In this article we are going to see how to create a DataTable and add it to a DataSet.
Example :
using System;
using System.Data;
namespace CreateDataTable
{
classProgram
{
static void Main(string[] args)
{
DataSet objDs = newDataSet();
// Add a DataTable to DataSet directly
DataTable objDt1 = objDs.Tables.Add("Table 1");
// add some columns here
// Creating the table and adding it to the DataSet
DataTable objDt2 = newDataTable("Table 2");
// add some columns here
objDs.Tables.Add(objDt2);
// Add multiple DataTables to the DataSet
DataTable objDt3 = newDataTable("Table 3");
DataTable objDt4 = newDataTable("Table 4");
// add some columns here
objDs.Tables.AddRange(newDataTable[] { objDt3, objDt4 });
Console.WriteLine("DataSet has {0} DataTables \n", objDs.Tables.Count);
foreach (DataTable objDt in objDs.Tables)
Console.WriteLine("{0}", objDt.TableName);
Console.WriteLine("\nPress any key to continue.");
Console.ReadLine();
}
}
}
In this example we are using the Add() and AddRange() methods of the DataTableCollection of the DataSet to add a DataTable to a DataSet. Here are examples of three different techniques to add a DataTable to a DataSet.
-
Add a DataTable directly to a DataSet
-
Create a DataTable and Add it to a DataSet
-
Create multiple DataTable objects and add them to DataSet using the AddRange() method of the DataTableCollection of the DataSet.
The DataTableCollection contains all of the DataTable objects for a particular DataSet. You can access the DataTableCollection of a DataSet using the Tables property.
The key methods of the DataTableCollection are Add(), AddRange(), AddRange(), Clear(), Contains(), CopyTo(), IndexOf(), Remove(), RemoveAt()
Add() method has four overloads:
Output :
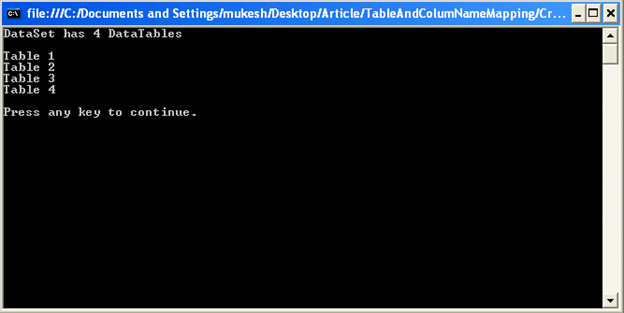