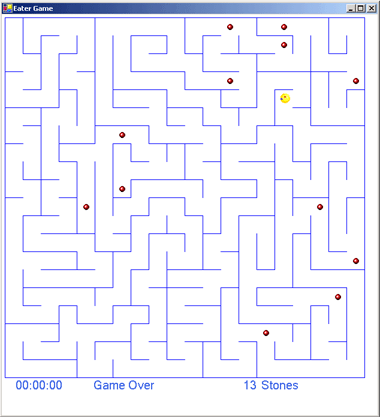
Figure 1 - Eater Game II
This program combines the designs of the Eater Game and the Maze Generation Program to create a more challenging game, Eater Game II. In this game, you move a pacman-like character through a maze and eat as many stones as you can before time runs out. You have a minute and a half to try to eat stones before the pac-man simply freezes-up and accepts his quota of stones. The design for the game adds the Maze to the Form as a 1-to-1 aggregate as shown in the UML class diagram below:
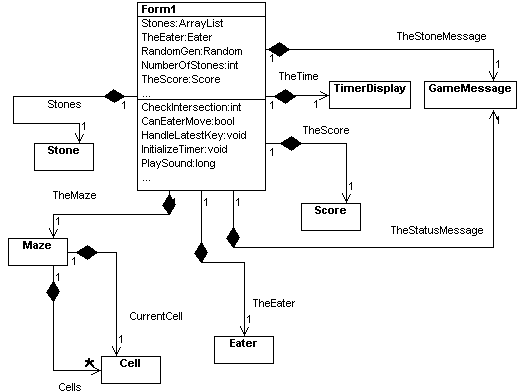
Figure 2 - Eater Game II Reverse Engineered using WithClass 2000 UML Tool for C#
The tricky part of altering this game was not drawing the maze, because this functionality is already built into the maze class. The hard challenge of bringing the maze into the picture was to keep the Pac-Man from traveling through the walls like a poltergeist every time you pressed an arrow key. The sequence diagram below shows the added functionality to test for maze walls when moving the pac-man creature.
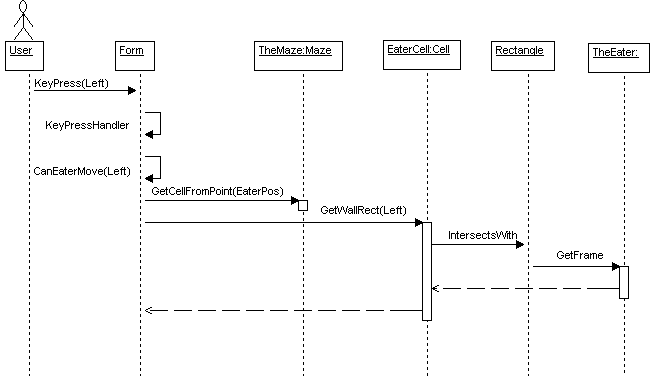
Figure 3 - Sequence of Checking for Wall Obstacle using WithClass 2000 UML Tool for C#
The sequence above shows that after the left arrow key is pressed, the program gets the cell where the eater is located in the maze. It then uses the intersection of the left wall rectangle of the cell and the bounds of the eater to determine if the eater can move towards the wall. If the eater does not intersect the wall, it can continue its motion to the left. The code for seeing if the eater can move is shown below:
private bool CanEaterMove(Side aSide)
{
int theSide = (int)aSide; // convert the side enumeration into an integer
// Determine the Cell in the Maze that is at the same position as the eater
Cell EaterCell = TheMaze.GetCellFromPoint(TheEater.Position.X + 10, TheEater.Position.Y + 10);
// If their is a wall on that side check to see if the eater intersects the wall
if (EaterCell.Walls[theSide] == 1)
{
// Ask the cell to return a rectangle representing the wall and see if the eater already intersects with it.
// If it does intersect, the eater is blocked. Return false
if (EaterCell.GetWallRect((int)aSide).IntersectsWith(TheEater.GetFrame()))
{
return false; // blocked
}
}
return true; // not blocked
}
Improvements
The game could be enhanced with a top score display as well as a reset button so the user can quickly try his luck at the game again. Also the test for maze wall barriers needs fine tuning because the eater seems to overrun the wall slightly when it is traveling along a perpendicular wall. Also it would be cool to add a "shift key" capture so the eater can be accelerated through straight-aways. Anyway, have fun with the game. I can't promise hours of enjoyment, but I can promise you'll enjoy it more than the previous game.