When i was working on ASP.NET application, one requirment was to have Resizable TextBox. I searched it and found in AjaxControlToolKit, but AjaxControlToolKit has Resizable Control which is actully a no editable container. Reforming this control to Resizable TextBox as UserControl is all about this article.
Again i am taking code provided by Microsoft, which is easily available here.
Step:1
Add reference of AjaxControlToolKit to your application. Create a user control name "ResizableTextBox" and add it to your project. Now place ResizableControlExender tag with textbox which should be within panel.
<asp:Panel ID="PanelText" runat="server" CssClass="frameText" > <asp:TextBox runat="server" ID="TextBoxResizable" CssClass="noborder" Width="100%" Height="100%" TextMode="MultiLine"> </asp:TextBox> </asp:Panel>
<cc1:ResizableControlExtender ID="ResizableTextBoxExtender" runat="server" TargetControlID = "PanelText" ResizableCssClass="resizingText" HandleCssClass="handleText" OnClientResizing = "OnClientResizeText" />
|
Here we have Panel control which contains textbox to resize. This textBox has 100% height and width (with TextMode MultiLine) becuase it has to fit within this panel irreespective of panel's height and width. Also TextBox is using "noborder" CSS class which is hiding its border.
ResizableControlExtender has TargetControlID to panel to handle resizing effect, OnClientResizing has attach with "OnClientResizeText" function which will be called during resizing of this panel.
Step:2
Now to get value from this textbox, either we have to search textbox within this usercontrol OR we can make property to get its value. Making properties is good choice. Simultaneously we have to create properties for control's minimum and maximum height/width. Lets start making all of these.
// Get or Set textbox's text public string Text { get {
return this.TextBoxResizable.Text; }
set {
this.TextBoxResizable.Text = value; } }
// Set
minimum height of text box public string MinimumHeight { get
{ return this.ResizableTextBoxExtender.MinimumHeight.ToString(); }
set {
this.ResizableTextBoxExtender.MinimumHeight =
int.Parse(value); } }
// Set minimum width of text box public string MinimumWidth { get
{ return this.ResizableTextBoxExtender.MinimumWidth.ToString();
}
set
{ this.ResizableTextBoxExtender.MinimumWidth = int.Parse(value);
} }
// Set maximum height of text box public string
MaximumHeight { set
{ this.ResizableTextBoxExtender.MaximumHeight = int.Parse(value);
} }
// Set maximum width of text box public string
MaximumWidth { set
{ this.ResizableTextBoxExtender.MaximumWidth = int.Parse(value);
} }
|
We are directly setting ResizableTextBoxExtender control's MinimumHeight, MinimumWidth, MaximumHeight, MaximumWidth using properties. Only MinimumHeight, MinimumWidth proerties has "get" which we will use for setting its default height and width.
Step:3
Now put this user control to your desired page with its properties, e.g.
<uc1:ResizableTextBoxControl id="ResizableTextBoxControl1" MinimumHeight="50"
MinimumWidth="100" MaximumHeight="300"
MaximumWidth="400"
runat="server"></uc1:ResizableTextBoxControl>
|
and when you will run your page, it will look like this
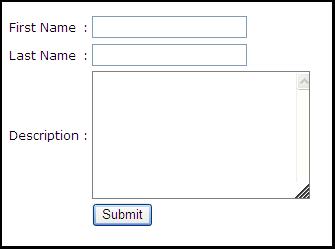
Before Resize
After Resize
Hope this article will fullfill the requirments of Resizable TextBox in ASP.NET applications.
Enjoy Coding!!!