I decided to expand ASP.NET sections of the site. In a series of articles, I will add articles showing most of the Web Controls and how to work with them. This series I am starting with the ListBox control.
In this article, I will discuss some commonly used ListBox Web control properties and methods and how to use List Box Web Control in Web forms.
The Rows and SelectionMode Properties
The Rows property of a ListBox control represents number of rows in a control.
The SelectionMode property represents if a list box allows multiple selection or not. The value of SelectionMode is ListSelectionMode enumeration type. The ListSelectionEnumeration has two members as shown in the following table:
The ListSelectionMode enumeration
Member |
Description |
Multiple |
Multiple item selection mode. |
Single |
Single item selection mode. |
Use the following code to select multiple items in a ListBox.
ListBox1.SelectionMode =ListSelectionMode.Multiple;
The Items Property
The Rows property of a ListBox control represents number of rows in a control.
The Items property of ListBox represents a collection of items of the control. The Items property is of type ListItemCollection class, which defines members to add, delete, insert, copy and count items to the collection. Some of the ListItemCollection class members are defined in the following table:
Member |
Description |
Capacity |
Represents maximum number of items of ListItemCollection. |
Count |
Total number of items in a collection. |
Add |
Adds an item to the collection. Takes an argument of string or ListItem type. |
Clear |
Removes all items from the collection. |
Remove |
Removes the specified item. |
InsertAt |
Adds an item at the specified location. |
RemoveAt |
Removes item from the specified location. |
Insert |
Inserts an item between two items. |
Adding Items to a ListBox
You can use ListBox.Items.Add method to add items to a ListBox control. This method takes
ListBox1.Items.Add(TextBox1.Text.ToString());
Removing Items from a ListBox
You can use ListBox.Items.Remove method to remove items from a ListBox control. The following line removes the selected item from the list.
ListBox1.Items.Remove(this.ListBox1.SelectedItem);
Sample
The following sample shows you how to add and remove items to a list control.
Create a ASP.NET Web application in VS.NET and add following controls to the form.
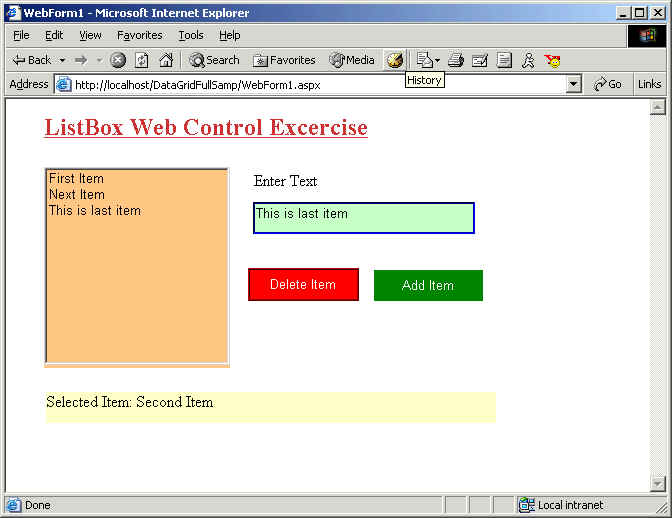
On the Add Item button click, write the following code:
private void Button1_Click(object sender, System.EventArgs e)
{
if (TextBox1.Text.ToString() == "")
{
this.Label1.Text = "Enter text in TextBox";
return;
}
this.ListBox1.Items.Add(TextBox1.Text.ToString());
}
On Delete Item button click, write the following code:
private void Button2_Click(object sender, System.EventArgs e)
{
if( this.ListBox1.SelectedIndex < 0 )
{
Label1.Text = "Select an item in ListBox";
return;
}
this.ListBox1.Items.Remove(this.ListBox1.SelectedItem);|
}