This article is continuation of my previous article on Populating Dropdown list in ASP.NET MVC 2 using Entity Framework 4 @ http://www.c-sharpcorner.com/UploadFile/2124ae/5628/
Here I will demonstrate Cascading dropdown list (populating a child dropdown based on selection of a parent dropdown) .
We will be using ajax method in Jquery by using JSON.
Over the course of this article - We will also see how to update Models using the Update Model Wizard in Entity Framework 4.0
I would be referring to database, Models, Views and Controllers used in my previous article @ (http://www.c-sharpcorner.com/UploadFile/2124ae/5628/)
and even the code discussed below would be an addition to the existing sample of the previous article.
1. Update ViewModel
Open IndexViewModel.cs and add code for second dropdown- Cities Drop down list which would serve as child dropdown for the State dropdown (parent dropdown).
//2nd DropDownList ID
public string ddlCityId
{
get;
set;
}
//2nd DropDownList Values
public List<SelectListItem> CityValue
{
get;
set;
}
2. Database part
- Here we will be creating a new table for cities.
- Create a new table -> tblCity (CityId(int - primary key, CityName - varchar(30),StateName-varchar(30)) in the existing database - SampleDB.mdf
- Insert sample cities for each corresponding state values(from tblState) in tblCity.
3. Models
Update Model
- We can use Update Model wizard to update the SampleDBModel.edmx file.
Update Model Wizard serves for the following purpose:
- ADD -> if an object has not been included in the existing model or if an object has been newly added to database - We can add the object to the Model.
- REFRESH -> if any of objects definition has been changes in the database -> We can update the object definition in the Model.
- DELETE -> in case any object (table, stored procedure, Views) has been deleted from database, it removes the object from the Model
- Here we will use the Update Model wizard to Add -> to basically include tblCity in our Model.
- Open SampleDBModel.edmx file.
- Right Click the Model browser window and select Update Model from database.
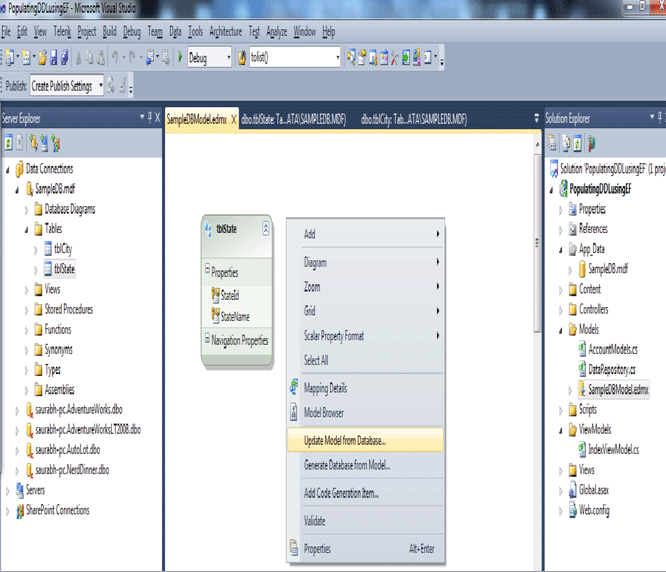
- Add tblCity and click Finish -> With this our model is updated with the new table.
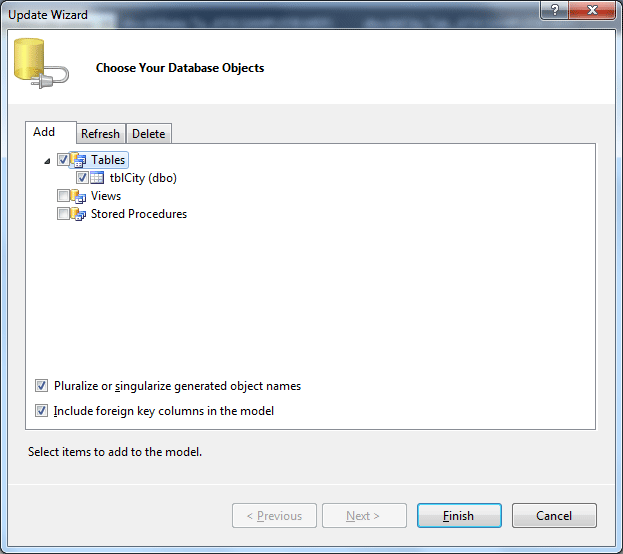
Update Repository Class
- Open DataRepository.cs from Models folder
- Include Code for fetching Cities based on Selected State Name
//Get the values for City DropDownlist based on Selected State Names
public List<SelectListItem> GetCityNames(string state_Selected)
{
var vCityName = (from tblCity in entities.tblCities
where tblCity.StateName==state_Selected
select new SelectListItem
{
Text=tblCity.CityName,
Value=tblCity.CityName
}
);
return vCityName.ToList();
}
4. Controllers
- Open HomeController.cs file from Controllers folder
- Inside ActionResult Index() get the first item in the state dropdown
//Get the first item of the First drop down list(State ddl)
string ddlStateId_First_Item = objIndexViewModel.StateValue[0].Value;
- Inside ActionResult Index() - for populating the Cities ddl - on the page load - based on first item in the state ddl
//Get the City names based on the first Item in the State ddl
objIndexViewModel.CityValue = objRepository.GetCityNames(ddlStateId_First_Item);
- Write a Controller action that returns a JSONRESULT - to return a list of cities based on selected Sate.
public JsonResult Cities_SelectedState(string Sel_StateName)
{
JsonResult result = new JsonResult();
var vCities = objRepository.GetCityNames(Sel_StateName);
result.Data = vCities.ToList();
result.JsonRequestBehavior = JsonRequestBehavior.AllowGet;
return result;
}
5. Views
- Open Index.aspx from Views -> Home -> Views folder.
- update it with the new Cities label and Cities dropdown
<%:Html.Label("Cities:") %>
<%:Html.DropDownListFor(m=>m.ddlCityId,Model.CityValue)%>
- Include JQuery to populate Cities ddl based on State ddl change.
<script type="text/javascript">
$(document).ready(function () {
$("#ddlStateId").change(function () {
var url = '<%= Url.Content("~/") %>' + "Home/Cities_SelectedState";
var ddlsource = "#ddlStateId";
var ddltarget = "#ddlCityId";
$.getJSON(url, { Sel_StateName: $(ddlsource).val() }, function (data) {
$(ddltarget).empty();
$.each(data, function (index, optionData) {
$(ddltarget).append("<option value='" + optionData.Text + "'>" + optionData.Value + "</option>");
});
});
});
});
</script>
- Open Site.Master (master page) from Views ->Shared folder and include jQuery script files inside Head Section.
<head runat="server">
<script type="text/javascript" src="../../Scripts/jquery-1.4.1.js"></script>
</head>
6. Compile And Run the Project (F5) - We can see on Page Load City Dropdown got populated based on first item in the State dropdown.
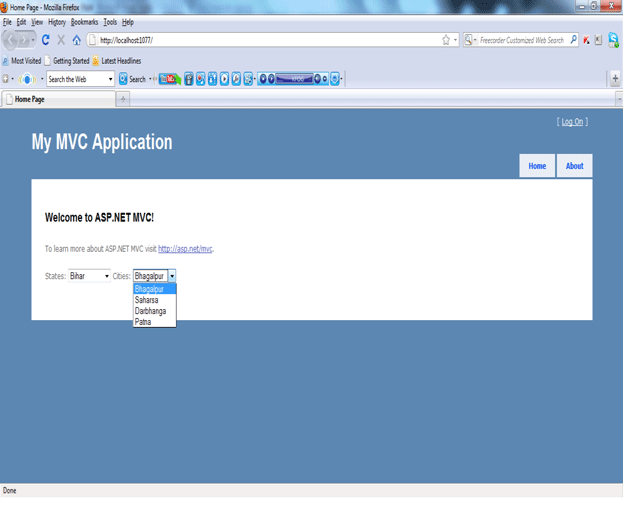
If we Change the value in the State dropdown -> Cities dropdown gets correspondingly populated.
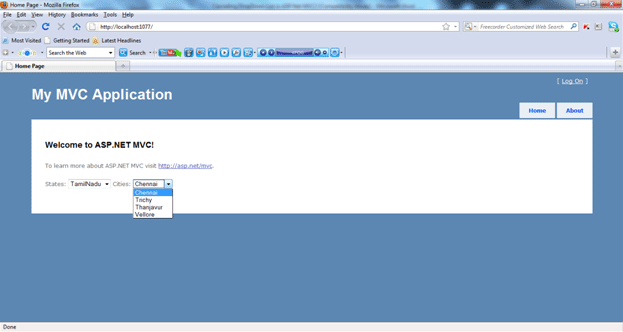
So in this article we have seen how can we implement Cascading DropDownList in ASP.Net MVC using AJAX methos in Jquery by using JSON.
I have attached the code for this sample application.
Happy Learning!!