Introduction
This article is intended to show how to invoke server-side methods (expecting input parameters) stored in the SQL Server 2005 Management System using the ObjectDataSource components. I'm going to illustrate how to pass values for these input parameters, that is, these values can be hard-coded or come from several sources such as query strings, session variables, properties of controls and others. In order to illustrate this data binding techniques, we're going to use the table Production.Product (representing business entity Product) in the database AdventureWorks shipped with SQL Server 2005.
Developing the solution
The first step is to create a Web application by opening Visual Studio.NET 2005 and select File | New | Web Site for the front-end project. Let's create a Class Library project for the data access layer components. This separation of objects (concerning their responsibilities) follows the best practices of enterprise applications development.
Let's add a strongly typed DataSet item to the Class Library project (see Figure 1).
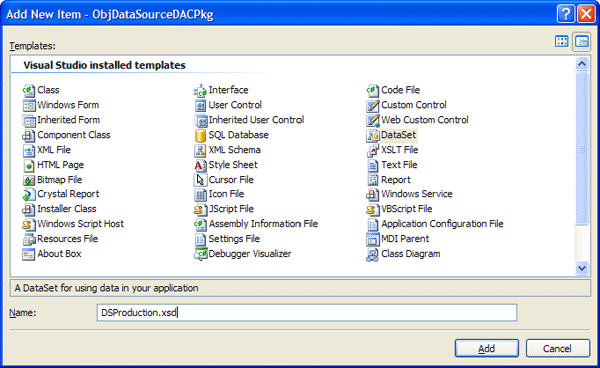
Figure 1
Let's define a TableAdapter item representing the logic to access the Production.Product table in the database AdventureWorks by using a SQL statement (see Figure 2).
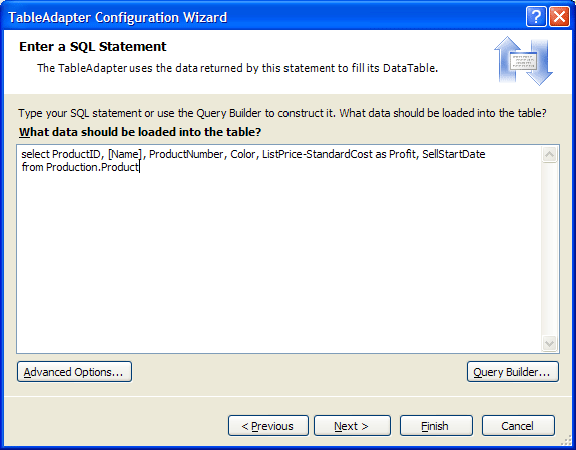
Figure 2
Now let's add a query to select a product using the product identifier criteria which is specified using input parameters. This query is shown in Figure 3 and Figure 4.
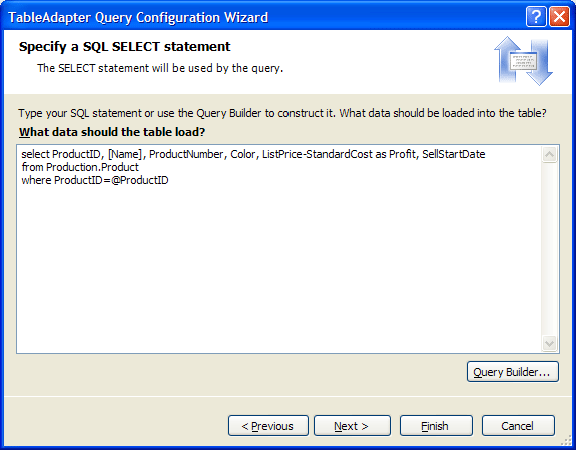
Figure 3
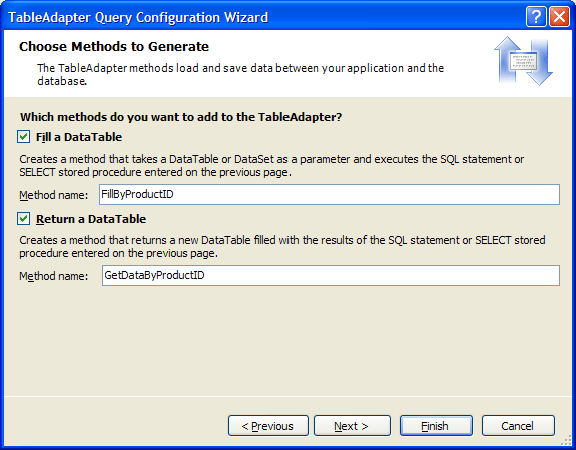
Figure 4
Now let's go to the Web front-end project and add a reference to the data access project (the Class Library project created before) and then add Web form to the project.
Now let's drag and drop an ObjectDataSource item from the Toolbox onto the Web form and click on the smart tag and select the Configure Data Source option in order to launch the configuration wizard.
In the first page (Choose a Business Object) you must select the ProductTableAdapter (see Figure 5).
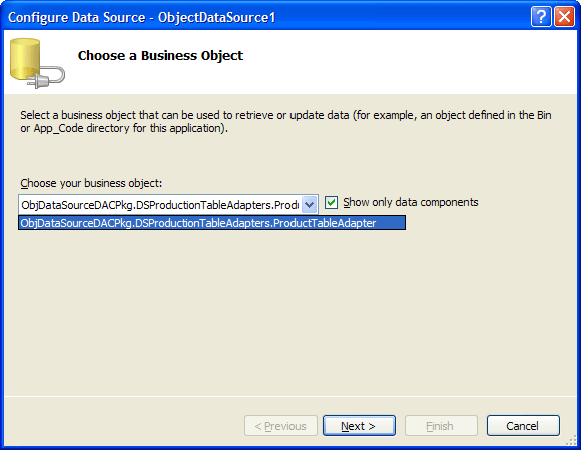
Figure 5
Click on the Next button. Because we want to display information about a particular product, in the Define Data Methods page, we select the GetDataByProductID method (see Figure 6).
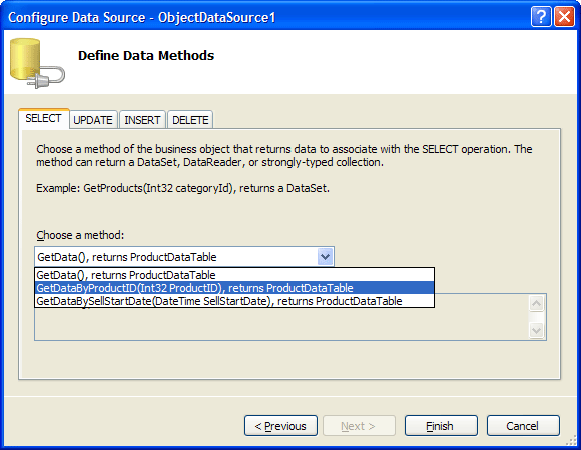
Figure 6
Click on the Finish button and you can see there's one more page for the wizard where we're asked to define the value to be used for the parameter. The Parameters list on the left shows all the parameters for the indicated method. In the Parameter Source and Default Value fields on the right, we can specify the value for the selected parameter. The Parameter Source drop-down list enumerates several possible sources for the parameter. If you want to specify a hard-coded value, you have to enter the value in the DefaultValue field (see Figure 7).
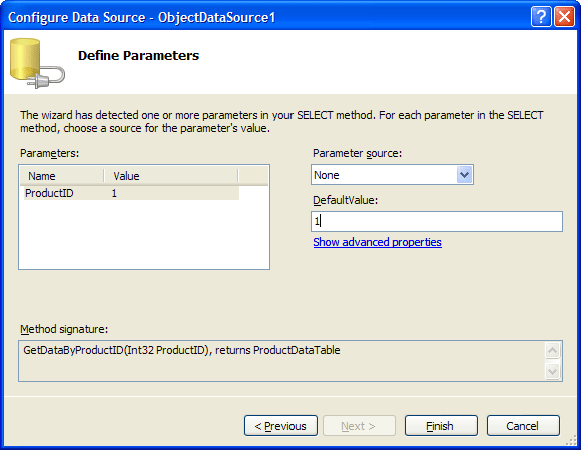
Figure 7
Now let's add a GridView Web control onto the Web form and link this control to the object data source (see Figure 8).
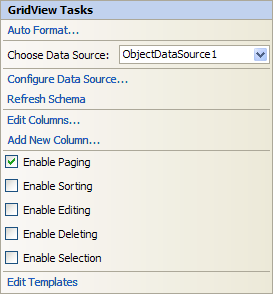
Figure 8
Now let's bind the parameter value to a property value of a Web control. Let's add a Label, a TextBox and a Button control. Set the TextBox control's ID property to ProductID. And then, re-configure the ObjectDataSource object by selecting Configure Data Source from the smart tag. In the Define Parameters page, select Control option from the Parameter Source drop-down list. Select ProductID option from the ControlID drop-down list, and set to 1 for the DefaultValue field (see Figure 9).
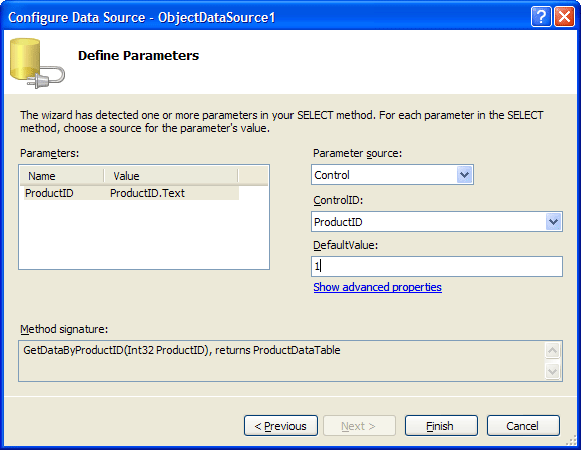
Figure 9
Now the last step is to illustrate how you can programmatically set values for the parameters in ObjectDataSource items. This scenario is very common when the parameter value comes from a source not already accounted for by one in object data source configuration.
Now let's re-configure the ObjectDataSource object again by selecting Configure Data Source from the smart tag. In the Define Parameters page, select None option from the Parameter Source drop-down list and leave the value 1 for the DefaultValue field (see Figure 10).
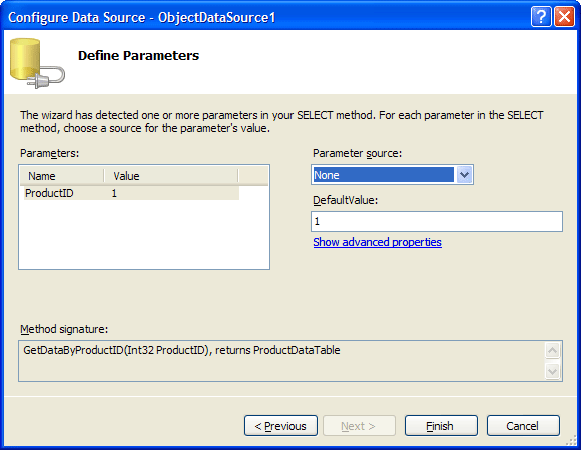
Figure 10
In order to set this value programmatically, we need to create an event handler for the ObjectDataSource item's Selecting event; by going to the Property windows, and double-clicking the Selecting event on the Events section (see Figure 11).
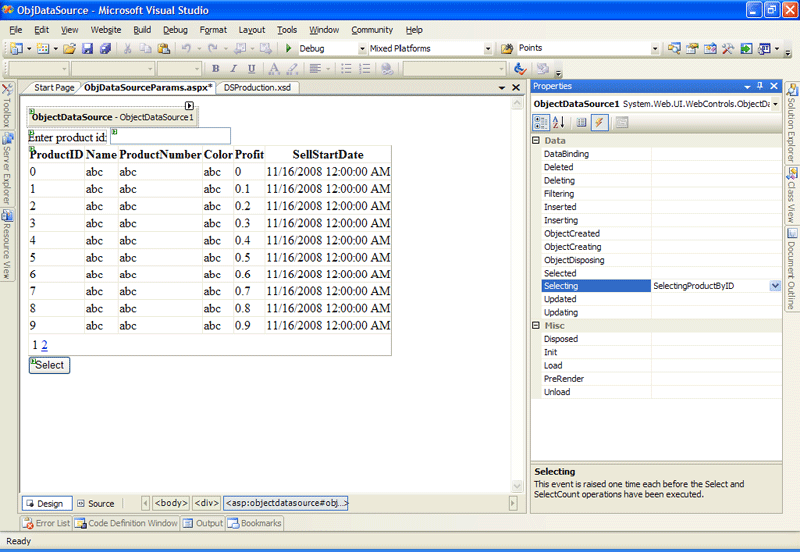
Figure 11
In this event handler, we can read and write to the parameters value using the InputParameters[parameterName] indexer of the ObjectDataSourceSelectingEventArgs event parameter (see Listing 1).
protected void SelectingProductByID(object sender, ObjectDataSourceSelectingEventArgs e) { e.InputParameters["ProductID"] = 2; }
|
Listing 1
Conclusion
In this article, I've discussed the main techniques to work with parameter values in ObjectDataSource items in ASP.NET 2.0.