Introduction
Here in this article I am discussing how F# implements inheritance. Some power of Object Oriented Programming comes in the form of inheritance. The ability to base a new type on the structure and function of a previously defined type. Inheritance allows you to extend a class that is already defined (subclassed), as well as to add new functionality or possibly to modify or replace the original functionality.
In Object Oriented Programming, inheritance is a way to compartmentalize and reuse code by creating collections of attributes and behaviors called objects which can be based on previously called objects. Whereas in classical inheritance, objects are defined by classes, classes can inherit other classes. The new classes are known as subclasses or derived classes.
Inheritance is an operation of Type Algebra that creates a new type from one or several parent types. The obtained type is called a derived type. It inherits some of the properties of its parent's type.
Inheritance should not be confused with subtype polymorphism or you can say just polymorphism; inheritance is a relationship between implementations, whereas polymorphism is a relationship between types.
The properties it inherits are as follows:
Subclasses
A subclass is in the simplest term, a class derived from a class which has already been defined. A subclass inherits its members from a base class in addition to its own members. You will define a subclass with an Inherit keyword, which must come directly after the equal sign.
A class can have one direct base class although it can have ancestors. If a class does not inherit from another class explicitly, it inherits implicitly from the .NET ultimate base class, object.
When a derived class inherits from a base class, automatically all the non-private members of the base class are available to the user of the derived class. A derived class uses the "base" keyword to access the members of the base class directly.
In the following we are discussing an example which shows an F# class, that derives from a base class. The sub class has one method as well as a base class has one method. We are showing that a subclass can use both the methods because it inherits from the base class.
Example
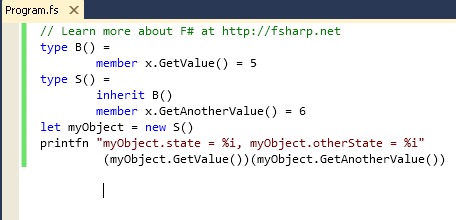
type B() =
member x.GetValue() = 5
type S() =
inherit B()
member x.GetAnotherValue() = 6
let myObject = new S()
printfn "myObject.state = %i, myObject.otherState = %i"
(myObject.GetValue())(myObject.GetAnotherValue())
Output
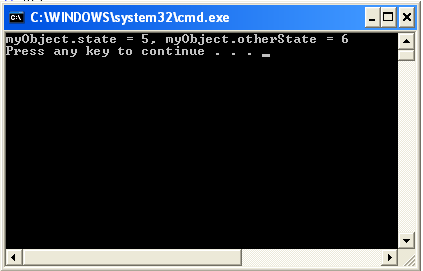
Overrides
Overrides means when you want a derived class to change default behavior of methods inherited from the base class or you can say that Overrides permits a class or objects to replace the implementation of an aspect; typically a behavior that it inherits. If a derived class implements the member functions with the same name, number and type of parameters as the base class, then the derived class hides the base's implementation.
Example
type Name(name: string) =
member this.Create() = printfn "Dea Saddler"
member this.Close() = ()
type MyEncryptedName(ename: string) =
inherit Name(ename)
// hides base implementation
member this.Create() = printfn "MyEncryptedName created"
Methods
A derived class can define new methods, overrides methods derived from its base class. Methods can be defined by one of four keywords:
-
Member
-
Overrides
-
Abstract
-
Default
The Member keyword defines a simple method which can't be overridden with an implementation.
The Abstract keywords define a method with no implementation that should be overridden in a derived class.
The Override keyword defines a method that overrides an inherited method that has an implementation in a base class.
Default is the same as Override except it is used to override an abstract method.
Example
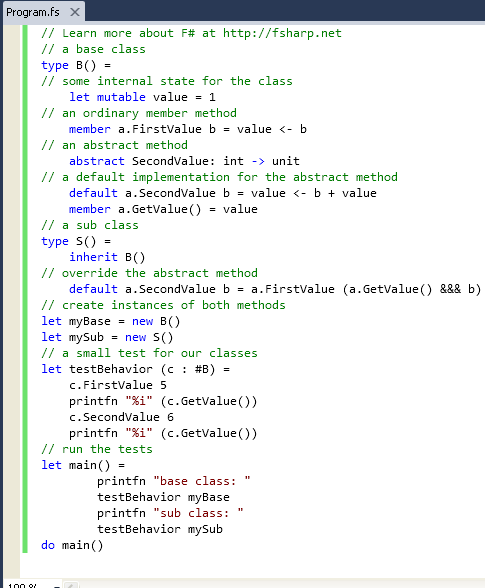
// a base class
type B() =
// some internal state for the class
let mutable value = 1
// an ordinary member method
member a.FirstValue b = value <- b
// an abstract method
abstract SecondValue: int -> unit
// a default implementation for the abstract method
default a.SecondValue b = value <- b + value
member a.GetValue() = value
// a sub class
type S() =
inherit B()
// override the abstract method
default a.SecondValue b = a.FirstValue (a.GetValue() &&& b)
// create instances of both methods
let myBase = new B()
let mySub = new S()
// a small test for our classes
let testBehavior (c : #B) =
c.FirstValue 5
printfn "%i" (c.GetValue())
c.SecondValue 6
printfn "%i" (c.GetValue())
// run the tests
let main() =
printfn "base class: "
testBehavior myBase
printfn "sub class: "
testBehavior mySub
do main()
Output
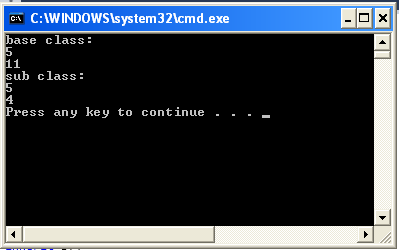
Summary
In this article I have discussed about inheritance in F#.