Introduction
Microsoft Windows services enable you to create long-running executable applications that run in their own Windows sessions. These services (e.g. SQL server Service) can be automatically started when the computer boots, can be paused and restarted, and do not show any user interface. Whenever you need long-running functionality that does not interfere with other users who are working on the same computer, you should go for windows service
You create windows service in C# as a Microsoft Visual Studio .NET project; defining code within it that controls what commands can be sent to the service and what actions should be taken when those commands are received. Commands that can be sent to a service include starting, pausing, resuming, and stopping the service, and executing custom commands.
After you create and build the application, you can install it by InstallUtil.exe/making setup project/ServiceInstaller.exe and passing the path to the service's executable file
We will create a windows service here which will make a log file and append that log file after specified time interval, we will pass two parameters to windows service, first is the name of the log file to be created and second is time interval in seconds after which new entry will be logged into log file
Creating Windows Service
To create a windows service, in visual studio click on new project and select windows service as shown in following figure.
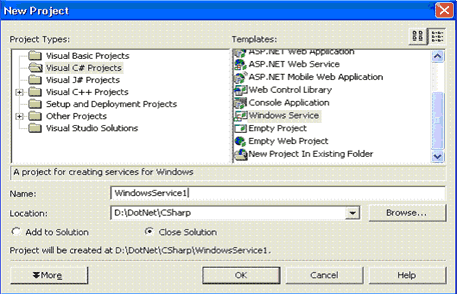
- Rename project name to AnandService
- Add one class and rename it to AnandServiceMain.cs.
- Rename service.cs class to AnandServiceHandler.cs. Note the main method of services.cs, later on we will move this method to AnandServiceMain.cs
- Add one more class and rename it to AnandServiceInstaller.cs
- Add reference to System.Configuration.Install and
- Right click on project, select properties and change assemble name to AnandService.
Now project explorer should look like this.
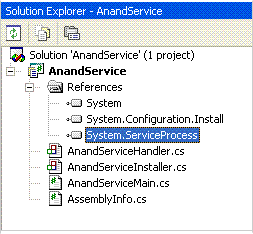
Now open AnandServiceMain.cs and paste the following code on it
using System;
using System.Collections;
using System.ComponentModel;
using System.Diagnostics;
using System.ServiceProcess;
using System.IO;
namespace AnandService
{
/// <summary>
/// Summary description for EventLogWriter.
/// </summary>
public class AnandServiceMain
{
public string _strLogPath;
public int _intSleepTime;
public AnandServiceMain()
{
//
// TODO: Add constructor logic here
//
}
public static void Main(System.String[] args)
{
AnandServiceMain anandServiceMain = new AnandServiceMain();
anandServiceMain._strLogPath = args[0];
anandServiceMain._intSleepTime = Convert.ToInt32(args[1]);
System.ServiceProcess.ServiceBase.Run(new AnandServiceHandler(anandServiceMain));
}
}
}
Now open AnandServiceHandler.cs and paste the following code on it
using System;
using System.Collections;
using System.ComponentModel;
using System.Diagnostics;
using System.ServiceProcess;
using System.IO;
namespace AnandService
{
public class AnandServiceHandler : System.ServiceProcess.ServiceBase
{
/// <summary>
/// Required designer variable.
/// </summary>
private System.ComponentModel.Container components = null;
AnandServiceMain m_AnandSvs = null;
System.Threading.Thread AnandThread = null;
int i = 0;
public AnandServiceHandler(AnandServiceMain AnandSvs)
{
m_AnandSvs = AnandSvs;
// This call is required by the Windows.Forms Component Designer.
InitializeComponent();
// TODO: Add any initialization after the InitComponent call
}
/// <summary>
/// Required method for Designer support - do not modify
/// the contents of this method with the code editor.
/// </summary>
private void InitializeComponent()
{
components = new System.ComponentModel.Container();
this.ServiceName = "AnandService";
}
/// <summary>
/// Clean up any resources being used.
/// </summary>
protected override void Dispose(bool disposing)
{
if (disposing)
{
if (components != null)
{
components.Dispose();
}
}
base.Dispose(disposing);
}
/// <summary>
/// Set things in motion so your service can do its work.
/// </summary>
protected override void OnStart(string[] args)
{
System.Threading.ThreadStart AnandThreadStart = new System.Threading.ThreadStart(WriteToLog);
AnandThread = new System.Threading.Thread(AnandThreadStart);
AnandThread.Start();
}
/// <summary>
/// Stop this service.
/// </summary>
protected override void OnStop()
{
// TODO: Add code here to perform any tear-down necessary to stop your service.
}
public void WriteToLog()
{
bool blnLog = true;
while (blnLog == true)
{
i = i + 1;
StreamWriter w = File.AppendText(m_AnandSvs._strLogPath);
Log(i.ToString(), w);
System.Threading.Thread.Sleep(m_AnandSvs._intSleepTime);
if (i > 10)
{
blnLog = false;
}
}
}
public void Log(string logMessage, TextWriter w)
{
w.WriteLine("+----------------------------------------------------------------+");
w.Write(" Log Entry " + logMessage + ": " + DateTime.Now.ToLongDateString() + " " + DateTime.Now.ToLongTimeString() + "\r\n");
w.Flush();
w.Close();
}
}
}
You may have noted that we have removed the main from services.cs (now AnandServiceHandler.cs) and make the separate file AnandServiceMain.cs, so that we can handle the parameters in better and clean way. And pass back them back to AnandServiceHandler.cs.
Now open AnandServiceInstaller.cs and paste the following code on it
using System;
using System.Collections;
using System.ComponentModel;
using System.Configuration.Install;
namespace WindowsService1
{
/// <summary>
/// Summary description for ProjectInstaller.
/// </summary>
[RunInstaller(true)]
public class ProjectInstaller : System.Configuration.Install.Installer
{
private System.ServiceProcess.ServiceProcessInstaller serviceProcessInstaller1;
private System.ServiceProcess.ServiceInstaller serviceInstaller1;
/// <summary>
/// Required designer variable.
/// </summary>
private System.ComponentModel.Container components = null;
public ProjectInstaller()
{
// This call is required by the Designer.
InitializeComponent();
// TODO: Add any initialization after the InitializeComponent call
}
/// <summary>
/// Clean up any resources being used.
/// </summary>
protected override void Dispose(bool disposing)
{
if (disposing)
{
if (components != null)
{
components.Dispose();
}
}
base.Dispose(disposing);
}
#region Component Designer generated code
/// <summary>
/// Required method for Designer support - do not modify
/// the contents of this method with the code editor.
/// </summary>
private void InitializeComponent()
{
this.serviceProcessInstaller1 = new System.ServiceProcess.ServiceProcessInstaller();
this.serviceInstaller1 = new System.ServiceProcess.ServiceInstaller();
//
// serviceProcessInstaller1
//
this.serviceProcessInstaller1.Password = null;
this.serviceProcessInstaller1.Username = null;
this.serviceProcessInstaller1.AfterInstall += new System.Configuration.Install.InstallEventHandler(this.serviceProcessInstaller1_AfterInstall);
//
// serviceInstaller1
//
this.serviceInstaller1.ServiceName = "AnandService";
this.serviceInstaller1.AfterInstall += new System.Configuration.Install.InstallEventHandler(this.serviceInstaller1_AfterInstall);
//
// ProjectInstaller
//
this.Installers.AddRange(new System.Configuration.Install.Installer[] {
this.serviceProcessInstaller1,
this.serviceInstaller1});
}
#endregion
private void serviceInstaller1_AfterInstall(object sender, System.Configuration.Install.InstallEventArgs e)
{
}
private void serviceProcessInstaller1_AfterInstall(object sender, System.Configuration.Install.InstallEventArgs e)
{
}
}
}
Now compile the project . You will find AnandService.exe in your bin/debug folder, now our exe is ready to install as windows services
Installing Windows Service
Windows Service exe cannot be run directly from the development environment or by clicking on exe. This is because the service in the project must be installed before the project can run.
You can quickly install your service application by using a command line utility called InstallUtil.exe. You can also create a setup project that contains your project's output and create a custom action with it that will run the installers associated with the project and install your service
But here, I am using the utility program ServiceInstaller.exe. ServiceInstaller.exe is a free utility and easy to use. Open on ServiceInstaller.exe you will prompting to enter text in textboxes
- Service Name - AnandSevice
- Service Label - AnandSevice
- Full Path to Executable - D:\DotNet\CSharp\WindowsService1\bin\Debug\AnandService.exe D:\DotNet\CSharp\WindowsService1\AnandService.log 30
Full Path to Executable consists of following values.
D:\DotNet\CSharp\WindowsService1\bin\Debug\AnandService.exe is the path to service exe
D:\DotNet\CSharp\WindowsService1\AnandService.log is name of the log file to be created
30 is the time interval in seconds
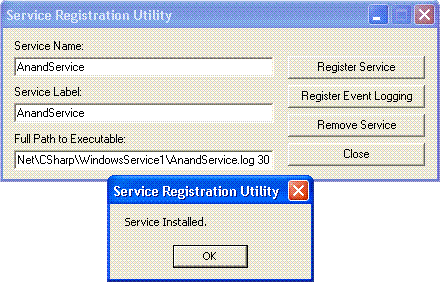
Now click on Register service and you will get the confirmation message. Close ServiceInstaller.exe. Now a service has been installed. Now open on the services from computer management console and you can see the newly created services there. Right click on service and click on Start menu.
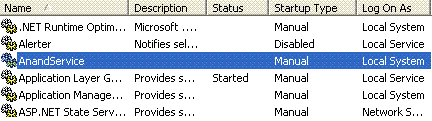
Now go to the folder, which you have passed the parameter to create log file (D:\DotNet\CSharp\WindowsService1\AnandService.log). You will see the log file there. Open log file, you can see the entries like following figure.
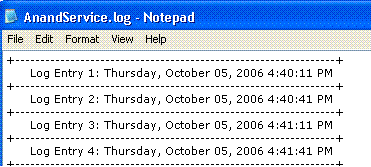
Conclusion
Windows service application is designed to be long running which don't required user interface. The .NET framework has a great support for windows services, all the code that is required for building, initializing, running, logging and performance is built into the classes on .NET framework. You can explore System.ServiceProcess and System.Diagnostics further.