Introduction
In order to provide your application with cool pictures you can employ two techniques (at least). One of them is that you can save the pictures in a folder and store the path to each one in a database or configuration file. The other one is to store the entire file into a database, along with its file name.
Each of them has its ups and downs:
- If you save your files to a folder, you might accidentally delete a file from that folder. If this happens, you will end up with a broken "link" in your database or configuration file. However, the hard disk storage space is cheap, so you can afford to store a lot of files.
- If you store your files into a database, you can enforce security by using the security settings of the database. Also, there are no broken links ever. However, the database storage space is more expensive. Another idea is that you can save a thumbnail of the image on the database for quick access and to save the actual picture on hard drive.
Of course, each application has its particularities and you have to choose which one you will use. Ok. Enough of the philosophical talk! Let's dive into the good stuff.
The application
The problem of uploading files to a database is not that difficult. You have to use, on the server, a certain data type when creating the table. This data type has to be capable of storing large amounts of binary data. When using Microsoft SQL Server, this data type is called image. For more information see BLOB (Binary Large OBject) for a short definition and Books Online for a complete reference.
The client has to obtain the data from the file in binary format - a byte array - and to call a procedure on the server with that array as a parameter.
In this presentation I assume that I have a database Pictures on the server with a table called Pictures. The structure of this table is as follows
Field Name |
Field Type |
kFileName |
Long |
Picture |
Image |
FileName |
Varchar(250) |
I also have stored procedures for uploading, downloading and retrieving the list of uploaded files. These procedures are shown below:
Procedure Name |
Procedure body |
UploadFile |
CREATE PROCEDURE [dbo].[UploadFile] ( @Picture image, @FileName varchar(250), @kFileName bigint output ) AS insert into Pictures (Picture, FileName) values (@Picture,@FileName) set @kFileName = @@IDENTITY GO |
DownloadFile |
CREATE PROCEDURE [dbo].[DownloadFile] ( @kFileName bigint, @FileName varchar(250) output ) AS select Picture, FileName from Pictures where kFileName=@kFileName GO |
getUploadedFiles |
CREATE PROCEDURE [dbo].[getUploadedFiles] AS select ltrim(str(kFileName)) + " - " + FileName as Name from Pictures GO |
The code presented below was written using C# and was tested in Visual Studio .NET 2003. The client code that gets the byte array from the file and calls these procedures is shown below.
using
System;
using System.IO;
using System.Data;
using System.Text;
using System.Data.SqlClient;
/*
* Autor: Ghiondea Alexandru
* Date: 08 october 2004
* Description: Implements methods for uploading and downloading files
* with MS SQL Server *
*/
namespace PicturesInSQLServer
{
/// <summary>
/// This class manages uploads and downloads to and from an SQL Server
/// </summary>
public class TransferPictures
{
/// <summary>
/// Gets from the server a list of uploaded files into a dataSet
/// </summary>
/// <param name="ds">The dataset</param>
/// <param name="table">The table in the dataset</param>
public void GetUploadedFiles(ref DataSet ds, string table)
{
//
// The variables required for connecting to the server.
//
SqlConnection conn =null;
SqlCommand cmd = null;
SqlDataAdapter da = null;
// ----------------------------------------------
try
{
//
// If the table already exists, cleares its content. Else adds a new table.
//
if (ds.Tables.Contains(table))
ds.Tables[table].Clear();
else
ds.Tables.Add(table);
// ----------------------------------------------
//
// Creates a connection to the database and initilizes the command
//
conn = new SqlConnection(ConnectionString());
cmd = new SqlCommand("getUploadedFiles",conn);
cmd.CommandType = CommandType.StoredProcedure;
// ----------------------------------------------
//
// Initializes the DataAdapter used for retrieving the data
//
da = new SqlDataAdapter(cmd);
// ----------------------------------------------
//
// Opens the connection and populates the dataset
//
conn.Open();
da.Fill(ds,table);
conn.Close();
// ----------------------------------------------
}
catch(Exception e)
{
//
// If an error occurs, we assign null to the result and display the error to the user,
// with information about the StackTrace for debugging purposes.
//
Console.WriteLine(e.Message + " - " + e.StackTrace);
}
}
/// <summary>
/// Uploads a file to the database server.
/// </summary>
/// <param name="fileName">The filename of the picture to be uploaded</param>
/// <returns>The id of the file on the server.</returns>
public long UploadFile(string FileName)
{
if (!File.Exists(FileName))
{ return -1; }
FileStream fs=null;
try
{
#region Reading file
fs = new FileStream(FileName,FileMode.Open);
//
// Finding out the size of the file to be uploaded
//
FileInfo fi = new FileInfo(FileName);
long temp = fi.Length;
int lung = Convert.ToInt32(temp);
// ------------------------------------------
//
// Reading the content of the file into an array of bytes.
//
byte[] picture=new byte[lung];
fs.Read(picture,0,lung);
fs.Close();
// ------------------------------------------
#endregion
long result = uploadFileToDatabase(picture,fi.Name);
return result;
}
catch(Exception e)
{
Console.WriteLine(e.Message + " - " + e.StackTrace);
return -1;
}
}
/// <summary>
/// Wrapper for downloading a file from a database.
/// </summary>
/// <param name="kFileName">The Unique ID of the file in database</param>
/// <param name="fileName">The file name as it was stored in the database.</param>
/// <returns>The byte array required OR null if the ID is not found</returns>
public byte[] DownloadFile(long kFileName, ref string fileName)
{
byte[] result = downloadFileFromDatabase(kFileName, ref fileName);
return result;
}
/// <summary>
/// Returns the connection string for connecting to the database
/// </summary>
/// <returns>The Connection string.</returns>
public static string ConnectionString()
{
//
// We consider that the database is situated on the same computer that runs the program.
// To connect to a remote server, replace 'Data Source' with the name of that server.
//
return "Connect Timeout=600;Integrated Security=SSPI;Persist Security Info=False;Initial Catalog=Pictures;Packet Size=4096;Data Source=" + System.Environment.MachineName.Trim(); }
/// <summary>
/// Uploades a file to an SQL Server.
/// </summary>
/// <param name="picture">A byte array that contains the information to be uploaded.</param>
/// <param name="fileName">The file name asociated with that byte array.</param>
/// <returns>The unique ID of the file on the server OR -1 if an error occurs. </returns>
private long uploadFileToDatabase(byte[] picture, string fileName)
{
//
// Defining the variables required for accesing the database server.
//
SqlConnection conn = null;
SqlCommand cmd =null;
SqlParameter kFileName =null;
SqlParameter FileName =null;
SqlParameter pic =null;
// By default, we assume we have an error. If we succed in uploading the file, we'll change this
// to the unique id of the file
long result=-1;
try
{
//
// Connecting to database.
//
conn = new SqlConnection(ConnectionString());
cmd = new SqlCommand("UploadFile",conn);
// We assume there is a stored procedure called UploadFile
cmd.CommandType = System.Data.CommandType.StoredProcedure;
// ----------------------------------------------
//
// Initializing parameters and assigning the values to be sent to the server
//
kFileName = new SqlParameter("@kFileName",System.Data.SqlDbType.BigInt,8); kFileName.Direction = ParameterDirection.Output;
// This parameter does not have a size because we do not know what the size is going to be.
pic = new SqlParameter("@picture",SqlDbType.Image);
pic.Value = picture;
FileName = new SqlParameter("@FileName",SqlDbType.VarChar,250);
FileName.Value = fileName;
// ----------------------------------------------
//
// Adding the parameters to the database. Remember that the order in which the parameters
// are added is VERY important!
//
cmd.Parameters.Add(pic);
cmd.Parameters.Add(FileName);
cmd.Parameters.Add(kFileName);
// ----------------------------------------------
//
// Opening the connection and executing the command.
//
conn.Open();
cmd.ExecuteNonQuery();
conn.Close();
// ----------------------------------------------
//
// The result is the unique identifier created on the database.
// result = (long)kFileName.Value;
// ----------------------------------------------
//
// Disposing of the objects so we don't occupy memory.
// conn.Dispose(); cmd.Dispose();
// ----------------------------------------------
}
catch(Exception e)
{
//
// If an error occurs, we report it to the user, with StackTrace for debugging purposes
//
Console.WriteLine(e.Message + " - " + e.StackTrace);
result = -1;
// ----------------------------------------------
}
return result;
}
/// <summary>
/// Downloades a file from a database according to the unique id in that database.
/// </summary>
/// <param name="kFile">The ID of the file in the database</param>
/// <param name="FileName">The filename of the file as it was stored in the database.</param>
/// <returns>A byte array containing the information required.</returns>
private byte[] downloadFileFromDatabase(long kFile, ref string FileName)
{
SqlConnection conn =null;
SqlCommand cmd = null;
SqlParameter kFileName = null;
SqlParameter fileName = null;
SqlDataReader dr=null;
byte[] result=null;
try
{
//
// Connecting to database.
//
conn = new SqlConnection(ConnectionString());
cmd = new SqlCommand("DownloadFile",conn);
cmd.CommandType = System.Data.CommandType.StoredProcedure;
// ----------------------------------------------
//
// Initializing parameters and assigning the values to be sent to the server
// kFileName= new SqlParameter("@kFileName",System.Data.SqlDbType.BigInt,8); kFileName.Value = kFile;
fileName = new SqlParameter("@FileName",SqlDbType.VarChar,250);
fileName.Direction = ParameterDirection.Output;
// ----------------------------------------------
//
// Adding the parameters to the database. Remember that the order in which the parameters
// are added is VERY important!
//
cmd.Parameters.Add(kFileName);
cmd.Parameters.Add(fileName);
// ----------------------------------------------
//
// Opening the connection and executing the command.
// The idea behind using a dataReader is that, on the SQL Server, we cannot assign to a
// variable the value of an image field. So, we use a querry to select the record we want
// and we use a datareader to read that query.
// Because we are returnig information based on a primary key, we are always returning
// only one row of data.
//
conn.Open();
dr = cmd.ExecuteReader();
dr.Read();
//
// We are casting the value returned by the datareader to the byte[] data type.
//
result = (byte[])dr.GetValue(0);
//
// We are also returning the filename associated with the byte array.
// FileName = (string)dr.GetValue(1);
//
// Closing the datareader and the connection
//
dr.Close();
conn.Close();
// ------------------------------------------
//
// Disposing of the objects so we don't occupy memory.
// conn.Dispose(); cmd.Dispose();
// ------------------------------------------
}
catch(Exception e)
{
//
// If an error occurs, we assign null to the result and display the error to the user,
// with information about the StackTrace for debugging purposes.
// Console.WriteLine(e.Message + " - " + e.StackTrace);
result = null;
}
return result;
}
}
}
I have also written a small application to demonstrate how to use these methods. A screenshot of it is shown below.
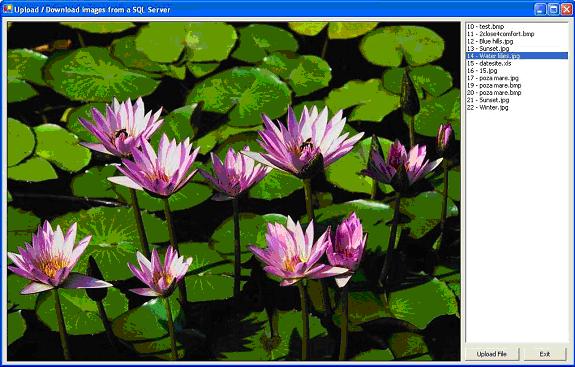
Here are some snippets of relevant code for this application:
private
void UploadedFiles_DoubleClick(object sender, System.EventArgs e)
{
//
// Finds the unique id of the file.
//
DataRowView drv = (DataRowView) UploadedFiles.SelectedItem;
string selectedText = drv.Row["Name"].ToString();
long id=-1;
id = long.Parse(selectedText.Substring(0,selectedText.IndexOf(" - ",0)).Trim());
string filename=null;
TransferPictures up = new TransferPictures();
byte[] result = up.DownloadFile(id,ref filename);
up = null;
try
{
//
// We cannot assign a byte array directly to an image.
// We use MemoryStream, an object that creates a file in memory
// and than we pass this to create the image object.
//
MemoryStream ms= new MemoryStream(result,0,result.Length);
Image im = Image.FromStream(ms);
Picture.Image = im;
}
catch(Exception ee)
{
MessageBox.Show("An error has occured.\n" + ee.Message);
}
}
private void UploadFile_Click(object sender, System.EventArgs e)
{
//
// Gets the file to be uploaded
//
OpenFileDialog ofd = new OpenFileDialog();
ofd.ShowDialog();
if (ofd.FileName=="" || !File.Exists(ofd.FileName))
{
//
// If the requested file is not ok...
//
return;
}
TransferPictures up = new TransferPictures();
long id =up.UploadFile(ofd.FileName);
string msg=null;
if (id >0)
{
msg = "Upload succesful"; LoadInformationFromDataBase();
}
else
{
msg = "An error has occured";
}
MessageBox.Show(msg);
}
private void LoadInformationFromDataBase()
{
TransferPictures up = new TransferPictures();
up.GetUploadedFiles(ref ds,"Pictures");
UploadedFiles.DataSource = ds.Tables["Pictures"];
UploadedFiles.DisplayMember = "Name";
}
private void frmMain_Load(object sender, System.EventArgs e)
{
LoadInformationFromDataBase();
}
Conclusion
Well, choosing which type of image - file - storage technique is up to the person designing a specific application. I have tried here to show you how you can store them in a database.