Introduction
This article describes a simple approach to downcasting in C#; downcasting merely refers to the process of casting an object of a base class type to a derived class type. Upcasting is legal in C# as the process there is to convert an object of a derived class type into an object of its base class type. In spite of the general illegality of downcasting you may find that when working with generics it is sometimes handy to do it anyway.
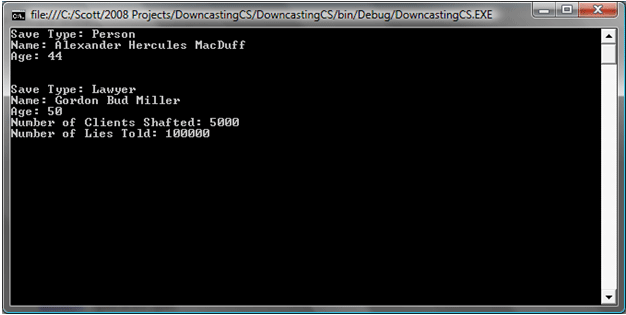
Figure 1: Test Application in Use
The Solution
The example solution provided is in the form of a console mode application; the entire solution is contained within the program.cs file.
The Code
The Program.cs file contains two classes used to provide an example of a base class and a derived class. They are the person class (the base) and the lawyer class (derived from person). The person contains some basic information about a person whilst the lawyer class contains a couple of a additional properties:
public class Person
{
public string FirstName { get; set; }
public string MiddleName { get; set; }
public string LastName { get; set; }
public int Age { get; set; }
}
public class Lawyer : Person
{
public int NumberOfPeopleShafted { get; set; }
public int NumberOfLiesTold { get; set; }
}
What now makes this all interesting is if we wanted to use a single method to save either a person or a lawyer (I'm going out on a limb here in indicating that lawyers inherit from persons). To illustrate the point, I built a static class entitled, "PeopleHandler". The sole method contained in this class can accept either a person or one of person's derived class which is in this case only lawyers. Now, if we pass the following method a object of the person type, everything is fine and all properties are visible in the code. However, if we pass in object of the lawyer type (the derived class), the object is treated as a person rather than a lawyer and the other lawyer properties are invisible to the code even though the lawyer object passed to the "SavePerson" method contains both properties. Since downcasting is not permitted, it is necessary to cast the lawyer as a lawyer rather than as a person; unfortunately, that does not work.
If you tried this: "(Lawyer)pers", the code would show an error indicating that one cannot cast T as a lawyer. Well, in this case, we know full well that "pers" is really a lawyer and not a person so it would be really helpful if we could use it as a lawyer rather than as a person.
In the following bit of code (highlighted) you can see a solution to the dilemma and it is pretty easy, just convert the lawyer to an object and then cast is over to lawyer; that works. After doing that, you will find that you can access the additional properties of the lawyer without difficulty. To avoid any problems with that, I get the type of object passed into the method and use a switch statement to limit my attempts to read lawyer properties to lawyer objects only.
The code that I used is provided in the following:
public static class PeopleHandler
{
public static void SavePerson<T>(T pers) where T : Person
{
Type tPers = pers.GetType();
string[] arr = tPers.ToString().Split('.');
string personType = arr[arr.Length - 1];
int mAge = pers.Age;
string mFirstName = pers.FirstName;
string mMiddleName = pers.MiddleName;
string mLastName = pers.LastName;
switch (personType)
{
case "Person":
Console.WriteLine("Save Type: " + personType);
Console.WriteLine("Name: " + mFirstName + " " +
mMiddleName + " " + mLastName);
Console.WriteLine("Age: " + mAge.ToString());
break;
case "Lawyer":
Console.WriteLine("Save Type: " + personType);
Console.WriteLine("Name: " + mFirstName + " " +
mMiddleName + " " + mLastName);
Console.WriteLine("Age: " + mAge.ToString());
Console.WriteLine("Number of Clients Shafted: " +
((Lawyer)(Object)pers).NumberOfPeopleShafted);
Console.WriteLine("Number of Lies Told: " +
((Lawyer)(Object)pers).NumberOfLiesTold);
break;
default:
break;
}
}
}
The Program Class
The program class itself is used to test the People Handler's "SavePerson" method by passing it an object of type person and an object of type lawyer. The results of running the executable will display information about both the person and lawyer objects passed to the method.
public class Program
{
static void Main(string[] args)
{
Person MacDuff = new Person();
MacDuff.Age = 44;
MacDuff.FirstName = "Alexander";
MacDuff.MiddleName = "Hercules";
MacDuff.LastName = "MacDuff";
PeopleHandler.SavePerson(MacDuff);
Console.WriteLine(Environment.NewLine);
Lawyer creep = new Lawyer();
creep.FirstName = "Gordon";
creep.MiddleName = "Bud";
creep.LastName = "Miller";
creep.Age = 50;
creep.NumberOfLiesTold = 100000;
creep.NumberOfPeopleShafted = 5000;
PeopleHandler.SavePerson(creep);
Console.Read();
}
}
Summary
This example provided an approach to downcasting; the article provides an example showing how downcasting might be useful when saving base and derived class objects using a common save method.