Introduction
E-mail is used to send notification to customer nowadays, and since any smart developer try to send any notification in a professional format, one of these issues to send your notification in a template, this template might be your employer template or any other template you want to send it from your application.
Solution
Most of people when they trying to send any content to the e-mail, they use string builder object to concat all content then send it through mail object.
Regular approach:
StringBuilder sb =
new StringBuilder();
sb.Append("Data 1");
sb.Append("Data 2");
sb.Append("Data 3");
sb.Append("Data 4");
MailMessage message = new MailMessage();
message.Body = sb.ToString();
The cons of this approach is the developer has to concat all message content in the code and if there's any further updates he has to go and change it again in the code.
Enhanced Approach:
- Create htm/html, or aspx page with required template.
- Put any special character in content area (for example $).
- Load this page at runtime.
- Read the html content.
- Drop the required content inside the template (Read Trick Section).
- Assign the content as string to E-mail message body.
The pros of this approach are that you can anytime change or enhance the template without even touching the code.
The figure below shows template design.
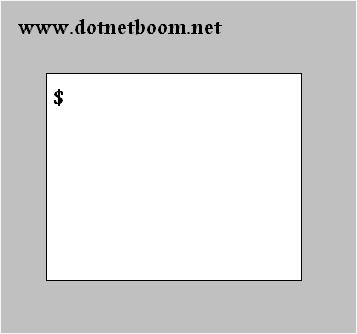
Trick
To set your content in the body area you have to flag it with special character or any other word which doesn't exist in any html document.
See the below code which give you how to do that:
string FullMessage = Template.Replace("$", sb.ToString());
Full method code:
public string GetNotificationContent()
{
string data = "Event=" + EventId;
StringBuilder sb = new StringBuilder();
sb.Append("Dear Mr./Mrs.");
sb.Append("<br>");
sb.Append("ANY CONTENT <br>");
sb.Append(data);
string Template = LoadTemplate();
string FullMessage = Template.Replace("$", sb.ToString());
return FullMessage;
}
public string LoadTemplate()
{
string emailPath = ConfigurationManager.AppSettings["EmailTemplate"].ToString();
StreamReader rdr = File.OpenText(emailPath);
try
{
string content = rdr.ReadToEnd();
return content;
}
catch
{
return "";
}
finally
{
rdr.Close();
rdr.Dispose();
}
}
Then you can use the string of full message to send it as a body on mail objects as follows:
MailMessage message = new MailMessage();
message.Body = sb.ToString();
Conclusion
This article is just to find a smart way to send a template to your e-mail.