This article has been excerpted from book "The Complete Visual C# Programmer's Guide from the Authors of C# Corner".
The Type property of Array objects provides information about array type declarations. Array objects with the same array type share the same Type object, so the array is said to be homogenous. But you can store wrapper classes as objects with aggregation or composition, which can store or link references to other objects. Listing 20.9 shows conversion of an Int32 array to a double array by copying and then modifying elements with changed values.
Listing 20.9: Array Copy and Conversion
// copying and converting array elements
using System;
class Test
{
public static void TestForEach(ref int[] myArray)
{
foreach (int x in myArray)
{
Console.WriteLine(x);
}
}
public static void TestForEach(ref double[] myArray)
{
foreach (double x in myArray)
{
Console.WriteLine(x);
}
}
public static void Main()
{
// an int array and an Object array
int[] myIntArray = new int[5] { 5, 4, 3, 2, 1 };
TestForEach(ref myIntArray);
Console.WriteLine("myIntArray: Type is {0}", myIntArray.GetType());
double[] myDblArray = new double[5];
Array.Copy(myIntArray, myDblArray, myIntArray.Length);
for (int i = 0; i < myDblArray.Length; i++)
myDblArray[i] += myDblArray[i] / 19;
TestForEach(ref myDblArray);
Console.ReadLine();
}
}
Output of above listing:
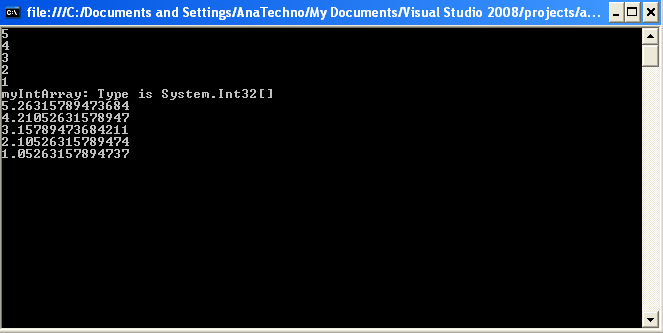
Conclusion
Hope this article would have helped you in understanding the Array Conversions in C#. See other articles on the website on .NET and C#.
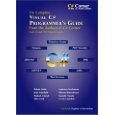 |
The Complete Visual C# Programmer's Guide covers most of the major components that make up C# and the .net environment. The book is geared toward the intermediate programmer, but contains enough material to satisfy the advanced developer. |