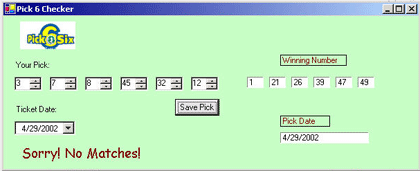
Figure 1 - Lottery Pick 6 Tracking Program
A few weeks ago, the New Jersey State lottery was up to 300,000,000 dollars. That's quite a large pot, and some lucky winner cashed in. If you come up with the winning number you have the option of taking a lesser pay-out in cash rather than the full annual payment.Of course, this is after the government takes its cut, but a few million dollars is nothing to sneeze at, and the money the government takes, goes to good causes such as education. Anyway, my girlfriend bought a ticket, and she kept asking me how to check the number. I decided that instead of waiting to watch a bunch of ping pong balls bounce around, it would be easier to take the lazy route and write a program that checked the winning number on the web against my girlfriend's number.If her 6 numbers matched all 6 of the winning numbers on the web, the program would happily proclaim that my girlfriend had won.
The program takes advantage of the fact that the New Jersey state lottery publishes its winning numbers on one of its web pages in a form that is fairly easy to parse.Below is the code for extracting the number of the lottery's web site.
private static string GetWinningNumbersFromWebSite()
{
// Web silte for Pick 6 Data
string fullpath = @http://www.state.nj.us/lottery/data/pick6.dat;
HttpWebRequest req;
HttpWebResponse res;
StreamReader sr;
string strResult;
try
{
// Create request for the web stream
req = (HttpWebRequest) WebRequest.Create(fullpath);
// Return request in a response stream
res = (HttpWebResponse) req.GetResponse();
sr = new StreamReader(res.GetResponseStream(), Encoding.ASCII);
//Put the results in one big string
strResult = sr.ReadToEnd();
sr.Close();
}
catch(Exception ex)
{
strResult = "Exception occured";
}
//return the results
return strResult;
}
The data is read in from a web stream on the New Jersey lottery web page. It is then placed in a string where the date and winning number information can be parsed out into a big 2 dimensional array. Each row of data represents a daily pick.The first 100 rows are extracted from the web site.In this program, only the most recent row (row 0) is used to determine a winner:
void PopulateData()
{
// Use a string reader to read each line
StringReader sr = new StringReader(webpage);
string[] lineArray;
for (int i = 0; i < 100; i++)
{
string nextLine = sr.ReadLine();
// split array into columns which is conveniently separated by the percent character
lineArray = nextLine.Split(new char[]{'%'});
for (int j = 0; j< lineArray.Length; j++)
{
// copy into a 2d array
RowArray[i, j] = lineArray[j];
}
}
}
This program also takes advantage of xml to save your personal ticket number in a file. Below is the format of the file to save both the number and the date of the drawing:
<?xml version="1.0" encoding="us-ascii" standalone="yes"?>
<numbers>
<number1>3</number1>
<number2>7</number2>
<number3>8</number3>
<number4>45</number4>
<number5>32</number5>
<number6>12</number6>
<date>4/29/2002</date>
</numbers>
The picks are saved using the XmlTextWriter, a convenient class for writing XML data to an xml file:
private void SavePicks()
{
// Save your picks from the up-down controls into an array
YourPick[0] = (int)numericUpDown1.Value;
YourPick[1] = (int)numericUpDown2.Value;
YourPick[2] = (int)numericUpDown3.Value;
YourPick[3] = (int)numericUpDown4.Value;
YourPick[4] = (int)numericUpDown5.Value;
YourPick[5] = (int)numericUpDown6.Value;
// Open a file stream in the application directory called picks.xml
string exepath = Application.ExecutablePath;
string exedir = exepath.Substring(0, exepath.LastIndexOf('\\'));
FileStream theStream = new FileStream(exedir + "\\" + "Picks.xml", FileMode.Create,
FileAccess.Write, FileShare.None);
// Create a XmlTextWriter on the file stream
XmlTextWriter sw = new XmlTextWriter(theStream, Encoding.ASCII);
// Put header info into the xml document
sw.WriteStartDocument(true);
sw.WriteStartElement("numbers");
// Write out all of the numbers to the xml file as elements number1, number2, number3..
for (int i = 0; i < 6; i++)
{
sw.WriteElementString("number" + (i+1).ToString(), YourPick[i].ToString());
}
// write out the date
sw.WriteElementString("date", this.dateTimePicker1.Value.ToString("M/dd/yyyy"));
// write xml document terminating elements to keep xml well-formed
sw.WriteEndElement();
sw.WriteEndDocument();
sw.Close();
// Display that the document has been saved
MessageBox.Show("Pick Saved.");
}
A timer is used to check to see if the drawing has been posted on the web every once in a while and if it matches the winning number.The event handler for the timer is shown below. (The code would probably look alot cleaner if both the numericUpDown controls and the number labels were placed in ArrayLists.Then the code would be only a few lines long.)
private void timer1_Tick(object sender, System.EventArgs e)
{
int match = 0;
// If the pick date matches the ticket drawing date, count the number of matches
// and display them.
if (this.dateTimePicker1.Text == this.PickDateLabel.Text)
{
if (numericUpDown1.Value == Convert.ToInt32(num1label.Text))
{
match++;
}
if (numericUpDown2.Value == Convert.ToInt32(num2label.Text))
{
match++;
}
if (numericUpDown3.Value == Convert.ToInt32(num3label.Text))
{
match++;
}
if (numericUpDown4.Value == Convert.ToInt32(num4label.Text))
{
match++;
}
if (numericUpDown5.Value == Convert.ToInt32(num5label.Text))
{
match++;
}
if (numericUpDown6.Value == Convert.ToInt32(num6label.Text))
{
match++;
}
MatchLabel.Text = GetMatchString(match);
}
Summary
It can probably be concluded from this article, that this program could be expanded to check and display results for any lottery whose state is kind enough to post the winning results on a website.Although this is just one example, this article should give you a pretty fair idea how you can use .NET to capture internet data and analyze it any way you wish.