html);
}
return xmlDoc;
}
function showFeeds()
{
document.write(feed);
}
You don't have to write anything in HTML. It'll remain simple and plain as shown below:
<!DOCTYPE HTML PUBLIC "-//W3C//DTD HTML 4.0 Transitional//EN" >
<html>
<head>
<script src="jquery-1.6.min.js" type='text/javascript'></script>
<script src="xAjaxJquery.js" type='text/javascript'></script>
<script src="getRSS.js" type='text/javascript'></script>
</head>
<body>
</body>
</html>
Just open the HTML file in browser and see the output:
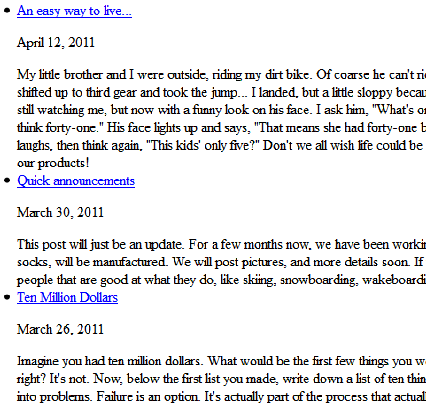
I have uploaded all the files in the attachment. Download, modify and use as you need.
Thanks.
Happy coding…