To improve the performance of application CACHING is the good way. By taking advantage of caching we can dramatically improve the performance of web applications.
Opening a database connection and retrieving the data from the database is a very slow operation. The best way to improve the performance of our data access code is not to access the database at all.
By taking the advantage of caching, we can cache our database records in memory. Retrieving data from a database is very slow. Retrieving data from cache on the other hand is lighting fast.
Here in this article I am going to show different caching mechanism supported by ASP.NET.
OverView of Caching
The ASP.NET 2.0 Frameworks supports the following types of caching
Partial Page Caching
Data Caching
Page Output Caching caches an entire page.
We can enable Page Output caching by adding
<%@ OutputCache Duration="15" VaryByParam="none" %>
directive to a page. I am showing this is this example
<%@ Page Language="C#" AutoEventWireup="true" CodeFile="Default.aspx.cs" Inherits="_Default" %>
<%@ OutputCache Duration="15" VaryByParam="none" %>
<!DOCTYPE html PUBLIC "-//W3C//DTD XHTML 1.0 Transitional//EN" "http://www.w3.org/TR/xhtml1/DTD/xhtml1-transitional.dtd">
<html xmlns="http://www.w3.org/1999/xhtml">
<head runat="server">
<title>CACHING: Page Output Caching</title>
</head>
<body>
<form id="form1" runat="server">
<div>
<asp:Label ID="lblTime" runat="server"></asp:Label>
</div>
</form>
</body>
</html>
The aspx.cs code of this is
using System;
using System.Data;
using System.Configuration;
using System.Web;
using System.Web.Security;
using System.Web.UI;
using System.Web.UI.WebControls;
using System.Web.UI.WebControls.WebParts;
using System.Web.UI.HtmlControls;
public partial class _Default : System.Web.UI.Page
{
protected void Page_Load(object sender, EventArgs e)
{
lblTime.Text = DateTime.Now.ToString();
}
}
Here on load event I am showing the current time in a label. If I refresh the then we can see time is not updated. After 15 second time is updating because in Output cache directive I set Duration ="15".
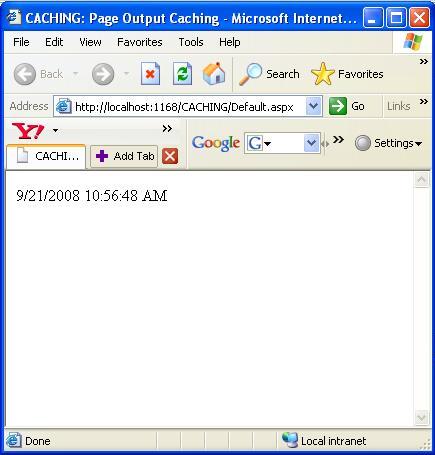
Figure 1.
If I refresh the page before 15 second then we can see that time is not updated
Figure 2.
After some time when I refresh the page then time updated
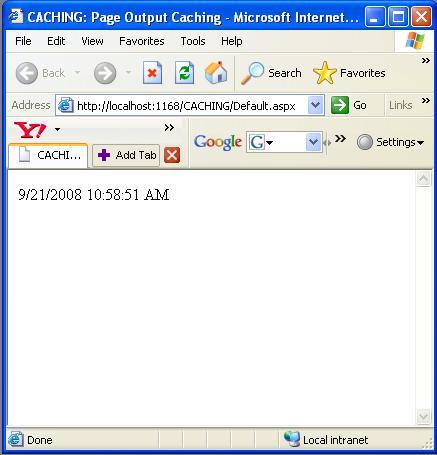
Figure 3.
VARYING the Output Cache By Parameter
Suppose we have two page on one page we are showing ID of records and on click on that ID we are fetching the respective records on other page. For this we use query string. If we cache the detail page then every one will see fist selected Employee details.
We can get around this problem by using VaryByParam attribute. The VaryByParam attribute causes a new instance of a page to be cached when a different parameter is passed to the page.
The aspx code for this is(the page where I am showing FirstName of employee I am showing, For this I used Northwind DataBase)
<%@ Page Language="C#" AutoEventWireup="true" CodeFile="OutPutCacheByPrameter.aspx.cs"
Inherits="OutPutCacheByPrameter_OutPutCacheByPrameter" %>
<!DOCTYPE html PUBLIC "-//W3C//DTD XHTML 1.0 Transitional//EN" "http://www.w3.org/TR/xhtml1/DTD/xhtml1-transitional.dtd">
<html xmlns="http://www.w3.org/1999/xhtml">
<head runat="server">
<title>Caching; Varying the Output Cache By Parameter</title>
</head>
<body>
<form id="form1" runat="server">
<div>
<asp:GridView ID="grdEmployee" runat="server" AutoGenerateColumns="false" ShowFooter="false"
ShowHeader="false">
<Columns>
<asp:HyperLinkField DataTextField="FirstName" DataNavigateUrlFields="EmployeeID"
DataNavigateUrlFormatString="EmployeeDetails.aspx?EmployeeId={0}" />
</Columns>
</asp:GridView>
</div>
</form>
</body>
</html>
The aspx.cs code
using System;
using System.Data;
using System.Configuration;
using System.Collections;
using System.Web;
using System.Web.Security;
using System.Web.UI;
using System.Web.UI.WebControls;
using System.Web.UI.WebControls.WebParts;
using System.Web.UI.HtmlControls;
using System.Data.SqlClient;
public partial class OutPutCacheByPrameter_OutPutCacheByPrameter : System.Web.UI.Page
{
SqlConnection con;
SqlCommand cmd = new SqlCommand();
DataSet ds = new DataSet();
SqlDataAdapter da;
protected void Page_Load(object sender, EventArgs e)
{
BindData();
}
public void BindData()
{
string Appcon = ConfigurationManager.AppSettings["ConnectionStr"].ToString();
con = new SqlConnection(Appcon);
cmd.CommandText = "Select * from Employees";
da = new SqlDataAdapter(cmd);
cmd.Connection = con;
con.Open();
cmd.ExecuteNonQuery();
da.Fill(ds);
if (ds.Tables[0].Rows.Count > 0)
{
grdEmployee.DataSource = ds;
grdEmployee.DataBind();
}
}
}
The Detail Page.aspx Code
<%@ Page Language="C#" AutoEventWireup="true" CodeFile="EmployeeDetails.aspx.cs"
Inherits="OutPutCacheByPrameter_EmployeeDetails" %>
<%@ OutputCache Duration="3600" VaryByParam="none" %>
<!DOCTYPE html PUBLIC "-//W3C//DTD XHTML 1.0 Transitional//EN" "http://www.w3.org/TR/xhtml1/DTD/xhtml1-transitional.dtd">
<html xmlns="http://www.w3.org/1999/xhtml">
<head runat="server">
<title>CACHING: Output Cache By Parameter: Employee Details</title>
</head>
<body>
<form id="form1" runat="server">
<div>
<asp:Label ID="lblTime" runat="server"></asp:Label><br />
<br />
<asp:DetailsView ID="dtlEmployee" runat="server">
</asp:DetailsView>
</div>
</form>
</body>
</html>
The aspx.cs code
using System;
using System.Data;
using System.Configuration;
using System.Collections;
using System.Web;
using System.Web.Security;
using System.Web.UI;
using System.Web.UI.WebControls;
using System.Web.UI.WebControls.WebParts;
using System.Web.UI.HtmlControls;
using System.Data.SqlClient;
public partial class OutPutCacheByPrameter_EmployeeDetails : System.Web.UI.Page
{
SqlConnection con;
SqlCommand cmd = new SqlCommand();
DataSet ds = new DataSet();
SqlDataAdapter da;
protected void Page_Load(object sender, EventArgs e)
{
lblTime.Text = System.DateTime.Now.ToString();
BindData();
}
public void BindData()
{
string Appcon = ConfigurationManager.AppSettings["ConnectionStr"].ToString();
con = new SqlConnection(Appcon);
cmd.CommandText = "Select * from Employees where EmployeeID=" + Request.Params[0].ToString();
da = new SqlDataAdapter(cmd);
cmd.Connection = con;
con.Open();
cmd.ExecuteNonQuery();
da.Fill(ds);
if (ds.Tables[0].Rows.Count > 0)
{
dtlEmployee.DataSource = ds;
dtlEmployee.DataBind();
}
}
}
When I run then application then the page.
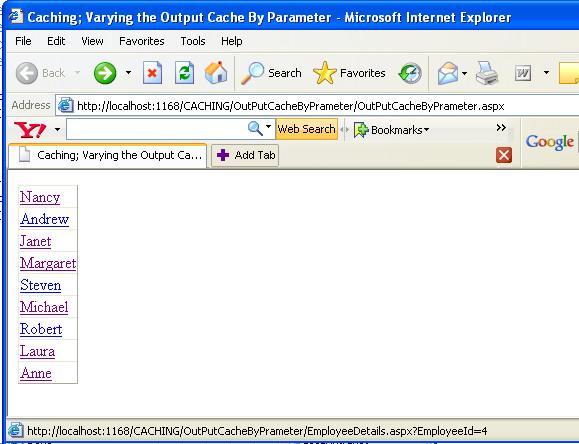
Figure 4.
When I click on any Employee Name the it shows Employee Detail Like as
Figure 5.
Here on this page I set a directive
<%@ OutputCache Duration="3600" VaryByParam="EmployeeID" %>
And I also use a label before the Employee Details we can see When I run the application first time then there is current time shows and if I go back on page where I m showing all ID of employee and click on same ID then it goes to detail page but here we can see time does not change, it’s mean our page is coming from cache not from database.
VARYING the Output Cache By Browser
There is a good way to create different cached versions of a page that depend on the type of browser being used to request the page is to use the VaryByCustom attribute. This attribute accepts the special value browser.
<%@ Page Language="C#" AutoEventWireup="true" CodeFile="CachedByBrowser.aspx.cs" Inherits="OutputCacheByBrowser_CachedByBrowser" %>
<%@ OutputCache Duration="3600" VaryByParam="none" VaryByControl="browser" %>
<!DOCTYPE html PUBLIC "-//W3C//DTD XHTML 1.0 Transitional//EN" "http://www.w3.org/TR/xhtml1/DTD/xhtml1-transitional.dtd">
<html xmlns="http://www.w3.org/1999/xhtml" >
<head runat="server">
<title>Vary By Browser</title>
</head>
<body>
<form id="form1" runat="server">
<div>
<%=DateTime.Now.ToString("T") %>
<hr />
<%=Request.UserAgent %>
</div>
</form>
</body>
</html>
When I run the application
Figure 6.
If here I close the application and run the application after sometime the we can see that time doesn't change.
In the next I will show more feature of Caching.
Enjoy Caching !!!!!