Delegates
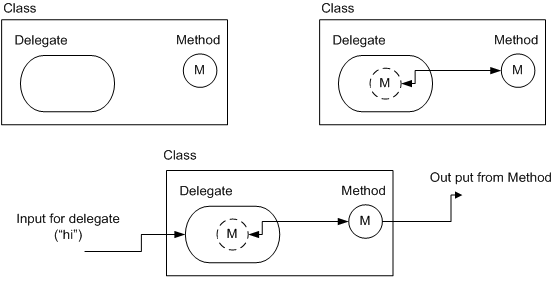
DEFINITION
Here for this example we have defined a class which contains definition for a delegate and a public static method.
i.e. delegate void myDel ( string text);
public void myMethod(string text) { ... }
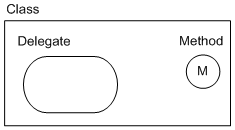
DECLARATION
Declare a delegate method which matches the return type and definition of provided delegate.To declare a delegate, attach a method with void return type and which can take string as input.
i.e. Mydel del = new MyDel(myMethod);
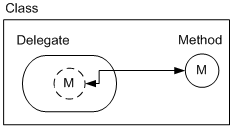
INVOCATION
To Invoke, declared delegate, you will need to call it just by passing required input parameter.
i.e. del ("Some Text")
Once you invoke the delegate,
- Delegate will pass the parameter to attached function
- Method will process the input and provide the output
If there are more than one method references in delegate than they will be called in First come first serve order
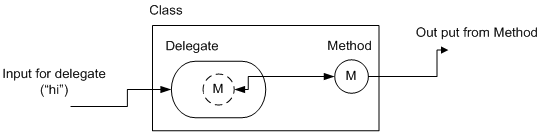
Sample Code
using System;
using System.Collections.Generic;
using System.Linq;
using System.Text;
using System.Threading;
namespace ConsoleApplication
{
class Program
{
// define a delegate for the long operation
delegate void myDelegate(string text);
static void Main(string[] args)
{
myDelegate myDelegate = new myDelegate(myMethod);
myDelegate("Hi");
}
public static void myMethod(string data)
{
Console.Write(data);
}
}
}
Where and When to Use Delegates - Real world example
- Best example of delegate is "Progress bar". One of the great functionality delegates provide is to make asynchronous calls using beinginvoke and endinvoke methods.
- Another good example is IO processing. For example if you have a heavy IO process that takes some time to finish the execution than you can trigger delegate to do this processing in the back ground meanwhile you can perform other tasks.
Articles worth Reading on Delegate
- http://blog.monstuff.com/archives/000040.html
- http://en.csharp-online.net/CSharp_Delegates_and_Events%E2%80%94Conclusion
- http://www.vikramlakhotia.com/HomePage.aspx
How are Events Tied to Delegate?
In visual studio when you double click on button from UI, it generates "Button_Click" event in code behind.
- Visual studio uses delegate mechanism to hook up your button and button_click event.
- Somewhere behind the screen there will be a hidden declaration like
Delegate void MyDel(object O , EventArgs e);
Event MyDel ClickEventHandler;
Button.Click += new ClickEventHander(...);
- This is classic delegate declaration and invocation.
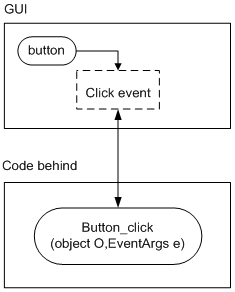
Is there a difference between Events and Delegate
As you can see internally events are defined by delegates however this question has been asked many times in interview and often developers get confused. Even though there is no theoretical difference between the two, you should note these points.
- Events can be included in Interfaces
- You can only invoke event from its own class unlike delegates
- Delegates has ability to be called for asynchronous operations using their internal mechanism i.e. beginInvoke and endInvoke