Introduction:
This article describes a simple approach to maintaining control state in an ASP.NET 2.0 custom web control. Control state is a new construct within ASP.NET 2.0 and it is really nothing more than view state however it is view state with a significant advantage; that advantage is that other developers using your control cannot disable control state as they can view state.
Control state survives even if the developer using the custom control disables view state. The advantage is pretty obvious; in prior versions of Visual Studio, the consumer of a custom control could disable view state; if the control relied on view state to maintain state information, the control would cease to function or at least misbehave. Creating control state removed the ability of a control consumer to disable view state based state management within the control; naturally the control designer may still opt to not use either view state or control state based state management.
One final note, most of the examples I have personally viewed regarding control state show a mechanization in which only one value is stored in the control state; the accompanying example herein shows how store a greater number of values of different data types can be easily stored in the control state.
Getting Started:
In order to get started, open up the Visual Studio 2005 IDE and start a new project. From the new project dialog (Figure 1), under project types, select the "Windows" node from beneath "C#", then select the "Web Control Library" template in the right hand pane. Key in a name for the project and then click "OK".
Once the project has opened; right click on the solution and click on the "Add" menu option, and then select "New Item". When the "Add New Item" dialog appears (Figure 2), select the "Web Custom Control" template, after selecting the template, key "ExampleStateControl.cs" into the name field and then click "Add" to close the dialog. You may now delete the default web control that was created when the project was originally initialized from the template.
At this point, we should have an open web control library project with a single web control named "ExampleStateControl.cs" in that project.
One last step prior to writing the code for this project will be to validate the references and import statements. Compare your import statements with this list and make any adjustments necessary:
using System;
using System.ComponentModel;
using System.Web;
using System.Web.UI;
using System.Web.UI.WebControls;
Figure 1: Visual Studio 2005 New Project Dialog
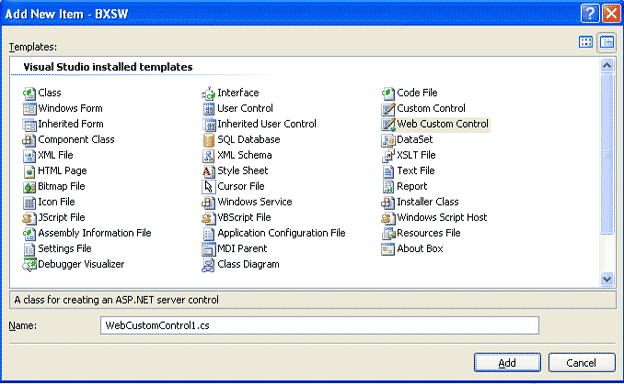
Figure 2: Add New Item Dialog
We are now ready to add the code necessary to make this control functional. At this point, the project's code should look something like this:
using System;
using System.ComponentModel;
using System.Web;
using System.Web.UI;
using System.Web.UI.WebControls;
[Serializable() ]
[ToolboxData("<{0}:ExampleStateControl runat=server></{0}:ExampleStateControl>")]
public class ExampleStateControl : WebControl
If we are in sync here, we can now start to write the necessary code. To begin, create a declarations region and add the following code to that region:
#region "Declarations"
private CurrentProperties mCurrentProps = new CurrentProperties();
[Serializable()]private struct CurrentProperties
{
public string pFirstName;
public string pLastName;
public int pAge;
public string pCity;
public string pState;
public string pCountry;
public bool pCitizen;
}
#endregion
In examing the declarations, note that there is a structure called CurrentProperties defined and there is a private member variable declared (mCurrentProps) that is of the CurrentProperties structure type. This instance of the structure is what we will be putting into and collecting out of control state.
The next thing to do will be to add the properties necessary to support the example custom web control. There is one property for each element contained in the structure. To accomplish this task, create a properties region and add in the following code:
#region "Properties"
[Browsable(true)]
[Category("Name")]
[DefaultValue("")]
[Localizable(true)]
[NotifyParentProperty(true)]
public string FirstName
{
get
{
return mCurrentProps.pFirstName;
}
set
{
mCurrentProps.pFirstName = value;
SaveControlState();
}
}
[Browsable(true)]
[Category("Name")]
[DefaultValue("")]
[Localizable(true)]
[NotifyParentProperty(true)]
public string LastName
{
get
{
return mCurrentProps.pLastName;
}
set
{
mCurrentProps.pLastName = value;
SaveControlState();
}
}
[Browsable(true)]
[Category("Status")]
[DefaultValue("")]
[Localizable(true)]
[NotifyParentProperty(true)]
public int Age
{
get
{
return mCurrentProps.pAge;
}
set
{
mCurrentProps.pAge = value;
SaveControlState();
}
}
[Browsable(true)]
[Category("Status")]
[DefaultValue("")]
[Localizable(true)]
[NotifyParentProperty(true)]
public bool IsCitizen
{
get
{
return mCurrentProps.pCitizen;
}
set
{
mCurrentProps.pCitizen = value;
SaveControlState();
}
}
[Browsable(true)]
[Category("Residence")]
[DefaultValue("")]
[Localizable(true)]
[NotifyParentProperty(true)]
public string City
{
get
{
return mCurrentProps.pCity;
}
set
{
mCurrentProps.pCity = value;
SaveControlState();
}
}
[Browsable(true)]
[Category("Residence")]
[DefaultValue("")]
[Localizable(true)]
[NotifyParentProperty(true)]
public string State
{
get
{
return mCurrentProps.pState;
}
set
{
mCurrentProps.pState = value;
SaveControlState();
}
}
[Browsable(true)]
[Category("Residence")]
[DefaultValue("")]
[Localizable(true)]
[NotifyParentProperty(true)]
public string Country
{
get
{
return mCurrentProps.pCountry;
}
set
{
mCurrentProps.pCountry = value;
SaveControlState();
}
}
#endregion
Note that each property's get and set methods either updates or retrieves from the active instance of the "ControlProps" structure. No other private member variables are used to retain each of the property's current values.
The next step in the process is to add in the methods used by the control. Add a Methods region to the code page and key in the following methods:
#region "Methods"
protected override void OnInit(System.EventArgs e)
{
Page.RegisterRequiresControlState(this);
base.OnInit(e);
}
protected override object SaveControlState()
{
return this.mCurrentProps;
}
protected override void LoadControlState(object savedState)
{
mCurrentProps = new CurrentProperties();
mCurrentProps = (CurrentProperties) savedState;
}
#endregion
Notice that in the init, the first line is used to instruct the page to maintain control state. The next two methods are used to save the current control state or to reload the control states values from the saved control state.
At this point, the only thing left to do is to define how the control will be rendered. To complete this step, create a "Rendering" region and, within this region, override the RenderContents sub with the following code:
#region "Rendering"
protected override void RenderContents(System.Web.UI.HtmlTextWriter writer)
{
try
{
writer.RenderBeginTag(HtmlTextWriterTag.Table);
writer.Write("<b>Control State Properties</b><hr />");
///''''''''''''''''''''''''''''''''''''''''''
writer.RenderBeginTag(HtmlTextWriterTag.Tr);
// first column
writer.RenderBeginTag(HtmlTextWriterTag.Td);
writer.Write("Name: ");
writer.RenderEndTag();
// second column
writer.RenderBeginTag(HtmlTextWriterTag.Td);
writer.Write(mCurrentProps.pFirstName + " " + mCurrentProps.pLastName);
writer.RenderEndTag();
///'''''''''''''''''''''''''''''''''''''''''
writer.RenderBeginTag(HtmlTextWriterTag.Tr);
// first column
writer.RenderBeginTag(HtmlTextWriterTag.Td);
writer.Write("Age: ");
writer.RenderEndTag();
// second column
writer.RenderBeginTag(HtmlTextWriterTag.Td);
writer.Write(mCurrentProps.pAge);
writer.RenderEndTag();
writer.RenderEndTag();
///'''''''''''''''''''''''''''''''''''''''''
writer.RenderBeginTag(HtmlTextWriterTag.Tr);
// first column
writer.RenderBeginTag(HtmlTextWriterTag.Td);
writer.Write("Is Citizen: ");
writer.RenderEndTag();
// second column
writer.RenderBeginTag(HtmlTextWriterTag.Td);
writer.Write(mCurrentProps.pCitizen.ToString());
writer.RenderEndTag();
writer.RenderEndTag();
///'''''''''''''''''''''''''''''''''''''''''
writer.RenderBeginTag(HtmlTextWriterTag.Tr);
// first column
writer.RenderBeginTag(HtmlTextWriterTag.Td);
writer.Write("City: ");
writer.RenderEndTag();
// second column
writer.RenderBeginTag(HtmlTextWriterTag.Td);
writer.Write(mCurrentProps.pCity.ToString());
writer.RenderEndTag();
writer.RenderEndTag();
///'''''''''''''''''''''''''''''''''''''''''
writer.RenderBeginTag(HtmlTextWriterTag.Tr);
// first column
writer.RenderBeginTag(HtmlTextWriterTag.Td);
writer.Write("State: ");
writer.RenderEndTag();
// second column
writer.RenderBeginTag(HtmlTextWriterTag.Td);
writer.Write(mCurrentProps.pState.ToString());
writer.RenderEndTag();
writer.RenderEndTag();
///'''''''''''''''''''''''''''''''''''''''''
writer.RenderBeginTag(HtmlTextWriterTag.Tr);
// first column
writer.RenderBeginTag(HtmlTextWriterTag.Td);
writer.Write("Country: ");
writer.RenderEndTag();
// second column
writer.RenderBeginTag(HtmlTextWriterTag.Td);
writer.Write(mCurrentProps.pCountry.ToString());
writer.RenderEndTag();
writer.RenderEndTag();
///'''''''''''''''''''''''''''''''''''''''''
writer.RenderEndTag(); // close table
}
catch (Exception)
{
writer.Write(this.ID);
}
}
#endregion
As you can see, the rendering of this example control is pretty simple; it is just generating a table and displaying each control state maintained property value in separate rows within that table.
The control is now complete. Prior to testing the control, rebuild the project. Once that has been completed and any errors encountered are repaired, it is time to test the control. To test the control, add a new web site project to the web control library project currently open. Once the test web site has been created, set the test project as the start up project by right clicking on the web site solution in the solution explorer and selecting the "Set as Start Up Project" menu option. Next, locate the Default.aspx page in the web site solution, right click on this page and select the "Set as Start Page" menu option.
If you downloaded the sample project, you may prefer to open IIS and create a virtual directory pointing to the web site and then open the project from within Visual Studio 2005. The solution contains both the sample web site and the sample custom web control library project.
Open the Default.aspx page for editing. Locate the newly created control in the toolbox (it should be at the top) and, if you are not working with the sample web site, drag the "ExampleStateControl" control onto the page (Figure 3).
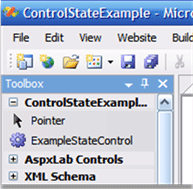
Figure 3: Custom Control in the Toolbox
If you are working with the sample code, you may still click on the control and set its properties through the property grid within the Visual Studio 2005 IDE if you wish to do so.
Build the application and run it; you should now be looking at the site displayed in the following figure (Figure 4). With the page running, you may key in new values into the spaces provided in the lower half of the page and hit the enter key or click on the submit button to force a post back. In either case, the application will update the current properties and save the control state; after the post back has finished you will note that the values stored in the control state have been used in the rendering of the control.
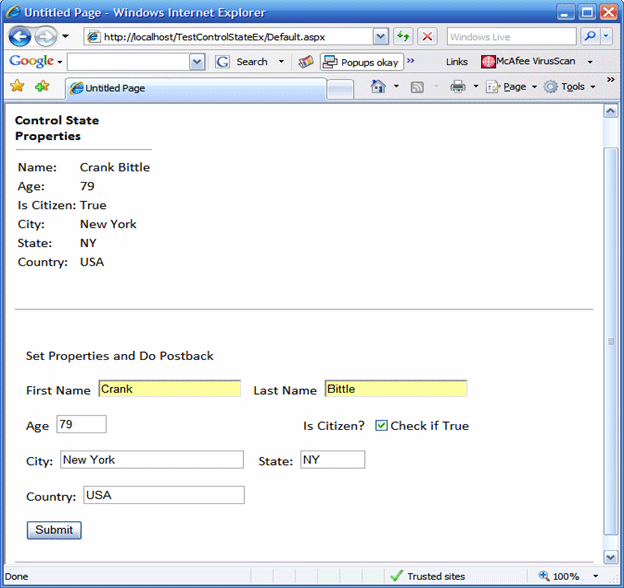
Figure 4: Control state managed control in operation
NOTE: THIS ARTICLE IS CONVERTED FROM VB.NET TO C# USING A CONVERSION TOOL. ORIGINAL ARTICLE CAN BE FOUND ON VB.NET Heaven (http://www.vbdotnetheaven.com/).