Everyone wants to know at what time they are starting and shutdown their system daily. How much time, system is running daily. It would be better to show startup, shutdown and time spent timings in a DataGrid.
Here, I am providing a solution to do this using Windows Service in C#.NET. Everyone knows about Windows Service. But for introduction sake, I will explain little bit about Windows Service. Then, we can see how to design the application.
What is a Windows Service?
Windows Service is nothing but process running in background without our knowledge. It mostly won't require User interaction. If we type "services.msc" in Run of Start Menu, we can see what are the services running in our system. Some will start automatically, when system starts. But some services has to be started by us manually.
Advantages of Windows Service:
- It can be run automatically.
- It wont require User-Interaction
- It runs in background
Normally, Windows Service is used to run time-consuming processes, like taking backup of a database.
Now, we are going to design a windows service to record startup and shutdown timings of your system. I had designed this application using Visual Studio 2003.
Step 1:
First open Visual Studio and select Visual C# Projects. Select Template as Windows Service. Give name as Monitoring as shown in figure below:
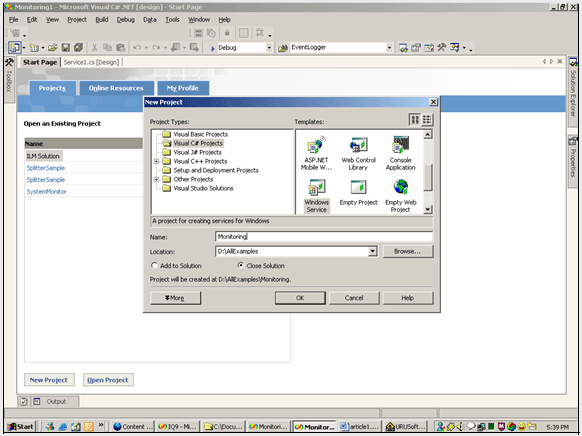
Step 2:
Open Code Window by pressing F7. Next include using System.IO namespace for writing system timings into a file. Next find Service1 word and replace all occurrences with Monitoring word. It will change namespace, classname, constructor name... to Monitoring. After that go to design mode(by pressing shift+F7). Select Solution Explorer(by pressing Ctrl+Alt+L). Click on Service1.cs and rename it to Monitoring.cs.
After that go to code window. And write this code in OnStart event.
As shown in figure:
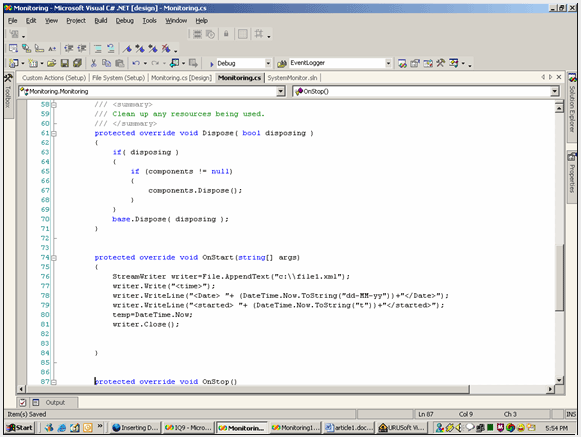
I will explain what I am doing in OnStart event:
First create a xml file in c drive with file1 as name.And write this code in that file:
<?
xml version="1.0" encoding="utf-8" standalone="no"?>
<times>
And close it.
I am creating a service, which will start automatically start and record startup time. It will shutdown, when system shutdowns. And it will record shutdown time and time spent on system.
First, I am creating StreamWriter to write startup time of system into file1.xml.
After copying the code to your OnStart event. Create a public variable temp, just above Monitoring Constructor as shown below:
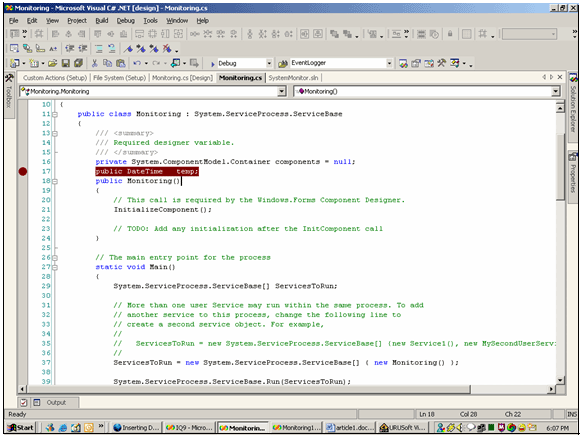
After that, copy this code into your code window:
Copy this code to OnStart event as shown in figure:
StreamWriter writer=File.AppendText("d:\\file1.xml");
writer.Write("<time>");
writer.WriteLine("<Date> "+ (DateTime.Now.ToString("dd-MM-yy"))+"</Date>");
writer.WriteLine("<started> "+ (DateTime.Now.ToString("t"))+"</started>");
temp=DateTime.Now;
writer.Close();
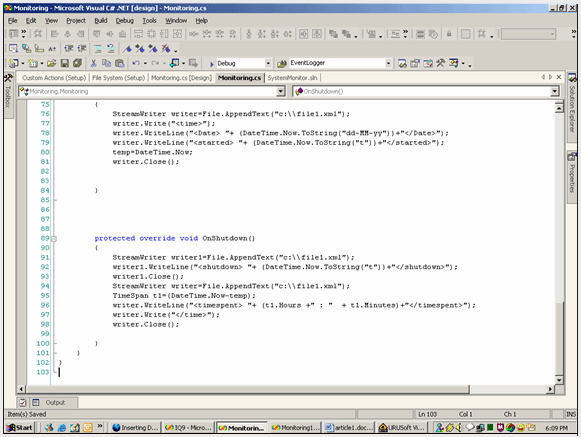
I will explain what I am doing in OnShutdown event:
I again opened file1.xml using a StreamWriter, when system is going to shutdown. It will write shutdown time. It will also write timespan between system startup and shutdown timings. I used a temp variable in OnStart and OnShutdown event. It is used in OnStart to store Startup time. It is used again OnShutdown event, to know time interval between start and shutdown timings.
Next, we have to configure our Windows Service for installation and running.
So, first go to design view and select properties(by pressing F4) window.
Set CanStop and CanShutdown to true. Next to install, we have to add a Installer.
So, right-click on design view window. Select Add Installer. Then, it will show ProjectInstaller.cs. In that, serviceInstaller1 and serviceProcessInstaller1 are present. Go to serviceProcessInstaller1 properties:
- Set Account to LocalSystem.
- Next goto serviceInstaller1 properties:
- Set DisplayName and ServiceName to Monitoring.
- Set StartType:automatic.
See below figure for reference:
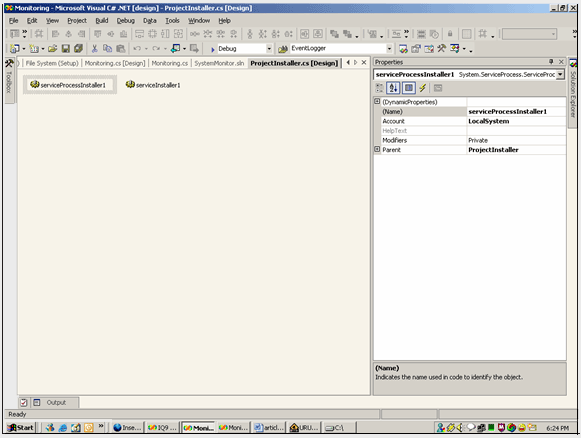
Next go to Command Prompt of VS.NET.
Go to Debug folder path of Service in console:
Type InstallUtil Monitoring.exe
If it shows Commit Phase Completed Successfully. Then, service is installed without errors.
Then, restart the system to start service. After restarting, open file1.xml. It has recorded start time of system. If shutdown system, it will record system shutdown time and time spent on system. By this part, we completed recording of timings of system. Next, we will create a web application to display the xml data in a Grid properly.
In next part, I will explain how to extract that xml file data and show it in a DataGrid as shown below:
Part-II:
Create a web application in C#. Name it as SystemMonitor.
Next include following namespaces:
using System.IO;
using System.Xml;
using System.Text;
After that drag DataGrid and Label control onto the page as shown below:
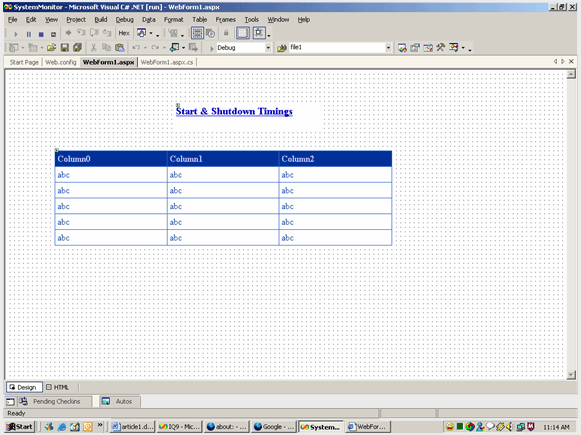
Next copy following code into Page_Load event:
File.Copy("c:\\file1.xml","c:\\temp1.xml",true);
StreamWriter writer1=File.AppendText("c:\\temp1.xml");
writer1.Write("<shutdown>undefined</shutdown>");
writer1.Write("<timespent>undefined</timespent>");
writer1.Write("</time>");
writer1.WriteLine("</times>");
writer1.Close();
DataSet ds=new DataSet();
ds.ReadXml("c:\\temp1.xml");
TimeSpan t=new TimeSpan();
DataGrid1.DataSource=ds;
DataGrid1.DataBind();
XmlTextReader reader=new XmlTextReader("c:\\temp1.xml");
while(reader.Read())
{
if(reader.NodeType==XmlNodeType.Element)
{
if(reader.Name=="timespent")
{
string temp11=reader.ReadInnerXml().ToString();
if(temp11!="undefined")
{
temp11=temp11.Replace(":",".");
temp11=temp11.Replace(" ","");
duration +=Convert.ToDouble(temp11);
}
}
}
}
Response.Write("Total Duration is : ");
double temp122=Convert.ToDouble(duration);
string hr=temp122.ToString();
string hrstr=hr.Substring(0,hr.IndexOf("."));
Response.Write(hrstr.ToString()+" Hours");
string mins=hr.Substring(hr.IndexOf(".")+1,(hr.Length -hr.IndexOf(".")-1));
Response.Write(" "+mins.ToString()+" Minutes");
reader.Close();
File.Delete("c:\\temp1.xml");
Next, paste this declaration above Page_Load event:
private double duration;
I will explain what I have done in Page_Load event:
First, I copied contents of file1.xml(which contains timings of system) to temporary file. Then, I am appending some tag to that temp file. Afterwards, I am reading contents of temp into a dataset and finally binding it to a DataGrid. Afterwards, I written logic to find total time spent. I hope, everyone can understand that code.
Finally, the page output will be like this:
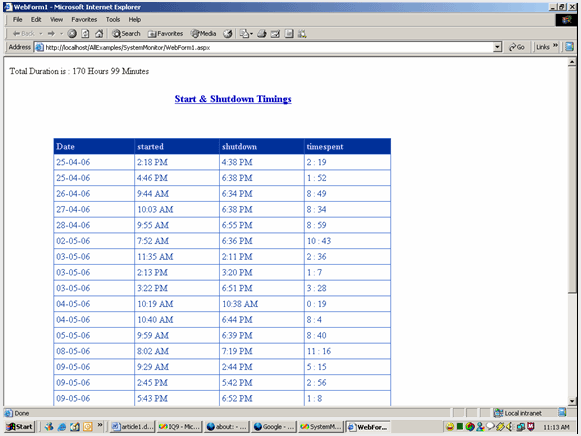