Introduction
This article demonstrates how to create Azure Service Bus Queue Trigger from Visual Studio to receive the messages from service bus explorer.
Azure Functions
- Create a New Azure Functions Project
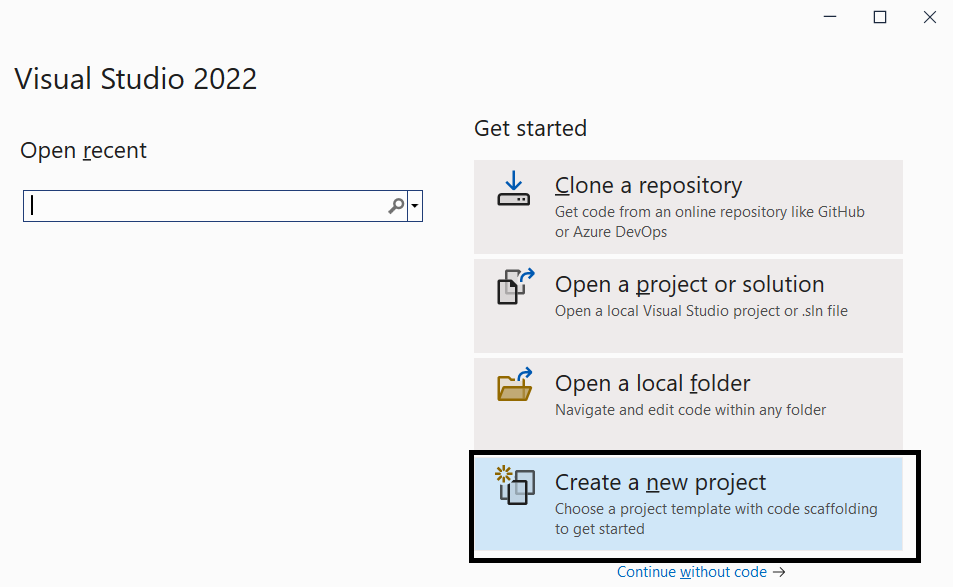
- Select the Azure Function and Click Next
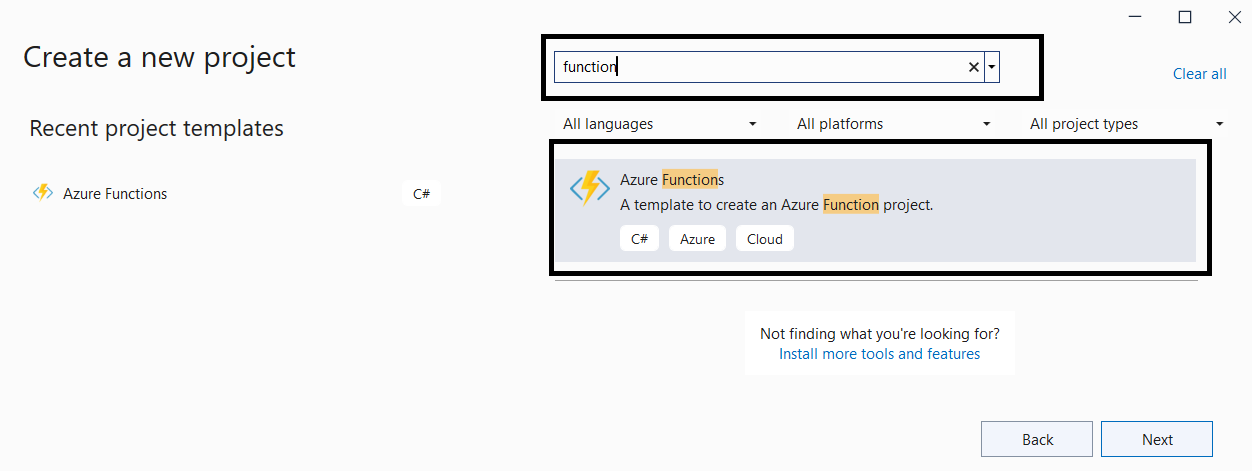
- Fill the Project Name and other values and click Next.
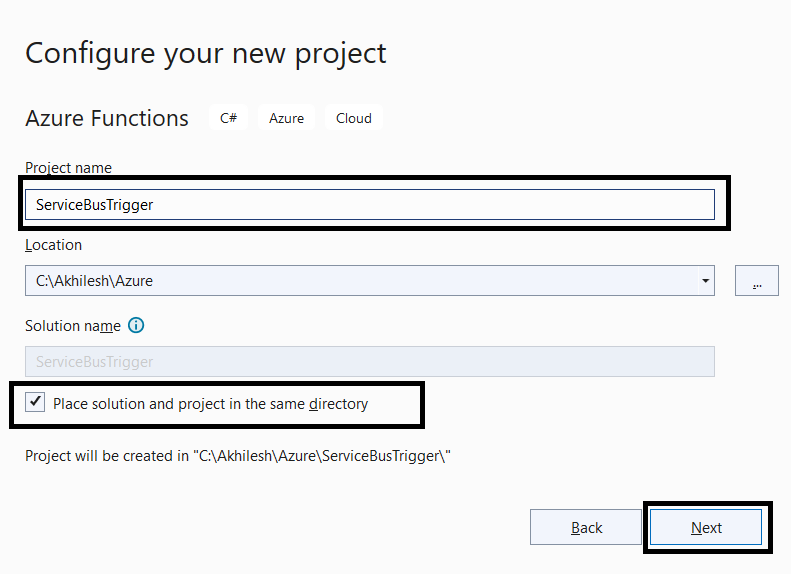
- There are already several features available in Azure Functions, each with a unique trigger. One feature that uses less code to consume the Service Bus is the Service Bus Queue Trigger.
- Add Additional Information, select the Function as Service Bus Queue Trigger and Fill the Connection String Setting name and Queue name.
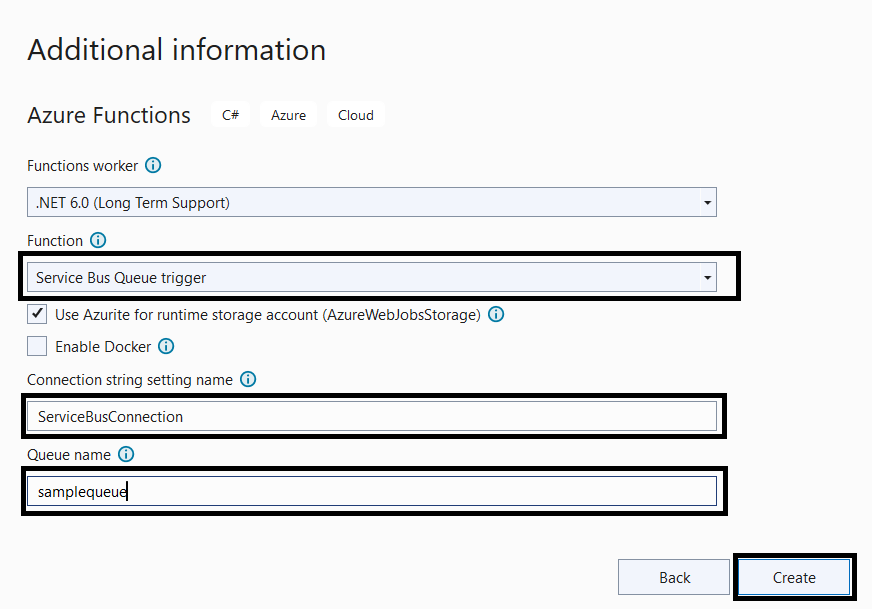
- Change the File Name, Class Name, or Function Name to what you want. I have updated the function to look like this.
public class ServiceBusTriggerFunction
{
[FunctionName("ServiceBusTriggerFunction")]
public void Run([ServiceBusTrigger("samplequeue", Connection = "ServiceBusConnection")]string myQueueItem, ILogger log)
{
log.LogInformation($"C# ServiceBus queue trigger function processed message: {myQueueItem}");
}
}
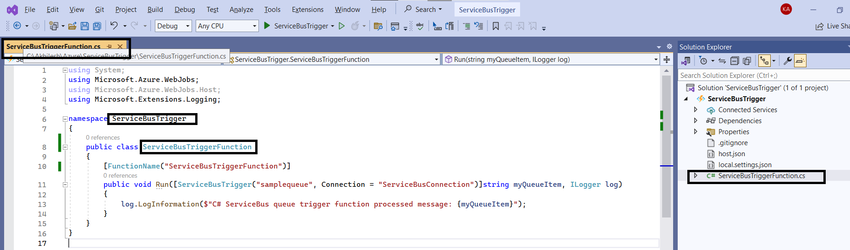
- Either the local.settings.json file or the function code itself can be used to set the Service Bus connection string and the Queue name. For cleaner code, we can use the local settings.json file. Let's log the configuration value as follows:
{
"IsEncrypted": false,
"Values": {
"ServiceBusConnection": "",
"AzureServicesAuthConnectionString": "",
"samplequeue": "samplequeue",
"FUNCTIONS_WORKER_RUNTIME": "dotnet"
}
}
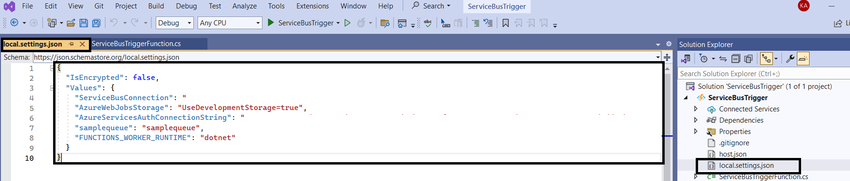
- Now that the configuration has been made, we can use the settings as follows in the code:
public static void Run([ServiceBusTrigger("%samplequeue%", Connection = "ServiceBusConnection")] string myQueueItem, ILogger log)
- Run the project, set a breakpoint, and we should receive the message as a string. After a message in the queue has been processed, it will, by default, no longer be visible on the service bus.
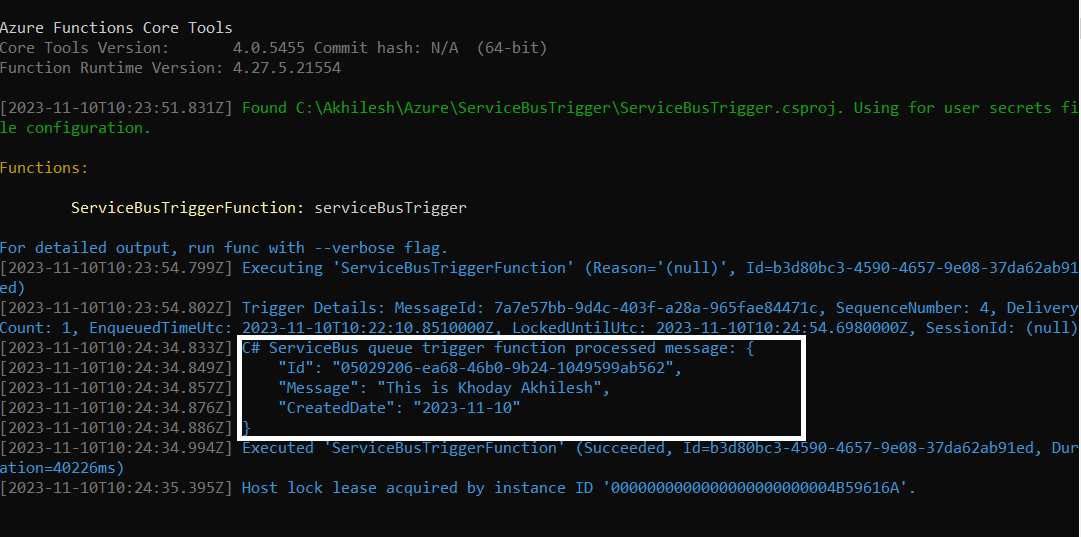
Summary
As we can see, Azure Functions provide us with the ability to handle Azure Service Bus Messages in a more efficient manner, requiring less code and a lower overall cost.