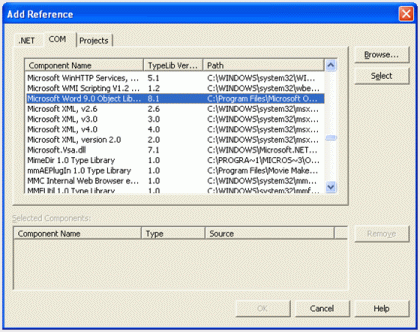
namespace ScreenCapture
{
/// <summary>
/// Summary description for Form1.
/// </summary>
public class Form1 : System.Windows.Forms.Form
{
private System.Windows.Forms.Button button1;
/// <summary>
/// Required designer variable.
/// </summary>
private System.ComponentModel.Container components = null;
public Form1()
{
//
// Required for Windows Form Designer support
//
InitializeComponent();
//
// TODO: Add any constructor code after InitializeComponent call
//
}
/// <summary>
/// Clean up any resources being used.
/// </summary>
protected override void Dispose( bool disposing )
{
if( disposing )
{
if (components != null)
{
components.Dispose();
}
}
base.Dispose( disposing );
}
#region Windows Form Designer generated code
/// <summary>
/// Required method for Designer support - do not modify
/// the contents of this method with the code editor.
/// </summary>
private void InitializeComponent()
{
this.button1 = new System.Windows.Forms.Button();
this.SuspendLayout();
//
// button1
//
this.button1.BackColor = System.Drawing.Color.CornflowerBlue;
this.button1.Font = new System.Drawing.Font("Microsoft Sans Serif", 10F,
System.Drawing.FontStyle.Bold, System.Drawing.GraphicsUnit.Point, ((System.Byte)(0)));
this.button1.ForeColor = System.Drawing.Color.Cornsilk;
this.button1.Location = new System.Drawing.Point(0, 0);
this.button1.Name = "button1";
this.button1.Size = new System.Drawing.Size(120, 23);
this.button1.TabIndex = 1;
this.button1.Text = "Capture";
this.button1.Click += new System.EventHandler(this.button1_Click);
//
// Form1
//
this.AutoScaleBaseSize = new System.Drawing.Size(5, 13);
this.ClientSize = new System.Drawing.Size(115, 22);
this.ControlBox = false;
this.Controls.Add(this.button1);
this.Name = "Form1";
this.Load += new System.EventHandler(this.Form1_Load);
this.ResumeLayout(false);
}
#endregion
/// <summary>
/// The main entry point for the application.
/// </summary>
[STAThread]
static void Main()
{
Application.Run(new Form1());
}
private void Form1_Load(object sender, System.EventArgs e)
{
}
private void CaptureDesk()
{
MethodsForCapture objMethods = new MethodsForCapture();
//First we will capture the image of desktop
objMethods.CaptureScreen(@"c:\TestCapture.jpg",ImageFormat.Jpeg);
MessageBox.Show("Captured");
FileStream f = new FileStream(@"c:\TestCapture.jpg",FileMode.Open);
//For copying the captured image we have to create a object of //BMP class and copy
that object to clipboard
Bitmap b = new Bitmap(f,true);
Clipboard.SetDataObject(b,true);
//Now we will create a word application which can host our //
//word document
Word.Application app = new Word.ApplicationClass();
//I assume that this file is already present at current location. You can replace this code
with simple file creation code which will check whether this file exists or not and if not it
will create that file
object file = @"c:\ScreenDoc.doc";
//Use Reflection to identify the missing values
object miss = System.Reflection.Missing.Value;
object otrue = true;
object ofalse = false;
try
{
// If you are using Microsoft word 11.0 library then use commented code
/* Word.Document doc = app.Documents.Open(ref file,ref miss,ref ofalse,ref
miss,ref miss,ref miss,ref miss,ref miss,ref miss,ref miss,ref miss,ref otrue, ref
miss,ref miss,ref miss,ref miss); */
// If you are using Microsoft word 9.0 library then use following code
Word.Document doc = app.Documents.Open(ref file,ref miss,ref ofalse,ref miss, ref
miss,ref miss,ref miss,ref miss,ref miss,ref miss,ref miss,ref otrue);
//now we specify the start and stop position of the image. We can also place
bookmark and can place an image at that point
object start = 0;
object end = 0;
Word.Range rng = doc.Range(ref start,ref end);
//Now we paste the image which we have on clipboard
rng.Paste();
//Saving the document
doc.Save();
app.Quit(ref otrue,ref miss,ref miss);
MessageBox.Show("Image saved in a document");
}
catch(Exception ex)
{
MessageBox.Show("" + ex.Message);
}
}
private void button1_Click(object sender, System.EventArgs e)
{
CaptureDesk();
}
}
//Now we will write a class which includes GDI32.dll methods
public class GDI32
{
[DllImport("GDI32.dll")]
public static extern bool BitBlt(int hdcDest,int nXDest,int nYDest,
int nWidth,int nHeight,int hdcSrc,
int nXSrc,int nYSrc,int dwRop);
[DllImport("GDI32.dll")]
public static extern int CreateCompatibleBitmap(int hdc,int nWidth,
int nHeight);
[DllImport("GDI32.dll")]
public static extern int CreateCompatibleDC(int hdc);
[DllImport("GDI32.dll")]
public static extern bool DeleteDC(int hdc);
[DllImport("GDI32.dll")]
public static extern bool DeleteObject(int hObject);
[DllImport("GDI32.dll")]
public static extern int GetDeviceCaps(int hdc,int nIndex);
[DllImport("GDI32.dll")]
public static extern int SelectObject(int hdc,int hgdiobj);
}
public class User32
{
[DllImport("User32.dll")]
public static extern int GetDesktopWindow();
[DllImport("User32.dll")]
public static extern int GetWindowDC(int hWnd);
[DllImport("User32.dll")]
public static extern int ReleaseDC(int hWnd,int hDC);
}
public class MethodsForCapture
{
public void CaptureScreen(string fileName,ImageFormat imageFormat)
{
//First we get a desktop window using user32.dll API and then find its //handle
int hdcSrc = User32.GetWindowDC(User32.GetDesktopWindow()),
hdcDest = GDI32.CreateCompatibleDC(hdcSrc),
//Create a BMP
hBitmap = GDI32.CreateCompatibleBitmap(hdcSrc,
GDI32.GetDeviceCaps(hdcSrc,8),GDI32.GetDeviceCaps(hdcSrc,10));
GDI32.SelectObject(hdcDest,hBitmap);
GDI32.BitBlt(hdcDest,0,0,GDI32.GetDeviceCaps(hdcSrc,8),
GDI32.GetDeviceCaps(hdcSrc,10),
hdcSrc,0,0,0x00CC0020);
SaveImageAs(hBitmap,fileName,imageFormat);
Cleanup(hBitmap,hdcSrc,hdcDest);
}
private void Cleanup(int hBitmap,int hdcSrc,int hdcDest)
{
User32.ReleaseDC(User32.GetDesktopWindow(),hdcSrc);
GDI32.DeleteDC(hdcDest);
GDI32.DeleteObject(hBitmap);
}
private void SaveImageAs(int hBitmap,string fileName,ImageFormat imageFormat)
{
Bitmap image =
new Bitmap(Image.FromHbitmap(new IntPtr(hBitmap)),
Image.FromHbitmap(new IntPtr(hBitmap)).Width,
Image.FromHbitmap(new IntPtr(hBitmap)).Height);
image.Save(fileName,imageFormat);
}
}
}