Adding a Button Click Event Handler
The Click attribute of a RepeatButton element adds the click
event handler and it keeps firing the event for the given Interval and delay
values. The code in Listing 4 adds the click event handler for a Button.
<Button x:Name="DrawCircleButton"
Height="40" Width="120"
Canvas.Left="10" Canvas.Top="10"
Content="Draw
Circle"
VerticalAlignment="Top"
HorizontalAlignment="Left">
Click="DrawCircleButton_Click"
</Button>
Listing 4
The code for the click event handler looks like following.
private void GrowButton_Click(object
sender, RoutedEventArgs e)
{
}
OK now let's write a useful application.
We are going to build an application with two buttons – Grow
and Shrink and a rectangle. The application looks like Figure 2.
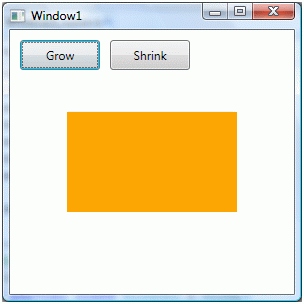
Figure 2
When you click and keep pressing the Grow button, the width
of rectangle will keep growing and when you click on the Shrink button, the
width of rectangle will keep shrinking.
The final XAML code is listed in Listing 5.
<Window x:Class="RepeatButtonSample.Window1"
xmlns="http://schemas.microsoft.com/winfx/2006/xaml/presentation"
xmlns:x="http://schemas.microsoft.com/winfx/2006/xaml"
Title="Window1" Height="300" Width="300">
<Grid Name="LayoutRoot">
<RepeatButton Margin="10,10,0,0"
VerticalAlignment="Top"
HorizontalAlignment="Left"
Name="GrowButton"
Width="80" Height="30"
Delay="500" Interval="100"
Click="GrowButton_Click">
Grow
</RepeatButton>
<RepeatButton Margin="100,10,0,0"
VerticalAlignment="Top"
HorizontalAlignment="Left"
Name="ShrinkButton"
Width="80" Height="30"
Delay="500" Interval="100"
Click="ShrinkButton_Click">
Shrink
</RepeatButton>
<Rectangle Name="Rect"
Height="100" Width="100"
Fill="Orange"/>
</Grid>
</Window>
Listing 5
Listing 6 is the click event handlers for the buttons that
change the width of rectangle.
private void GrowButton_Click(object
sender, RoutedEventArgs e)
{
Rect.Width += 10;
}
private void ShrinkButton_Click(object
sender, RoutedEventArgs e)
{
Rect.Width -= 10;
}