In this article, we will look into Visual Studio 2008 new feature called as tracepoints. Tracepoints is a handy feature in logging the values in debugging mode. In earlier versions of Visual Studio, we use console.writeline statement or write to a file using stream objects for logging the values of a variable. But, later we need to remove those statements while delivering the code. In order to resolve this kind of troubles, visual studio came up with tracepoints. We can use tracepoints to print variable's values to Output window's Debug pane. Most of us use debugging, to check whether the values coming are correct or not. But, few applications might change their behavior like multi-threaded while debugging it. For this kind of applications, we can use tracepoints to log values of variables, sequence of functions called.
We will look into this feature deeply with examples. Create a sample console application and name it as TracepointsApp as shown below:
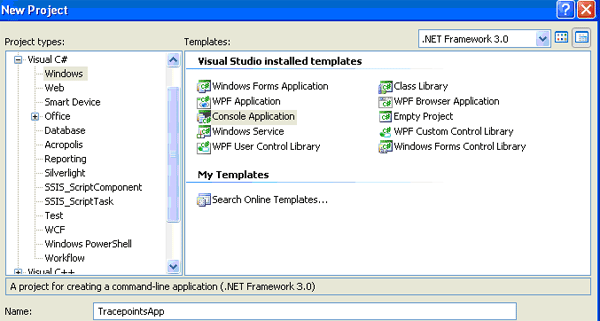
Now add below lines to Main method for doing a sum of three numbers.
namespace TracepointsApp
{
class Program
{
static void Main(string[] args)
{
int a1 = 0, b1 = 0, c1 = 0,sum=0;
Console.WriteLine("Enter a1");
a1 = Convert.ToInt32(Console.ReadLine());
Console.WriteLine("Enter b1");
b1 = Convert.ToInt32(Console.ReadLine());
Console.WriteLine("Enter c1");
c1 = Convert.ToInt32(Console.ReadLine());
sum = a1 + b1;
sum = sum + c1;
Console.WriteLine(String.Format("Sum is {0}",sum));
Console.ReadLine();
}
}
}
Now, we will add a tracepoint to get sum of a1 and b1. Follow the below steps to do it.
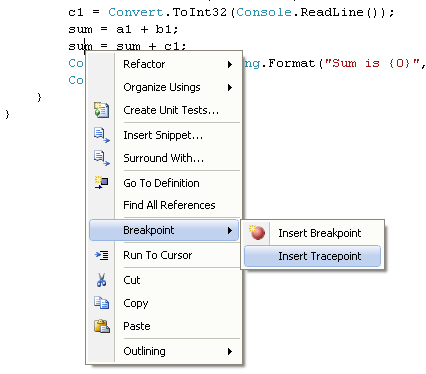
It will show a popup for setting the values, that to be displayed in Output's Debug pane. We can display value of variables by specifying it in between {}. Here, I want only value of sum of 2 numbers and function name. So, the message to be printed will be:
Function: $FUNCTION, Sum of a1 and b1 :{sum}
We can uncheck "Continue execution" for breaking the execution on reaching the tracepoint. Run the application and clear contents of Output's Debug pane.
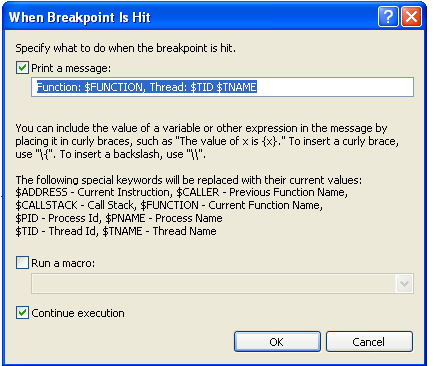
The output will be as shown below:
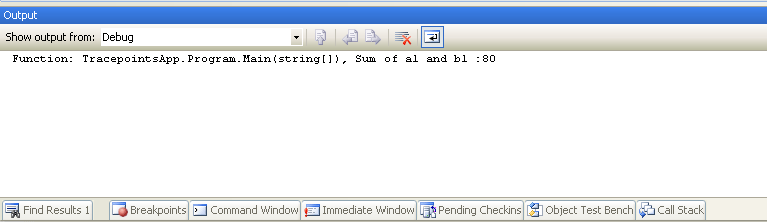
In this way, we can log other values like thread name, caller function name etc without breaking the execution of code.
Below is the list of keywords that can be used in tracepoints:
Keyword |
Value |
{x} |
Print variable x value |
$CALLER |
Previous Function Name |
$TNAME |
Thread Name |
$TID |
Thread ID |
$PNAME |
Process Name |
$PID |
Process ID |
SFUNCTION |
Current Function Name |
$CALLSTACK |
Call Stack |
$ADDRESS |
Current Statement |
I am ending up the things here. I hope this article will be helpful for all.