Validation Groups in ASP.Net 2.0
Validation Controls are used to validate user input entered in a web form.
Types of Validation Controls:
- RequiredFieldValidator Control: Ensures that the user provides a value for the specied control
- CompareValidator Control: Validate a control value against a constant value or against the value of another control.
- RangeValidator Control: Validate a control value against an upper and lower boundary value.
- RegularExpressionValidator Control: Validate a control value against a specified expression pattern.
- CustomValidator Control: Validate a control value using custom validation logic.
Validation Groups
Validation Group is a new feature introduced in ASP.Net 2.0. This enhancement allows specifying selective partial validation in a web form using validation controls. Each validation group is evaluated separately from other validation groups in the web form.
How are Validation Groups specified?
You can specify Validation Groups by setting the ValidationGroup Property of Validation Controls to a value. All the Validation Controls with the same value set for the ValidationGroup property are included in the same Validation Group.
How do you specify the Validation Group that is to be used for the validation event?
Controls that can trigger validation, such as the button control, dropdownlist control etc, have the ValidationGroup property which is used to identify the set of validation controls to evaluate, if this control activates a PostBack.
How are Validation Groups evaluated?
When a Page is posted back, the IsValid property of the Page is set based on the evaluation of the validation controls that are included in the ValidationGroup which is specified in the control causing the Postback.
Different controls can thus be setup to invoke a different set of validations for the web form.
Case Sensitive
Note that the ValidationGroup property is case-sensitive.
Sample: Using Validation Groups
In this sample, the RequiredFieldValidator Controls setup for the Login and Password text fields are included in the "VGrpAuthenticate" validation group and the RequiredFieldValidator Control setup for the NickName field is included in the "VGrpAnon" validation group.
The Login button control's ValidationGroup is set to "VGrpAuthenticate" and clicking the Login button results in evaluation of only the RequiredFieldValidator controls setup for the login and password text boxes. The RequiredFieldValidator control for the NickName field is not included in this ValidationGroup
Sample Code: Using Validation Groups
<%@ Page Language="C#" AutoEventWireup="true" CodeFile="Default.aspx.cs" Inherits="_Default" %>
<!DOCTYPE html PUBLIC "-//W3C//DTD XHTML 1.0 Transitional//EN" "http://www.w3.org/TR/xhtml1/DTD/xhtml1-transitional.dtd">
<html xmlns="http://www.w3.org/1999/xhtml" >
<head runat="server">
<title>Untitled Page</title>
</head>
<body>
<form id="form1" runat="server">
<center><H3> Validation Groups Sample</H3>
<div style="width:60%;border-bottom:blue thin solid;border-top:blue thin solid;border-left:blue thin solid;border-right:blue thin solid">
<br />
<center>
<table border="0">
<tr>
<td style="width: 100px; height: 23px">
User ID</td>
<td style="width: 215px; height: 23px">
<asp:TextBox ID="txtLogin" runat="server"></asp:TextBox>
<asp:RequiredFieldValidator ID="RequiredFieldValidator1" runat="server" ControlToValidate="txtLogin"
ErrorMessage="User ID is required" ValidationGroup="VGrpAuthenticate">*</asp:RequiredFieldValidator></td>
</tr>
<tr>
<td style="width: 100px; height: 23px">
Password</td>
<td style="width: 215px; height: 23px">
<asp:TextBox ID="txtPassword" runat="server" TextMode="Password"></asp:TextBox>
<asp:RequiredFieldValidator ID="RequiredFieldValidator2" runat="server" ControlToValidate="txtPassword"
ErrorMessage="Password cannot be blank" ValidationGroup="VGrpAuthenticate">*</asp:RequiredFieldValidator></td>
</tr>
<tr>
<td style="width: 100px; height: 21px">
NickName</td>
<td style="width: 215px; height: 21px">
<asp:TextBox ID="txtNickName" runat="server"></asp:TextBox>
<asp:RequiredFieldValidator ID="RequiredFieldValidator3" runat="server" ControlToValidate="txtNickName"
ErrorMessage="NickName is required" ValidationGroup="VGrpAnon">*</asp:RequiredFieldValidator></td>
</tr>
<tr>
<td style="height: 21px" colspan="2">
<br />
<asp:Button ID="btnLoginAuth" runat="server" Text="Login" ValidationGroup="VGrpAuthenticate" />
<asp:LinkButton ID="LinkButton1" runat="server" ValidationGroup="VGrpAnon">Login as Guest</asp:LinkButton><br />
</td>
</tr>
</table>
</center>
</div>
<asp:ValidationSummary ID="ValidationSummary1" runat="server" ShowMessageBox="True"
ShowSummary="False" ValidationGroup="VGrpAuthenticate" />
<asp:ValidationSummary ID="ValidationSummary2" runat="server" ValidationGroup="VGrpAnon" />
</center>
</form>
</body>
</html>
Image: Using Validation Groups
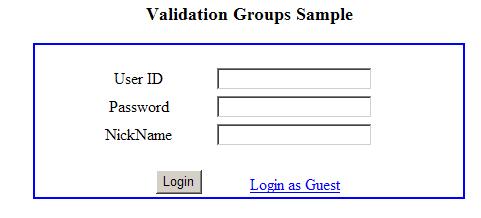
Image: Using Validation Groups: "Login" button is clicked
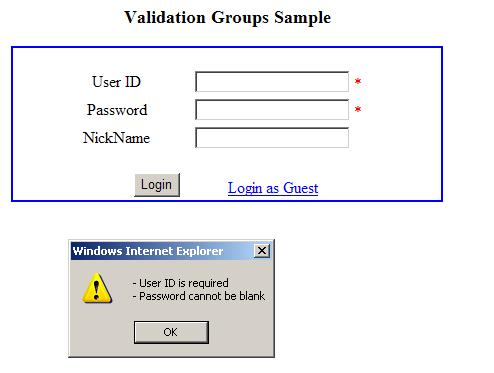
Image: Using Validation Groups: "Login as Guest" link is clicked
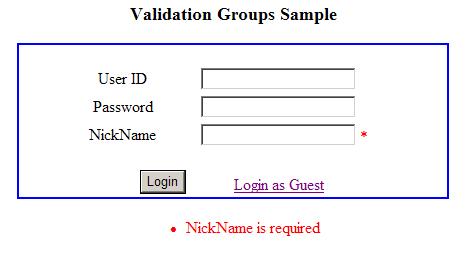
Programatic Validation
To programmatically validate based on selective groups, you can call the Page.Validate method overload which accepts a string specifying the ValidationGroup to validate.
ValidationSummary Control
The validation summary control also contains a ValidationGroup property. The validation summary control displays the summary results of the validation for a validation group if the ValidationGroup specified for the Validation Control is being evaluated and has validation errors.
Multiple ValidationSummary controls can be included in the web form, to specify different ValidationGroups and these validation summary controls can be individually configured.
Conclusion
In this article, we examined the use of the Validation Group feature. This enhancement in ASP.Net 2.0 allows for handling real life validation scenarios, which were not very easily configurable in previous versions.