Introduction
The RbmBinaryImage control will help you display images directly from your database. You could bind the Image field directly to the ImageContent property. Also, you could specify whether you want the display to be as a thumbnail and provide the thumbnail size. 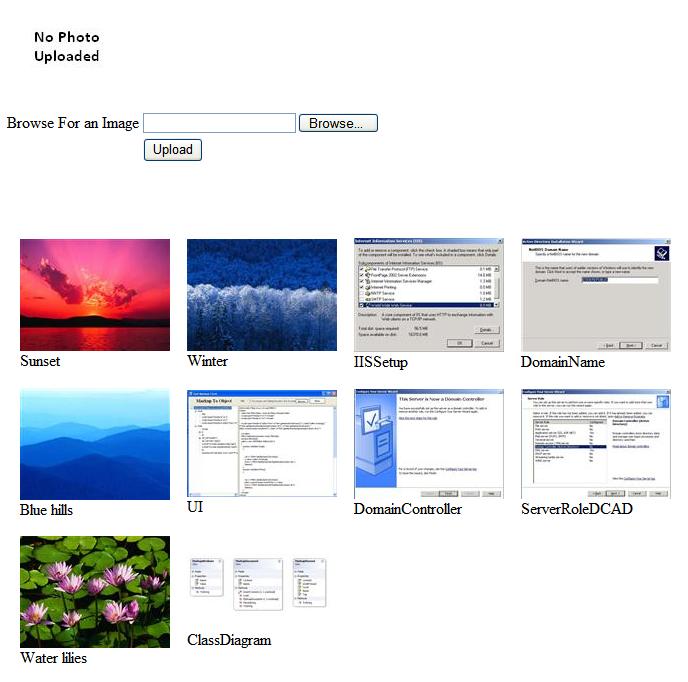
Using the RbmBinaryImage Control
First, you need to add reference RbmControls.dll, then place the code below in the system.web section in your web.config.
<httpHandlers>
<add verb="GET" path="__RbmImageHandler.rbm"
type="RbmControls.RbmImageHandler" />
</httpHandlers>
Then, in the page you want to use the control, register it by using:
<%@ Register Assembly="RbmControls" Namespace="RbmControls" TagPrefix="Rbm" %>
You could bind the control directly using the ImageContent property. Also, you can specify whether to display the image as a thumbnail, and specify an image to display when ImageContent is empty. An alternative is to do that by code:
RbmBinaryImage1.ImageContent = FileUpload1.FileBytes;
Using the Code
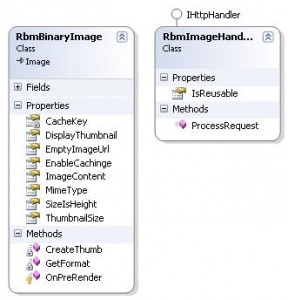
The RbmBinaryImage class inherits from System.Web.UI.WebControls.Image and add the functionality of storing and rendering a binary image. It also adds the ability to generate a thumbnail based on a specified size and also caches your image.
The Imagecontent property retrieves and stores the image bytes in the ViewState. Also, if the DisplayThumbnail property is set to true, then it retrieves the thumbnail of the image:
public byte[] ImageContent
{
get
{
byte[] imageBytes = ViewState["ImageContent"] as byte[];
if (!DisplayThumbnail)
return (imageBytes == null) ? null : imageBytes;
else if (imageBytes != null)
{
byte[] bytes = CreateThumb();
return bytes;
}
else
return null;
}
set
{
ViewState["ImageContent"] = value;
}
}
The OnPreRender method is used to set the ImageUrl based on the property settings of the Image control:
protected override void OnPreRender(EventArgs e)
{
base.OnPreRender(e);
if (DesignMode)
return;
if (ImageContent != null)
{
if (string.IsNullOrEmpty(CacheKey))
CacheKey = "Rbm" + System.DateTime.Now.Ticks;
ImageUrl = String.Format("~/__RbmImageHandler.rbm?MimeType={0}&EnableCaching={1}&ImageContent={2}",
MimeType, EnableCachinge ? "1" : "0", CacheKey);
if (this.Context.Cache[CacheKey] == null)
this.Context.Cache[CacheKey] = ImageContent;
}
else if (ImageUrl == "" || ImageUrl == null)
{
ImageUrl = EmptyImageUrl;
}
}
The RbmImageHandler class implements the IHttpHandler interface and overrides the ProcessRequest method to display the image:
public void ProcessRequest(HttpContext context)
{
#region Caching Properties
string cacheKey = context.Request["ImageContent"];
if (String.IsNullOrEmpty(cacheKey))
return;
bool enableCaching = false;
if (!String.IsNullOrEmpty(context.Request["EnableCaching"]))
Boolean.TryParse(context.Request["EnableCaching"], out enableCaching);
#endregion
#region Image Properties
string mimeType = context.Request["MimeType"];
if (string.IsNullOrEmpty(mimeType))
mimeType = "image/jpeg";
byte[] imageData = context.Cache[cacheKey] as byte[];
#endregion
if (!enableCaching)
context.Cache.Remove(cacheKey);
context.Response.ContentType = mimeType;
context.Response.OutputStream.Write(imageData, 0, imageData.Length);
}
Share your thoughts
If you liked the control or have any comments on it, or features you want to add to it, kindly share your thoughts here.
You could download the source from the top of the article.