Question:
a) How to display single record per page and navigate through single record using ASP.NET.
Solution:
There are various ways this can be accomplished. One of the ways is described in article which uses ViewState. We'll see how this can be done using a Datagrid and its Paging functionality.
We'll discuss two methods by which this can be achieved.
1. Navigating single record using the default Paging style of DataGrid (In this sample we use mode as numeric).
2. Navigating single record using the conventional ASP way by having "First", "Next", "Previous", "Last" buttons.
Method 1: Navigating single record using the default Paging style of DataGrid
Step 1: UI
Lets drag and drop the Datagrid
<
p align ="center">
<asp:DataGrid id="DataGrid1" runat="server" BorderColor="#E7E7FF"
AllowPaging="True" OnPageIndexChanged="PageRecords" PageSize=<%#intPageSize%>
BorderWidth="1px" BackColor="White" CellPadding="3" GridLines="Horizontal" BorderStyle="None" Font-Names="Verdana" Font-Size="X-Small" Width="50%" ItemStyle-Width="25%">
<SelectedItemStyle Font-Bold="True" ForeColor="#F7F7F7" BackColor="#738A9C"></SelectedItemStyle>
<AlternatingItemStyle BackColor="#F7F7F7"></AlternatingItemStyle>
<ItemStyle ForeColor="#4A3C8C" BackColor="#E7E7FF"></ItemStyle>
<HeaderStyle Font-Bold="True" ForeColor="#F7F7F7" BackColor="#4A3C8C"></HeaderStyle>
<FooterStyle ForeColor="#4A3C8C" BackColor="#B5C7DE"></FooterStyle>
<PagerStyle Visible="true" HorizontalAlign="Right" ForeColor="#4A3C8C" BackColor="#E7E7FF" Mode="NumericPages"></PagerStyle>
</asp:DataGrid>
</p>
Short Description of what we have done:
a) Set property AllowPaging=True for DataGrid.
b) For Paging we write relevant code in the event PageIndexChanged.
c) In normal scenario for paging the PageSize is set to as many records that we wish to display on the page which imply the number of rows to be displayed per page. In the current sample code we are trying to display the records for a single row on a single page. So in this case we'll set the PageSize to the number of the columns in the DataSet
d) We set property Visible = true for PagerStyle with a numeric mode.
Step 2: Code
//Method 1-> Part a.
SqlConnection mycn;
SqlDataAdapter myda;
DataSet ds;
String strConn;
protected int intPageSize;
private void Page_Load(object sender, System.EventArgs e)
{
// Put user code to initialize the page here
strConn="Data Source=localhost;uid=sa;pwd=;Initial Catalog=pubs";
mycn = new SqlConnection(strConn);
myda = new SqlDataAdapter ("Select * FROM titles", mycn);
ds= new DataSet ();
myda.Fill (ds,"Table");
//The data is displayed as
//Column1 -> ColumnNames
//Column2 -> Column Data
//Hence the intPageSize is set to number of Columns to be Displayed on page
intPageSize = ds.Tables [0].Columns.Count;
//GoDoReshape(ds)-> Function: To display the data vertically
DataGrid1.DataSource=GoDoReShape(ds);
if (!Page.IsPostBack )
{
DataGrid1.DataBind ();
}
}
public DataSet GoDoReShape(DataSet ds)
{
DataSet NewDs=new DataSet();
NewDs.Tables.Add();
//Create Two Columns with names "ColumnName" and "Value"
//ColumnName -> Displays all ColumnNames
//Value -> Displays ColumnData
NewDs.Tables[0].Columns.Add("ColumnName");
NewDs.Tables[0].Columns.Add("Value");
foreach(DataRow dr in ds.Tables [0].Rows )
{
foreach(System.Data.DataColumn dcol in ds.Tables[0].Columns)
{
//Declare Array
string[] MyArray={dcol.ColumnName.ToString(),dr[dcol.ColumnName.ToString()].ToString()};
NewDs.Tables[0].Rows.Add(MyArray);
}
}
return NewDs;
}
Code for Paging
//Method 1-> Part b.
protected void PageRecords(object source, System.Web.UI.WebControls.DataGridPageChangedEventArgs e)
{
DataGrid1.CurrentPageIndex = e.NewPageIndex;
DataGrid1.DataBind();
}
The Output for this is shown below (After Method 2 Description)
Method 2 : Navigating single record using the conventional ASP way by having "First", "Next", "Previous", "Last" buttons.
Step 1: UI. Moving to next method i.e. ASP way add 4 buttons
<
p align="center">
<asp:DataGrid id="DataGrid1" AllowPaging="True" PageSize=<%#intPageSize%> PagerStyle-Visible="False" runat="server" BorderColor="#E7E7FF" BorderWidth="1px" BackColor="White" CellPadding="3" GridLines="Horizontal" BorderStyle="None" Font-Names="Verdana" Font-Size="X-Small" Width="50%" ItemStyle-Width="25%">
<SelectedItemStyle Font-Bold="True" ForeColor="#F7F7F7" BackColor="#738A9C"></SelectedItemStyle>
<AlternatingItemStyle BackColor="#F7F7F7"></AlternatingItemStyle>
<ItemStyle ForeColor="#4A3C8C" BackColor="#E7E7FF"></ItemStyle>
<HeaderStyle Font-Bold="True" ForeColor="#F7F7F7" BackColor="#4A3C8C"></HeaderStyle>
<FooterStyle ForeColor="#4A3C8C" BackColor="#B5C7DE"></FooterStyle>
</asp:DataGrid>
<br>
<asp:Button id="Firstbutton" Text="First" CommandName="First" runat="server" onClick="PagerButtonClick" />
<asp:Button id="Prevbutton" Text="Prev" CommandName="prev" runat="server" onClick="PagerButtonClick" />
<asp:Button id="Nextbutton" Text="Next" CommandName="next" runat="server" onClick="PagerButtonClick" />
<asp:Button id="Lastbutton" Text="Last" CommandName="last" runat="server" onClick="PagerButtonClick" />
<br>
The DataGrid tag part is as UI given in Method 1 except for:
a) The PagerStyle-Visible Property of datagrid is set to false.
As far as coding for binding the DataGrid, its same as Step 2->Method 1-> Part a->given above.
We'll move to the Navigational Part
We have 4 buttons First, Next... and for handling the click Events of this button code is as follows:
Step 2: Code
//Method 2.
protected void PagerButtonClick(object sender, System.EventArgs e){
string btnCommandName = ((Button)sender).CommandName;
switch (btnCommandName.ToUpper())
{
case "FIRST" :
DataGrid1.CurrentPageIndex = 0;
break;
case "PREV" :
DataGrid1.CurrentPageIndex = Math.Max(DataGrid1.CurrentPageIndex -1, 0);
break;
case "NEXT" :
DataGrid1.CurrentPageIndex = Math.Min(DataGrid1.CurrentPageIndex + 1, DataGrid1.PageCount - 1);
break;
case "LAST" :
DataGrid1.CurrentPageIndex = DataGrid1.PageCount - 1;
break;
default :
break;
}
DataGrid1.DataBind();
}
Method 1 Output: Method 2 Output:
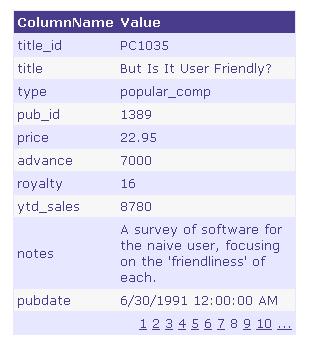
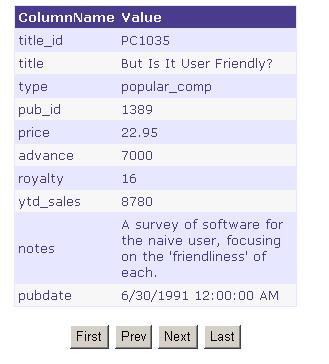