Introduction
This is another short article on the date time picker control. This article will address an approach that may be used to require the user to confirm changing a date. Using this approach, the application shall display a confirmation dialog each time the user changes the date value associated with a date time picker control.
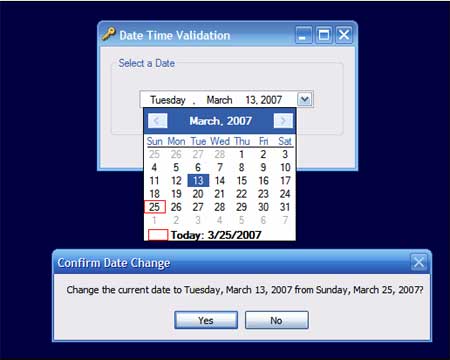
Figure 1: Confirming an Update to the Date Time Picker Control's Value.
The date time picker contains a validation event which may be handled in code; the problem with validating the control using its validation event is that, if the validation includes confirmation of the change, the validation event will fire twice. The first time will occur when the value is changed, the second time will occur if the user denies the confirmation and the control's value is swapped back to the previous value. To that end, if one is using a message box control to capture the confirmation, the users will be treated to viewing that confirmation twice in a row.
Getting Started
The solution contains only a single project and that project contains only a single form. On the form is a single date time picker. All code necessary to perform the confirmation is contained in the single form class.
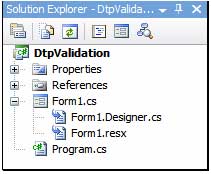
Figure 2: Solution Explorer.
Code: Form1
In order to confirm the selection of a new date value, the user is presented with the display of a confirmation message box. The confirmation box shall display the previous and current date values and ask the user to confirm which date time value to retain. The form class begins with the usual imports, namespace declaration, and class constructor.
using System;
using System.Collections.Generic;
using System.ComponentModel;
using System.Data;
using System.Drawing;
using System.Text;
using System.Windows.Forms;
namespace DtpValidation
{
public partial class Form1 : Form
{
/// <summary>
/// Local member variables used to
/// keep track of the previous change; this
/// is necessary to allow the user to
/// rollback the date change if they decide
/// to left the date in its previous state
/// </summary>
DateTime mPreviousTime;
/// <summary>
/// Default constructor
/// </summary>
public Form1()
{
InitializeComponent();
mPreviousTime = DateTime.Now;
}
After the namespace, a single local member variable is declared. This variable will be used to capture and retain the previous time. If the user refuses to accept an initiated value change, the current value of the date time picker shall be repopulated with the previous date as stored in this variable.
The next bit of code to look at in this form is the date time picker's CloseUp event handler; it is in this handler and not in the control's validation event handler that we actually do the validation; by making the check here we may perform the validation and possibly the rollback to the prior value without issuing the duplicated confirmation message. In this code, the confirmation box is display; if the user selects "Yes", the previous time value is set to contain the value contained in the date time picker; if the user selects "No", the date time picker value is returned to previous value using the copy contained in the previous time variable.
/// <summary>
/// Validate the value of the data time picker control
/// from its CloseUp event handler
/// </summary>
/// <param name="sender"></param>
/// <param name="e"></param>
private void dateTimePicker1_CloseUp(object sender, EventArgs e)
{
DialogResult dr = MessageBox.Show("Change the current date to " +
dateTimePicker1.Value.ToLongDateString() + " from " +
mPreviousTime.ToLongDateString() + "?",
"Confirm Date Change", MessageBoxButtons.YesNo);
if (dr == DialogResult.Yes)
{
mPreviousTime = dateTimePicker1.Value;
}
else
{
dateTimePicker1.Value = mPreviousTime;
}
}
Summary.
This article was intended to demonstrate an approach to allowing the developer to confirm setting changeds to the value contained within an instance of the date time picker control. If the developer opts to perform the confirmation task in the date time picker's value changed event handler, the user will presented with two confirmation boxes each time the value is changed and then restored to its previous value.