Web Services
Web Services means structuring operations and data according to SOAP specification in an XML document. SOAP (Simple Object Access Protocol) is a way for a program running in one kind of operating system or language (e.g. Windows or .Net) to communicate with a program in the same or another kind of an operating system or language (e.g. Linux or Java) by using the HTTP and XML as the mechanisms for information exchange. Since Web protocols are installed and available for use by all major operating system platforms, HTTP and XML provide an already at-hand solution to the problem of how programs running under different operating systems in a network can communicate with each other. SOAP specifies exactly how to encode an HTTP header and an XML file so that a program in one computer can call a program in another computer and pass it information. It also specifies how the called program can return a response. Once a Web Service is implemented, a client sends a message to the component as an XML document and the component sends an XML document back to the client as the response.
Interoperability
The goal of this article is to show to integrate Microsoft's .NET Platform Web service with other platform like Java and ASP. The samples demonstrate basic techniques and principles that are used to cross-platform interoperability via Web services. You start off by writing code for your Web service. After you compile, the Web services framework uses your code to dynamically generate a WSDL file. When clients request your Web service definition, they retrieve the generated WSDL and then create the client proxy based on that definition.
The example here contains a web services in .Net C#, which returns the data from Customer table of Northwind database SQL Server in XML format. Data is retrieved through Dataset and then converted to XML. To display the data in the client side browser it is accessed by .Net, Java and classic ASP.
Through .Net there are two ways to display data in browser from web service. First it can be convert back to Dataset using XMLDataDocument and Schema. And Second one by using XMLDocument and style sheet.
In Java client Apache client proxy is used to get the data from .Net web services and then XML Document Builder and XML Document is used to display the data in browser with the help of XSL Transformer.
Classic ASP use Microsoft SOAP Toolkit 3.0 to get data from web services and MSXML 4.0 to load and display the data in browser.
.Net C# Web service
Visual Studio automatically creates the necessary files and includes the needed references to support an XML Web service, to create a web services in C# in Visual Studio.NET.
1. On the File menu. File->New->Project.
2. In the New Project dialog box, select either the visual C# Projects folder. Select the ASP.NET
Web Service icon.
3. Enter the address of the Web server on which you will develop the XML Web service and specify TestWebService as the directory name, such as "http://localhost/TestWebService". Click OK to create the project.
4. When you create an XML Web service, Service1.asmx will be created automatically. Change the name of Service1.asmx to ThakurService.asmx.
5. Specify the properties to WebService attribute. The namespace property you specify is used within the WSDL document of the Web service to uniquely identify the callable entry points of the service. Name is service name to display in help page. Description property of this attribute is included in the Service help page to describe the services usage. As shown in example here:
[WebService (Namespace = "http://ThakurService", Name = "ThakurService", Description = "Test Service By Anand Thakur")]
//CODE ThakurService.asmx
[WebService ( Namespace = "http://ThakurService", Name = "ThakurService", Description = "Test Service By Anand Thakur" )]
public class ThakurService : System.Web.Services.WebService
{
public ThakurService()
{
InitializeComponent();
}
[WebMethod(MessageName = "GetCustomerData",Description = "Return DataSet XML of Contact Name and Company Name from Northwind.Customers table" )]
public String GetCustomerData()
{
///data source string
string source = "Data Source=ATHAKUR;Initial Catalog=Northwind;user=sa;password=cincom" ;
// And this is the SQL statement that will be issued
string select = "SELECT Top 20 ContactName,CompanyName FROM Customers";
SqlConnection conn=new SqlConnection(source);
// Open the database connection
conn.Open () ;
/// Create the SQL command...
SqlCommand cmd = new SqlCommand ( select , conn ) ;
conn.Close ( ) ;
SqlDataAdapter myDataAdapter = new SqlDataAdapter(cmd);
///declare myDataSet as DataSet
DataSet myDataSet= new DataSet("AnandThakur");
///fill the dataset with returned records
myDataAdapter.Fill(myDataSet, "Products");
///finally return dataset
return myDataSet.GetXml();
}
}
6. Add one public method GetCustomerData with return type string. Add WebMethod attribute to this method. The WebMethod attribute instructs .NET to add the code necessary to marshal calls using XML and SOAP. The MessageName property of the WebMethod attribute enables the XML Web service to uniquely identify overloaded methods using an alias. Unless otherwise specified, the default value is the method name. The Description property of the WebMethod attribute supplies a description for an XML Web service method that will appear on the Service help page
7. Add the necessary code to retrieve the data from Northwind.Customers table. From dataset return XML using GetXML method as shown in code.
8. Run the web service. You will see the following output shown in figure. You can see the service as well as web method name and description in output and link to service WSDL. You can further explore the web method to see method SOAP and output.
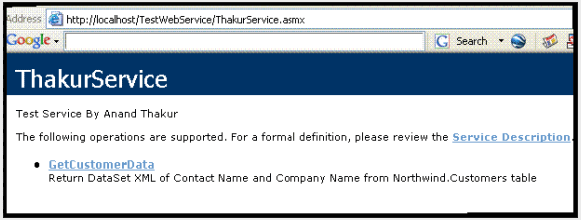
9. Now our web service is complete and ready to serve clients. We will consume the service from Java, .net and classic ASP client.
Note: if you want to browse web service directly through browser without VS.net. Then add following entries in web.config within <system.web>.
<webServices>
<protocols>
<add name="HttpSoap"/>
<add name="HttpPost"/>
<add name="HttpGet"/>
</protocols>
</webServices>
.Net Client
To test the above web services with .net client.
1. Open VS.net. On the File menu, click New->Project.
2. The New Project dialog box appears.
3. In the Project Type pane, click Visual C# Projects, and select ASP.NET Web Application.
4. Name your application by changing the default name in the Location box (such as http://localhost/times as in example) Click OK.
5. WebForm1.aspx and WebForm1.aspx.cs will be created automatically.WebForm1.aspx - Contains the visual representation of the Web Form and WebForm1.aspx.cs - The code-behind file that contains the code for event handling.
6. In the solution explorer, right click on project and select Add Web Reference from context menu. Add Web Reference window will be opened to enter the web service URL. Enter the URL to web service (http://localhost/TestWebService/ThakurService.asmx). Enter the web reference as localhost and click on Add Reference button. Now web references added to the project tree in solution explorer. You can see WSDL, DISCO and .cs proxy class for web services.
7. Now we ready to consume the service. Here we are consuming our service by two ways. One through dataset and another one using XMLDocument.
8. To consume the service through dataset. Add dataset schema to client web application. Right click project in solution explorer and select Add->Add New Item->XML Schema. Enter the file name as Products.xsd. And paste the code below in the file.
//CODE Products.xsd
<xs:schema id="AnandThakur" xmlns="" xmlns:xs="http://www.w3.org/2001/XMLSchema" xmlns:msdata="urn:schemas-microsoft-com:xml-msdata">
<xs:element name="AnandThakur" msdata:IsDataSet="true">
<xs:complexType>
<xs:choice maxOccurs="unbounded">
<xs:element name="Products">
<xs:complexType>
<xs:sequence>
<xs:element name="ContactName" type="xs:string" minOccurs="0" />
<xs:element name="CompanyName" type="xs:string" minOccurs="0" />
</xs:sequence>
</xs:complexType>
</xs:element>
</xs:choice>
</xs:complexType>
</xs:element>
</xs:schema>
9. Now open the WebForm1.aspx.cs file and add following code in Page Load Event.
//Code WebForm1.aspx.cs
private void Page_Load(
object sender, System.EventArgs e)
{
//web service proxy object
times.localhost.ThakurService ts1 =
new times.localhost.ThakurService();
//Call web method
string strHelloThakur = ts1.GetCustomerData();
XmlDataDocument xm=
new XmlDataDocument();
StreamReader myStreamReader =
null;
myStreamReader =
new treamReader(Server.MapPath("Products.xsd"));
xm.DataSet.ReadXmlSchema(myStreamReader);
//Laod XML return from web method
xm.LoadXml(strHelloThakur);
Response.Write("<Table bgcolor='ffffff' cellpadding='1' width='640' border='1'>");
Response.Write("<TR>");
Response.Write("<TD><B>Contact Name</B></TD>");
Response.Write("<TD><B>Company Name</B></TD>");
Response.Write("</TR>");
//get the data into dataset and dispay in asp.net page
DataSet ds = xm.DataSet;
for(
int i=0;i<ds.Tables["Products"].Rows.Count;i++)
{
Response.Write("<TR>");
Response.Write("<TD> "+ ds.Tables[0].Rows[i][0].ToString()+ "</TD>");
Response.Write("<TD> "+ ds.Tables[0].Rows[i][1].ToString()+ "</TD>");
Response.Write("</TR>");
}
Response.Write("</Table>");
}
10. Now right click on WebForm1.aspx and select Set as Start Page. And run the application. If everything works fine, you will see the following output in browser.
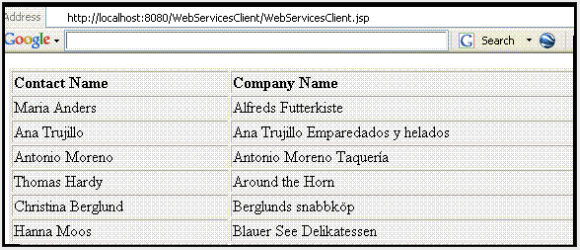
11. To get same output through XMLDocument. Add one more Web Form (WebForm2.aspx)
12. Right click project in solution explorer and select Add->Add New Item->XSLT file. Name the file as Products.xsl. And paste the following code in .xsl file.
//CODE Products.xsl
<xsl:stylesheet xmlns:xsl="http://www.w3.org/1999/XSL/Transform" version="1.0">
<xsl:template match="/">
<xsl:apply-templates />
</xsl:template>
<xsl:template match="AnandThakur">
<table bgcolor="eeefff" cellpadding="1" width="640" border="1">
<tr>
<td><B>Contact Name
</B></td>
<td><B>Company Name
</B></td>
</tr>
<xsl:apply-templates select="Products"/>
</table>
</xsl:template>
<xsl:template match="Products">
<tr>
<td> <xsl:value-of select="ContactName" /></td>
<td> <xsl:value-of select="CompanyName" /></td>
</tr>
</xsl:template>
</xsl:stylesheet>
13. Now open the WebForm2.aspx.cs file and add following code in Page Load Event. Set WebForm2.aspx as start up page and run the application. You will see the same output as in dataset case.
//CODE WebForm2.aspx.cs
private void Page_Load(
object sender, System.EventArgs e)
{
times.localhost.ThakurService ts1 =
new times.localhost.ThakurService();
string str = ts1.GetCustomerData();
XmlDocument xmlDoc =
new XmlDocument();
xmlDoc.LoadXml(str);
XslTransform XMLTrans =
new XslTransform();
XMLTrans.Load(Server.MapPath("Products.xsl"));
XMLTrans.Transform(xmlDoc,
null, Response.OutputStream,
null );
}
Java/JSP Client
Eclipse and TOMCAT is used in this example to make a JSP client with the help of Apache SOAP toolkit (http://ws.apache.org/soap). You can get the TOMCAT plug-in for Eclipse from http://www.sysdeo.com/eclipse/tomcatPlugin.html. Assumption here is that you are bit familiar with JSP and Eclipse.
1. Click on File->New ->project ->Tomcat Project.
2. A web project will be created. Right click on project and select properties. In property window select Java Build Path and click on Libraries. Click on External Files Button and add soap.jar, activation.jar, mailapi.jar and xerces.jar reference to project. You can download these jar file from Apache/Sun site.
3. In order to create SAOP proxy, right click on project in Project Explorer and select New->Package. Name the package as WebServicesClient. New package will be created. Now add new class named MsgBody.java in this package. And paste the following code in class file.
//CODE MsgBody.Java
package WebServicesClient;
import java.io.*;
import org.apache.soap.*;
import org.apache.soap.util.*;
import org.apache.soap.util.xml.*;
import org.apache.soap.rpc.SOAPContext;
public class MsgBody extends Body
{
public void marshall (String strEncodeStyle,Writer msgSink,NSStack nameSpaceStack,
XMLJavaMappingRegistry registry,SOAPContext context)
throws IllegalArgumentException, IOException
{
// Start Element
msgSink.write ('<'+"SOAP-ENV"+':'+ Constants.ELEM_BODY+'>'+ StringUtils.lineSeparator);
// End Element
msgSink.write ("</" + "SOAP-ENV" + ':'+ Constants.ELEM_BODY+'>'+ StringUtils.lineSeparator);
nameSpaceStack.popScope ();
}
}
4. Add one more class in package named WebServiceProxy.java with following code
in it. As you can see in this code URL set to web service http://localhost/TestWebService/ThakurService.asmx. And SOAP URI set to http://ThakurService/GetCustomerData. SOAP URI contains the Namespace of web service we have set in web service plus web method MessageName.
//CODE WebServiceProxy.java
package WebServicesClient;
import java.net.*;
import org.apache.soap.*;
import org.apache.soap.messaging.*;
import javax.activation.*;
public class WebServiceProxy
{
private URL m_url = null;
private String m_soapUri = "";
private Message m_strMsg_ = new Message ();
private Envelope m_envelope = new Envelope ();
private DataHandler m_strReturnMsg = null;
public WebServiceProxy () throws MalformedURLException
{
this.m_url = new URL ("http://localhost/TestWebService/ThakurService.asmx");
}
public synchronized void setWebServiceURL (URL url)
{
this.m_url = url;
}
public synchronized URL getWebServiceURL ()
{
return this.m_url;
}
public synchronized String GetCustomerData () throws SOAPException {
String strReturn = "";
if (this.m_url == null)
{
throw new SOAPException (Constants.FAULT_CODE_CLIENT,"A URL not specified");
}
this.m_soapUri = "http://ThakurService/GetCustomerData";
MsgBody ourBody = new MsgBody ();
this.m_envelope.setBody (ourBody);
m_strMsg_.send (this.getWebServiceURL(), this.m_soapUri, this.m_envelope);
try
{
this.m_strReturnMsg = this.m_strMsg_.receive();
strReturn=this.m_strReturnMsg.getContent().toString();
}
catch (Exception exception)
{
exception.printStackTrace ();
}
return strReturn;
}
}
5. Now in the project add file customers.xsl and paste following code on that.
//CODE customers.xsl
<xsl:stylesheet xmlns:xsl="http://www.w3.org/1999/XSL/Transform" version="1.0">
<xsl:template match="/">
<xsl:apply-templates />
</xsl:template>
<xsl:template match="AnandThakur">
<table bgcolor="efefef" cellpadding="1" width="640" border="1">
<tr>
<td><b>Contact Name
</b></td>
<td><b>Company Name
</b></td>
</tr>
<xsl:apply-templates select="Products"/>
</table>
</xsl:template>
<xsl:template match="Products">
<tr>
<td> <xsl:value-of select="ContactName" /></td>
<td> <xsl:value-of select="CompanyName" /></td>
</tr>
</xsl:template>
</xsl:stylesheet>
6. Project explorer, right click project->new->File. Add new JSP file WebServicesClient.jsp. and paste following code .
//CODE WebServicesClient.jsp
<%@ page language= "java"%>
<%@ page import="java.io.*" %>
<%@ page import="WebServicesClient.*" %>
<%@ page import="org.w3c.dom.*,javax.xml.parsers.*" %>
<%@ page import="javax.xml.transform.*" %>
<%@ page import="javax.xml.transform.stream.*"%>
<%
try
{
// Parsing the XML
DocumentBuilderFactory dbf=DocumentBuilderFactory.newInstance();
DocumentBuilder db=dbf.newDocumentBuilder();
Document xmldoc=null;
try
{
WebServiceProxy proxy = new WebServiceProxy ();
String str = proxy.GetCustomerData();
str=str.replaceAll("<","<");
str=str.replaceAll(">",">");
byte[] bytes = str.getBytes("UTF8");
ByteArrayInputStream bais = new ByteArrayInputStream(bytes);
xmldoc = db.parse(bais);
}
catch(Exception e)
{
}
//Get the xsl file
String virtualpathLoginXSL = "customers.xsl";
String strRealPathLoginXSL = pageContext.getServletContext().getRealPath(virtualpathLoginXSL);
StreamResult htmlResult = new StreamResult(out);
StreamSource xslSource = new StreamSource(strRealPathLoginXSL);
TransformerFactory tf = TransformerFactory.newInstance();
Transformer tfmr = tf.newTransformer(xslSource);
//Parse XML to generate the html
tfmr.transform(new javax.xml.transform.dom.DOMSource(xmldoc),htmlResult);
}
catch(Exception e)
{
out.println("Error While Calling Web Services");
}
%>
7. Now run the tomacat and access the file WebServicesClient.jsp(e.g http://localhost:8080/ WebServicesClient/ WebServicesClient.jsp. You will see the same output as the .net client.
Classic ASP Client
Before you start building ASP client, make sure that Microsoft SOAP toolkit 3.0 and MSXML 4.0 installed in your computer. You can download them from Microsoft site (http://www.microsoft.com/downloads)
1. Open Microsoft Visual Interdev 6.0. File -> New Project -> Visual Interdev Project -> New Web Project. Name the Project (e.g. WebServiceClient).
2. In Project Explorer you will see the project node (e.g. localhost/ WebServiceClient), under which you will see the global.asa. add ASP file by right click on project->Add-> Active Server Page. Name the file as WebServiceClient.asp. And paste the below code in file.
// CODE WebServiceClient.asp
<%
'Soap Client
Dim oSOAP
'XML Document to laod XML fromˈweb Services
Dim objDOMDoc
'Node list
Dim objNodeList
'Child node list of above Modeˈlist
Dim objChildNodeList
'Create a SOAP client and callthe C# web services
Set oSOAP = Server.CreateObject("MSSOAP.SoapClient")
oSOAP.ClientProperty("ServerHTTPRequest") =
True
oSOAP.mssoapinit(
http://localhost/TestWebService/ThakurService.asmx?wsdl)
'Create XML document and load XML from Web services method
Set objDOMDoc = Server.CreateObject("Msxml2.DOMDocument.4.0")
objDOMDoc.loadXML(
CStr(oSOAP.GetCustomerData))
'Get the node list of product
Set objNodeList = objDOMDoc.selectNodes("AnandThakur/Products")
'write the output to the browser
Response.Write "<Table bgcolor='efefef' cellpadding='1' width='640' border='1'>"
Response.Write "<TR><TD><B>Contact Name</B></TD><TD><B>Company Name</B></TD></TR>"
'Get the data from nodelist
For Each objNode
In objNodeList
Response.Write "<TR>"
'Get data from Child elements
Set objChildNodeList = objNode.childNodes
For Each objChildNode
In objChildNodeList
Response.Write "<TD>" + objChildNode.Text + "</TD>"
Next
Response.Write "</TR>"
Next
Response.Write "</Table>"
%>
3. Right click on WebServiceClient.asp page and select Set as Start Page. And View in the browser, you will see the same output as .net and java client.
Conclusion
Web services are technologies designed to improve the interoperability between the many diverse application development platforms. Web service interoperability goals are to provide seamless and automatic connections from one software application to another. SOAP, WSDL, and UDDI protocols define a self-describing way to discover and call a method in a software application -- regardless of location or platform. We have seen here, how different clients (.net, java and ASP) can access the .net web services by different ways.
Aah! Another bug! Well, it's the life.