We can create a PDF in Silverlight using following steps.
Step 1: Create PDF in Silverlight.
Make classes in our application for e.g.:
- pdfclass()
- pdfpageclass()
- pdffonclass()
- pdftableclass()
Step a: Pdffontclass, in this class we have general font which we used for PDF.
public class PdfFontClass
{
public Font simplefont
{
get
{
// create a basecolor to use for the footer font, if needed.
Font font = FontFactory.GetFont("Arial", 8, Font.BOLD);
return font;
}
}
// Set Font style for paragraph.
public Font fontstyleforpara
{
get
{
// create a basecolor to use for the footer font, if needed.
Font font = FontFactory.GetFont("Myriad_Pro", 9);
return font;
}
}
// Set Font style for page header
public Font fontstyleforheader
{
get
{
// create a basecolor to use for the footer font, if needed.
Font font = FontFactory.GetFont("AGaramond",16 );
return font;
}
}
// Set Font style for weblink
public Font fontstyleforlink
{
get
{
// create a basecolor to use for the footer font, if needed.
Font link = FontFactory.GetFont("Arial", 12, Font.UNDERLINE, BaseColor.BLUE);
return link;
}
}
}
Step b: pdfpageClass, in this class we created pages. we can set footer, header and page size.
public override void OnStartPage(PdfWriter writer, Document doc)
{
PdfPTable headerTbl = new PdfPTable(1);
headerTbl.TotalWidth = doc.PageSize.Width;
Image logo = Image.GetInstance(imagepath + "/logo_new.jpg");
logo.ScalePercent(40f);
PdfPCell cell = new PdfPCell(logo);
cell.HorizontalAlignment = Element.ALIGN_RIGHT;
cell.PaddingRight = 20;
cell.Border = 0;
headerTbl.AddCell(cell);
headerTbl.WriteSelectedRows(0, -1, 0, (doc.PageSize.Height - 10), writer.DirectContent);
}
public override void OnEndPage(PdfWriter writer, Document doc)
{
PdfPTable footerTbl = new PdfPTable(2);
footerTbl.TotalWidth = doc.PageSize.Width;
footerTbl.HorizontalAlignment = Element.ALIGN_CENTER;
Paragraph para = new Paragraph(" Prefred System", footer);
//para.Add(Environment.NewLine);
//para.Add("Trustfort India PVT LTD");
PdfPCell cell = new PdfPCell(para);
cell.Border = 0;
cell.PaddingLeft = 10;
footerTbl.AddCell(cell);
para = new Paragraph(i+" of 4", footer); //--------set the toltal no. of pages in pdf
cell = new PdfPCell(para);
cell.HorizontalAlignment = Element.ALIGN_RIGHT;
cell.Border = 0;
cell.PaddingRight = 10;
footerTbl.AddCell(cell);
footerTbl.WriteSelectedRows(0, -1, 0, (doc.BottomMargin + 0), writer.DirectContent);
i++;
}
Step 2: PdfTableclass, in this class we create a table, with PdfPCell.
public PdfPTable GetSingleColumnTable()
{
PdfPTable Maintable = new PdfPTable(1);
Maintable.TotalWidth = 160f;
Maintable.LockedWidth = true;
PdfPTable table = new PdfPTable(1);
PdfPCell cell = new PdfPCell(new Phrase("Market highlights Q210", new Font(FontFactory.GetFont("Arial", 10, BaseColor.DARK_GRAY))));
invisiblecellBorder(cell);
string content = "· Volatility returned during the second quarter due, in part," +
" to fears over the European debt situation and a potential global slowdown.";
string content1 = ". Bonds remained strong, as stocks experienced a selloff.";
string content2 = "· Housing slipped, though the economy continued to show some signs of recovery.";
table.AddCell(cell);
createCell(table, content);
createCell(table, content1);
createCell(table, content2);
PdfPCell maincell = new PdfPCell(new PdfPTable(table));
maincell.BackgroundColor = new iTextSharp.text.BaseColor(System.Drawing.Color.LightSteelBlue);
Maintable.AddCell(maincell);
return Maintable;
}
Step 3: CreatePDF using the above classes
Document doc = new Document(PageSize._11X17);
Here we can set the page size like doc=new doc(pagesize.A4).
PdfWriter writer;
getChart gc = new getChart();
PdfTableClass pc = new PdfTableClass();
string path = "D:/pdf/";
string imagepath = "D:/employee pics/";
string imgMain = "pdfnew.png"; //---- Front Main Page IMAGE
string imgPPF = "emp1image.jpg";
public void CreatePdf(string filename, int accountID)
{
PdfFontClass pf = new PdfFontClass();
pdfPage page = new pdfPage();
writer=PdfWriter.GetInstance(doc, new FileStream(path + filename+".pdf", FileMode.Create));
writer.PageEvent = page;
doc.Open();
//Anchor anchor = new Anchor("http://www.xyz.com%22,%20fontstyleforlink/);
//anchor.Reference = "http://www.xyz.com/";
//doc.Add(anchor);
spacing(3);
Image giff = Image.GetInstance(imagepath + imgMain);
giff.Alignment = Image.ALIGN_BOTTOM;
doc.Add(giff);
doc.NewPage();
Image port = Image.GetInstance(imagepath + imgPPF);
port.Alignment = Image.ALIGN_LEFT;
doc.Add(port);
DrawLine(37, 120);
spacing(1);
doc.Add(new Paragraph("Your Annual Review", new Font(FontFactory.GetFont("AGaramond",30))));
string str = String.Format("{0:MMM/dd/yyyy}", DateTime.Now);
doc.Add(new Paragraph("As of " + str, new Font(FontFactory.GetFont("AGaramond", 12))));
spacing(1);
doc.Add(new Paragraph(" Client Accounts Details", pf.fontstyleforheader));
spacing(3);
}
By completing the application code we will get PDF.
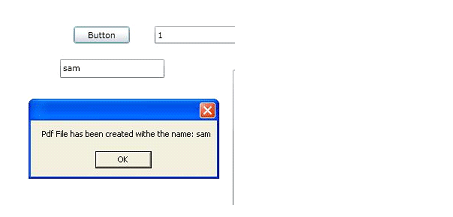
This PDF will be generated in the D:\Pdf folder; we mention the path in our application.
We can see the generated PDF. Open the PDF folder which is D:\ .
Summary : We can create the PDF in Silverlight using some PDF classes.