In this article I introduce you to Observable Collections in Silverlight.
Creating tables:
create table Person
(
id int identity primary key,
FirstName varchar(50),
LastName varchar(50)
)
insert into Person values ('Jill','Jack');
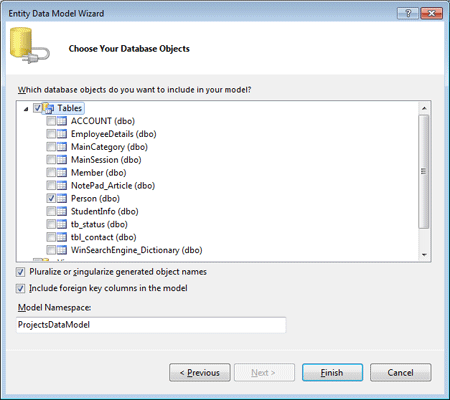
The Data Model was created as shown below:
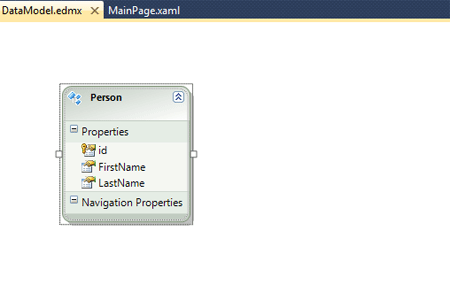
Created a class Person as shown below:
public class Person : INotifyPropertyChanged
{
public event PropertyChangedEventHandler PropertyChanged;
public void NotifyPropertyChanged(string propertyName)
{
if (PropertyChanged != null)
{
PropertyChanged(this, new PropertyChangedEventArgs(propertyName));
}
}
private string _firstname;
public string FirstName
{
get { return _firstname; }
set
{
_firstname = value;
NotifyPropertyChanged("FirstName");
}
}
}
Note that the class implements the interface InotifyPropertyChanged. The Interface looks like below:
namespace System.ComponentModel
{
// Summary:
// Notifies clients that a property value has changed.
public interface INotifyPropertyChanged
{
// Summary:
// Occurs when a property value changes.
event PropertyChangedEventHandler PropertyChanged;
}
}
Creating Silverlight Enabled WCF Service.
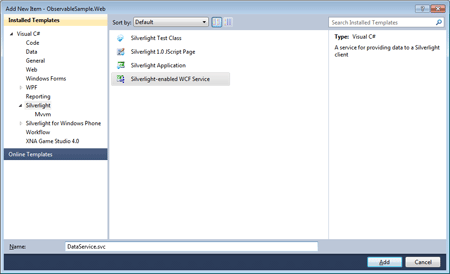
Added the method GetPersons:
[OperationContract]
public IEnumerable<Persons> GetPersons()
{
ProjectsDataEntities context = new ProjectsDataEntities();
var resultset = from person in context.People
select new Persons
{
Firstname = person.FirstName
};
return resultset;
}
Created a POCO class:
public class Persons
{
public string Firstname { get; set; }
}
Run the service:
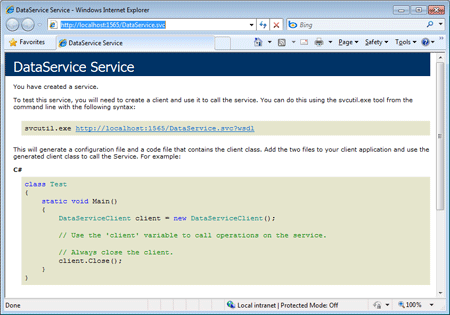
Add the Service Reference.
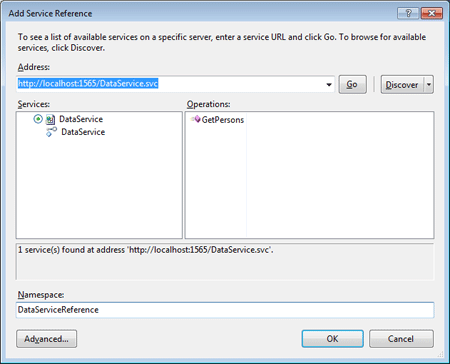
Now let's go to the Mainpage.xaml.cs.
Adding the reference as shown below:
using ObservableSample.DataServiceReference;
public partial class MainPage : UserControl
{
ObservableCollection<Persons> collection;
public MainPage()
{
InitializeComponent();
collection = new ObservableCollection<Persons>();
DataServiceClient client = new DataServiceClient();
client.GetPersonsCompleted += new EventHandler<GetPersonsCompletedEventArgs>(client_GetPersonsCompleted);
client.GetPersonsAsync();
}
void client_GetPersonsCompleted(object sender, GetPersonsCompletedEventArgs e)
{
collection = e.Result;
Dispatcher.BeginInvoke(delegate()
{
collection[0].Firstname = "Gill";
});
dataGrid1.ItemsSource = collection;
}
}
In the client_GetPersonsCompleted, I pass the resultset from the WCF Service to the Collection. Modify the first element of the collection and then assign the collection to the ItemSource of the DataGrid.
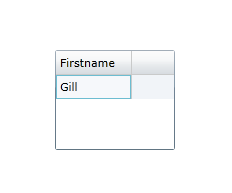
In this example we have seen how to use an Observable Collection though I have not shown you the real advantage of Observable Collection. This was for the very beginners. I will in my next article explain how an Observable collection helps us in two-way binding i.e. Database to Silverlight and Silverlight to Database.