In this example we will build a web service that authenticates userid and password from an a very simple MS Access database. This web service exposes only one method to the client. This method takes input username and password, checks the values in the table, and if found returns the remaining fields from the table.
Create a database schema as shown below :
Begin creating a web service project on the VS development environment.
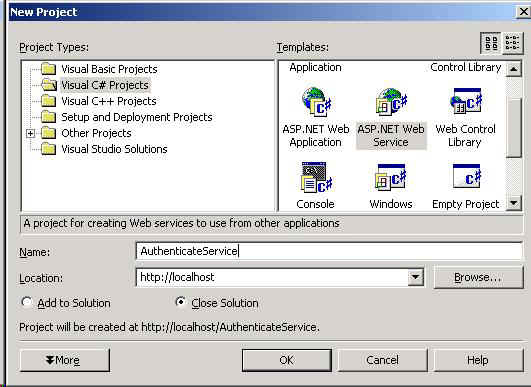
It creates a project under your webserver root folder http://localhost/AuthenticateService . This service creates a default file Service1.asmx. The .asmx extension is used to designate Web Services, while .aspx designates Web Forms, which could be used to access the Web Service.
Web service consists of methods that are exposed to the world via the [WebMethod] call before the function. Here is the example of the default Hello World method.
// WEB SERVICE EXAMPLE
// The HelloWorld() example service returns the string Hello World
// To build, uncomment the following lines then save and build the project
// To test this web service, press F5
// [WebMethod]
// public string HelloWorld()
// {
// return "Hello World";
// }
For our database example add a database connection object in the Constructor
private OleDbConnection conn;
public Service1()
{
//CODEGEN: This call is required by the ASP.NET Web Services Designer
InitializeComponent();
conn=new OleDbConnection("PROVIDER=Microsoft.Jet.OLEDB.4.0;Data Source=C:\\DB1.MDB");
conn.Open();
}
We will now add the new method that we wish to expose to the world . In this case the mentod takes the input (USERID and PASSWORD ), authenticates the user and if found returns back the user information in XML format.
[WebMethod(Description="Get Employee Info")]
public string GetUserInfo( string userId , string password )
{
string XMLFormat ;
string command = "select NAME , AGE , ADDRESS1 , ADDRESS2 , CITY , STATE , ZIP , TEL , EMLAI from CUSTOMER WHERE USERID = '" + userId + "' AND PASSWORD = '" + password + "'";
OleDbCommand cmd=new OleDbCommand(command,conn);
OleDbDataReader rdr;
rdr=cmd.ExecuteReader();
bool ret = rdr.Read();
if ( ret )
{
XMLFormat = "<DETAILS>\n";
XMLFormat += "<NAME>"+rdr.GetValue(0).ToString()+"</NAME>\n";
XMLFormat += "<AGE>"+rdr.GetValue(1).ToString()+"</AGE>\n";
XMLFormat += "<ADDRESS1>"+rdr.GetValue(2).ToString()+"</ADDRESS1>\n";
XMLFormat += "<ADDRESS2>"+rdr.GetValue(3).ToString()+"</ADDRESS2>\n";
XMLFormat += "<CITY>"+rdr.GetValue(4).ToString()+"</CITY>\n";
XMLFormat += "<STATE>"+rdr.GetValue(5).ToString()+"</STATE>\n";
XMLFormat += "<ZIP>"+rdr.GetValue(6).ToString()+"</ZIP>\n";
XMLFormat += "<TEL>"+rdr.GetValue(7).ToString()+"</TEL>\n";
XMLFormat += "<EMAIL>"+rdr.GetValue(8).ToString()+"</EMAIL>\n";
}
else
XMLFormat= "" ;
rdr.Close();
return XMLForat;
}
Its that simple. Compile the code and test the application( press the F5 Key to execute ) . When you execute the application , the system opens it in a web browser.