I have encountered instances when I needed a high level look and feel of Windows Forms and controls. Of course, I can set property value in each form at design time. But this one-time change is inflexible and impractical when I have to manage hundreds of Window Forms. For example, in multi-lingual capable application, Windows From font is likely to change accordingly to user environment. And the application may not choose the same font as the Windows default setting. To remedy this issue, this obviously requires programmatically changes.
The key to manage this requirement is to enforce look of feel at runtime. My solution is to use helper class and codify look and feel. All Window Forms will make one simple method call to standardize look and feel. This framework not only alleviates developers from mundane change at design time, it also prevents accidental modification without notice.
Here're the steps I take:
- Define your look and feel class.
I create a static class called as UniformLookAndFeel. I codify my look and feel in static method Format.
///
/// This static class enforces look and feel on all Windows Forms.
///
public class UniformLookAndFeel
{
static UniformLookAndFeel() {}
///
/// Define default ui look and feel setting.
///
/// Windows From to be formatted
public static void Format(System.Windows.Forms.Form form)
{
System.Windows.Forms.Control.ControlCollection ctrlCol = form.Controls;
System.Collections.IEnumerator ienum = ctrlCol.GetEnumerator();
ienum.Reset();
while(ienum.MoveNext())
{
Object obj = ienum.Current;
string typeStr = obj.GetType().ToString();
// Define labels look and feel
if(typeStr=="System.Windows.Forms.Label")
{
// Define font
Label label = (Label)obj;
label.Font = new Font("Arial", 9, System.Drawing.FontStyle.Bold);
// Set warning label text color as red
if(label.Name=="lblWarning")
label.ForeColor = System.Drawing.Color.Red;
}
// Define textboxes look and feel
if(typeStr=="System.Windows.Forms.TextBox")
{
// Define font
TextBox textBox = (TextBox)obj;
textBox.Font = new Font("Times New Roman", 9);
}
}
}
}
This method iterates the input form and defines properties on appropriate control. In my case, I want all labels to use bolded Arial font with size 9; for warning label, the font color should be red; for text control, use Times New Roman with size 9.
- After creating my own calss, I invoke the method at right place.
public MyWinForm()
{
// This call is required by the Windows Form Designer.
InitializeComponent();
// Unify look and feel
UniformLookAndFeel.Format(this);
}
I invoke the call in my Windows Form constructor. To trigger look and feel formatting, let InitializeComponent method complete the initialization. Afterward the Windows Form call UniformLookAndFeel.Format(System.Windows.Forms.Form).
The change is completed. As, you can see, you only put minimal effort to make a huge difference on Windows Forms.
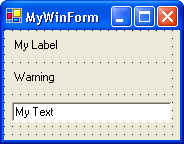
Windows Form at design time.
Notice I did not modify any font properties on labels and textboxes.
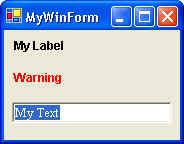
Windows Form at runtime.
Change is made programatically. Thus, uniform look and feel is accomplished.
To keep my explanation simple, I only focus on font management. But the limit doesn't end there. You may also standardize look and feel on buttons, status bar and other Windows controls. As you can see, this framework can handle many situations to comply with your own look and feel standard.