In this example we will create a Web Service which provides spell check functionality. We will learn how to access the Word Automation object through COM Interoperability using the .Net Framework.
Step 1
Create a Visual C# Web Services project in Visual Studio.Net. You could also use any other editor and create a .asmx file for the Web service.
Step 2
Add a reference to Microsoft Word Object Library.
Step 3
Add a Web Service method with the code shown below. An explanation of the code follows the code listing.
[WebMethod]
public String SpellCheck(String strCheck)
{
Boolean bResult;
String strReturn;
Object filler = null;
Word.Application appWord = null;
try
{
appWord = new Word.Application();
bResult = appWord.CheckSpelling(strCheck, ref filler,ref filler, ref filler, ref filler, ref filler, ref filler , ref filler, ref filler, ref filler, ref filler, ref filler , ref filler);
strReturn = "Spell Check result: " + bResult;
}
catch(Exception Ex)
{
strReturn = "Exception occurred:" + Ex.Message;
}
finally
{
if (appWord != null)
{
appWord.Quit(ref filler, ref filler,ref filler);
}
}
return strReturn;
}
Code Listing : Spell Check Web Service Method
You will need to verify that the web service runs with the required permissions on the server.
Explanation
We create an instance of the Word Application and invoke the CheckSpelling method on the Word object for the string passed to the web method as input parameter. The other arguments expected by the CheckSpelling method are not required to be set by our method. In C#, the arguments are not optional and we need to pass null objects as parameters.
If an exception occurs, the return string contains some details of the explanation and otherwise we return the results of the spell check.
After the processing is over, we close the Word Application in the program. This is an important step as the Word process instances will linger on the server unless the Application is Quit.
Here are the results:
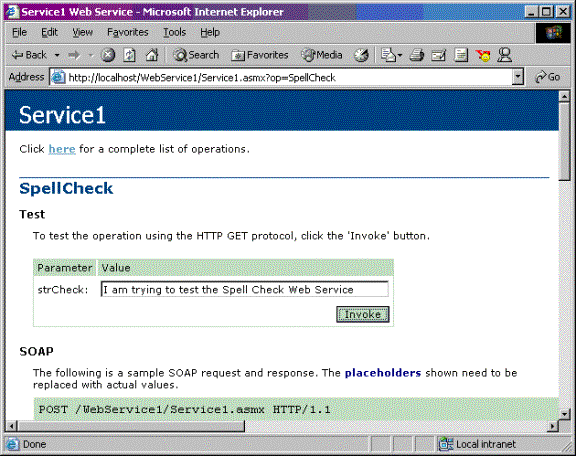
Figure 1: Testing the Spell Check Web Service
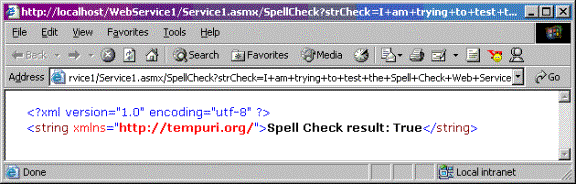
Figure 2: Results from the Spell Check Web Service
You can easily create clients to access functionality from this web service. The web method can be invoked from other Web Services, Win Forms Applications, Web Forms Applications, even Mobile Web Forms applications You could also access this web method from a Java application.
NOTE: Microsoft does not recommend Server Side Automation of Office. (Refer to http://support.microsoft.com/default.aspx?scid=KB;EN-US;Q257757) The main purpose of this example is to demonstrate the use of COM Interop from Web Services. This example is purely for educational purposes. Please be sure to check any licensing issues if you plan to use a similar strategy in commercial applications.