Introduction:
This article describes a quick and simple approach to creating gradient panel custom control. In this example, the standard Windows Forms panel container control is extended to provide a persistent gradient background; this differs from using an approach where a gradient background is created dynamically against the graphics context of the panel. Even though the background is persistent, it is dynamically updated whenever it is resized. Figure 1 illustrates the control in use:
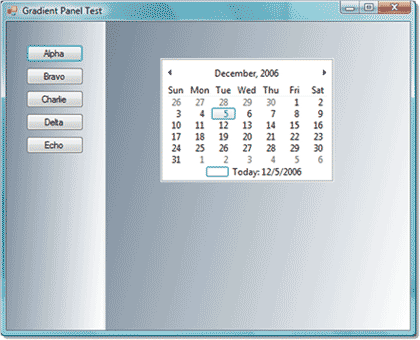
Figure 1. Gradient Panel Control in Use
Getting Started:
In order to get started, start up the Visual Studio 2005 IDE and open the included project. The solution consists of a Win Forms project with a single form and a single custom control included. The form is used as a test bed for the custom control and the custom control is an extension of the standard windows forms panel control. Figure 2 shows the solution explorer for this project:
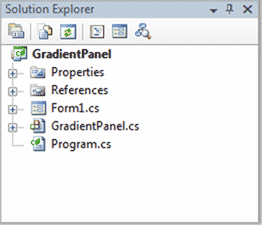
Figure 2. Solution Explorer
The Code: Main Form
The main form of the application does not contain any meaningful code; the form itself contains two instances of the gradient panel control and the gradient panel control is configured through the property editor for the purposes of the control test.
As the main form is trivial; I will not describe any further in this document.
The Code: Gradient Panel Control
The Gradient Panel control is a custom control built to extend the standard Windows Forms Panel control; the only additions made to the standard control are those required to render the gradient background at run time.
If you open the code up and examine the imports, you will note following imports prior to the namespace and class declaration.
using System;
using System.Collections.Generic;
using System.ComponentModel;
using System.Data;
using System.Drawing;
using System.Drawing.Drawing2D;
using System.Text;
using System.Windows.Forms;
namespace GradientPanel
{
public partial class GradientPanel : System.Windows.Forms.Panel
{
After the class declaration; the code creates two member variables used to hold the beginning and ending colors of the gradient. After the declaration of these two variables, the class constructor calls the Paint Gradient method which in turn paints the gradient on the panel:
// member variables
System.Drawing.Color mStartColor;
System.Drawing.Color mEndColor;
public GradientPanel()
{
InitializeComponent();
PaintGradient();
}
protected override void OnPaint(PaintEventArgs pe)
{
// TODO: Add custom paint code here
// Calling the base class OnPaint
base.OnPaint(pe);
}
The Paint Gradient method called in the class constructor is described next:
private void PaintGradient()
{
System.Drawing.Drawing2D.LinearGradientBrush gradBrush;
gradBrush = new System.Drawing.Drawing2D.LinearGradientBrush(new
Point(0, 0), new Point(this.Width, this.Height), PageStartColor,
PageEndColor);
Bitmap bmp = new Bitmap(this.Width, this.Height);
Graphics g = Graphics.FromImage(bmp);
g.FillRectangle(gradBrush, new Rectangle(0, 0, this.Width, this.Height));
this.BackgroundImage = bmp;
this.BackgroundImageLayout = ImageLayout.Stretch;
}
The Paint Gradient method is used to generate and size the linear gradient brush; this method creates a bitmap and applies the gradient to the bitmap. The background image property is then set to point at the bitmap which results in the display gradient behind the panel. Using this approach, the controls placed in the container do not interfere with the display of the gradient.
At design time, the control user may set these properties in the property grid, or the values may be set in the code.
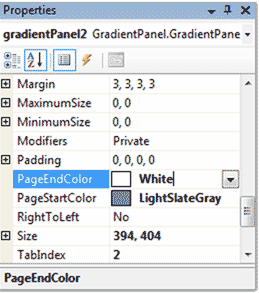
Figure 3. Properties for Start and End Colors
Summary
This article described an approach to making a persistent gradient background on a panel control based custom control. The control provided in the example could be dropped into other projects and used as is. The purpose of the control was to provide a tool for making Gradient Panel without drawing the gradient directly on the graphics context of the panel page.