In this article we can explore the queue creation in storage account.
As a refresh, I would like to repeat that there are 3 types in Azure Storage
The Table creation was explored in the previous article. Queue creation will be explored here.
Concepts in Queue
Following are the key concepts in queue.
- FIFO implementation
- Messages are added to end of the Queue and processed from the front
- Queues provides a good way of Front end and Back end decoupling
In the real world example the user can queue a job through the web role (front end) and the job can be processed by a worker role (back end). This gives an opportunity to decouple the web role and worker role.
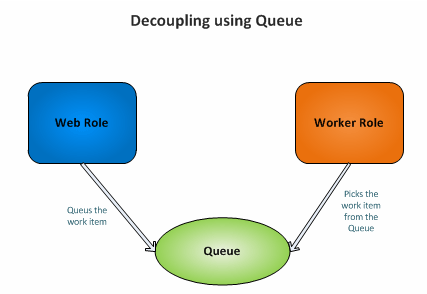
Note: Blob are stored in container, Entity in table and Message in Queue
The steps involved in creating a queue are following:
Step 1: Create new project
As always create a new azure project and add a web role into it. Now add reference to the StorageClient dll file.
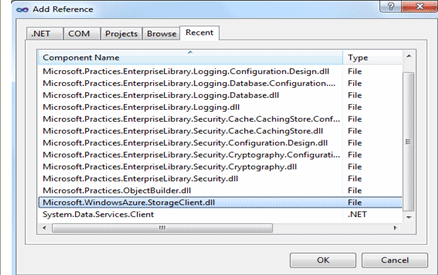
Step 2: Modify the Default.aspx
Place a new label control on the aspx page and add the following code in the page load event.
protected void Page_Load(object sender, EventArgs e)
{
StorageCredentialsAccountAndKey accountAndKey = new StorageCredentialsAccountAndKey("account", "key");
CloudStorageAccount account = new CloudStorageAccount(accountAndKey, true);
CloudQueueClient client = account.CreateCloudQueueClient();
CloudQueue queue = client.GetQueueReference("workitems");
queue.CreateIfNotExist();
CloudQueueMessage message = new CloudQueueMessage("Test Work Item");
queue.AddMessage(message);
// Populate the messages
message = queue.GetMessage();
if (message != null)
Label1.Text = "Message in Queue: " + message.AsString;
}
Place your account name and key in the credentials. Ensure that the queue name is lower case (upper case will throw exception)
The above code will create a new message in the queue and after that it will retrieve the queue message and show in the page.
Important Classes
The important classes in the queue processing are stated below:
- CloudQueueClient
- CloudQueue
- CloudQueueMessage
The CloudQueueClient class takes care of getting the queue reference from the storage account. The CloudQueue class takes care of the holding the queue object. The CloudQueueMessage holds the actual message for the queue.
Summary
In this article we have seen how to put message into Queue and later retrieve it. The code attached contains the projects discussed above.