Overview
The .NET framework library provides thousands of classes and other objects, that allow us (developers) to build operating system independent applications. However, .NET is a new technology and it's not very complete yet. There are some areas where managed code is not available and one of those areas is Multimedia devices. But fortunately the .NET Framework provides COM Interoperability services, which can be used to access any COM component in managed code. In this article Ill try to explain the issue of the COM Interoperability and how to use Multimedia Device controls in manage code.
Description
In this article, Ill show how to use (just a basic example) the Multimedia Device Control. The first step is to import the ActiveX control in the Visual Studio Environment.
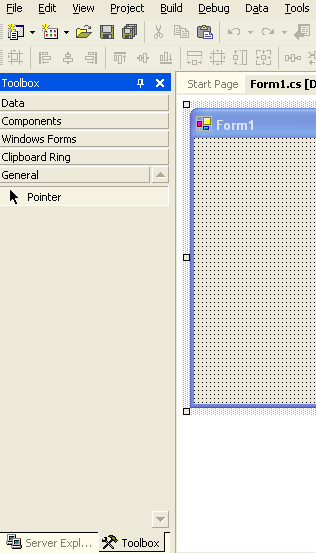
Click with the right button on the toolbox, and choose customize toolbox:
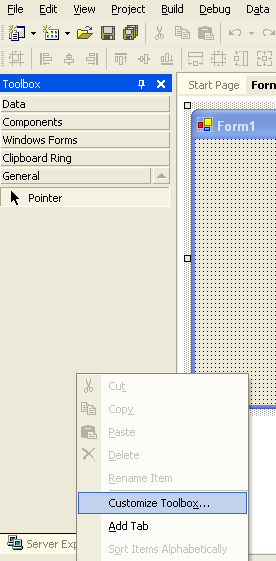
and then Window Multimedia Control 6:
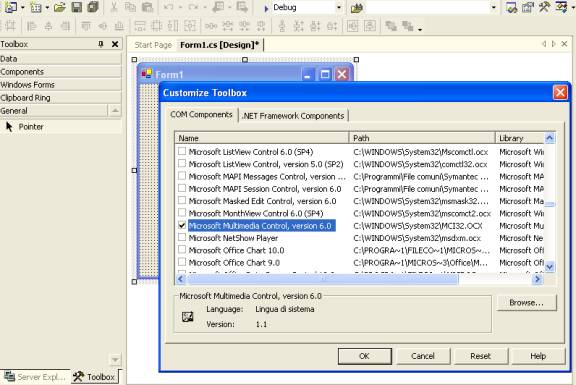
you will have:
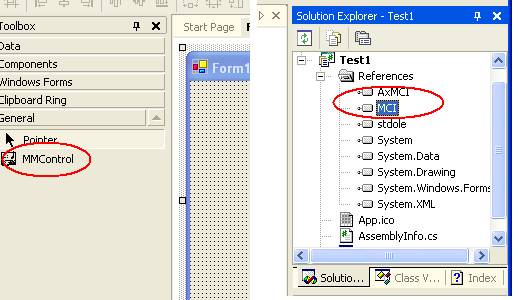
In this way, Visual Studio will have imported for you the ActiveX Control.
Now lets make the program, let put the MCI Control, 4 buttons, a label, and a openFileDialog.
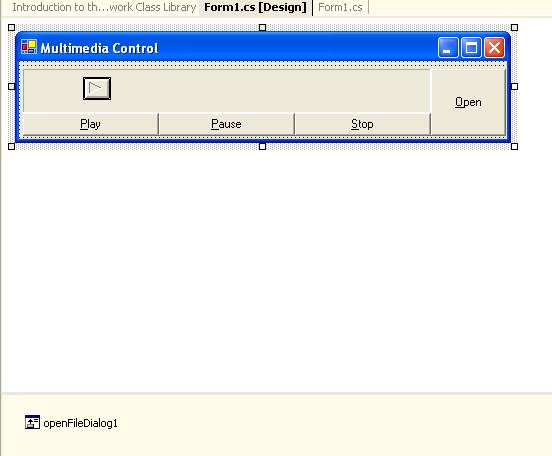
Now lets have a look at the code:
private
void button1_Click(object sender, System.EventArgs e)
{
// just write how to filter into the dialog box
openFileDialog1.Filter = "File Musicali (*.wav, *.wma) | *.wav; *wma";
// then open it
openFileDialog1.ShowDialog();
// and finally write in the label the selected file.
label1.Text = openFileDialog1.FileName;
}
the first step is to open the MMC and the you can use it. In this case e.g.: axMMControl1.Command = Open; and then
axMMControl1.Command = Play
you have many possibility, as Pause, Stop, Eject, etc.
private
void button2_Click(object sender, System.EventArgs e)
{
string btn = ((Button)sender).Name;
switch (btn)
{
case "btnPlay":
if(label1.Text != "")
{
axMMControl1.Command = "Close";
axMMControl1.FileName = openFileDialog1.FileName;
axMMControl1.Command = "Open";
axMMControl1.Command = "Play";
}
else
{
MessageBox.Show("you have to select a file...","Media Control",MessageBoxButtons.OK,MessageBoxIcon.Information);
button1.Focus();
}
break;
case "btnPause":
axMMControl1.Command = "Pause";
break;
case "btnStop":
axMMControl1.Command = "Stop";
break;
}
}
private void Form1_Closed(object sender, System.EventArgs e)
{
axMMControl1.Command = "Stop";
axMMControl1.Command = "Close";
}