Overview
Assembly should be the reply to the issues that Microsoft defined as DLL Hell. Perhaps it wont be the end of all the issues caused by DLL but, its a very big step ahead, in fact assembly has the capacity to describe itself, so in this way you shouldnt have a problem when you change the version of one or more of your DLL. In this article, Ill try to explain how to build an assembly and use it.
Description
In this article, Ill show you how to build an assembly step by step and create a client application to access it. The first step is to start Visual Studio and choose Class Library:
then you have to add in the reference all the libraries you need (in this case I added System.Windows.Forms) and then call it in the code:
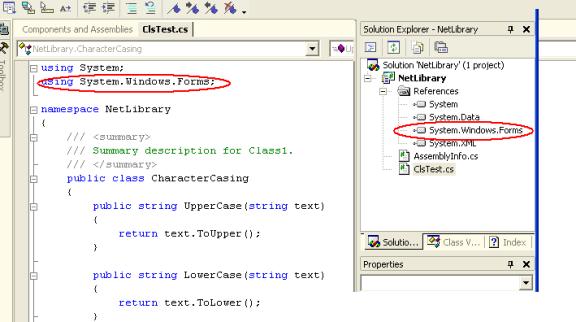
now lets write the code. The example is extremely easy and useless !! but well give us the possibility to understand in a quicker way.
namespace
NetLibrary
{
/// <summary>
/// Summary description for Class1.
/// </summary>
public class CharacterCasing
{
public string UpperCase(string text)
{
return text.ToUpper();
}
public string LowerCase(string text)
{
return text.ToLower();
}
}
}
as you can see, the first thing is to name our own namespace (in this case NetLibrary) and the you can add all the classes you need to achieve your goal. I just added one class and two methods. Its even useless to describe the code because is very simple.
Now lets use our assembly in our new project. So open Visual Studio and choose a windows application, and then add in the reference the assembly we had built:
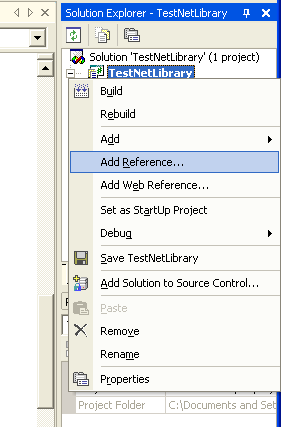
and choose browse and look for your dll:
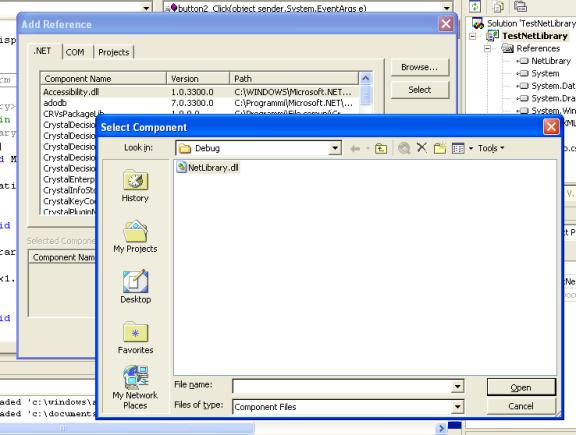
now our assembly has been added in the reference ready to be used:
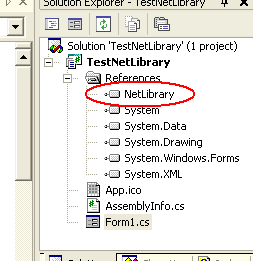
lets see how to use it:
private
void button1_Click(object sender, System.EventArgs e)
{
NetLibrary.CharacterCasing test = new NetLibrary.CharacterCasing();
textBox1.Text = test.UpperCase(textBox1.Text);
}
private void button2_Click(object sender, System.EventArgs e)
{
NetLibrary.CharacterCasing test = new NetLibrary.CharacterCasing();
textBox1.Text = test.LowerCase(textBox1.Text);
}
Enjoy !