Introduction
Trackbars and scrollbars are convenient ways of displaying a data range. The trackbars and scrollbars that ship with Visual Studio .NET, however, are a little lacking in aesthetics. We at Mycos set out to develop a high-performance dynamic trackbar that tool advantage of the GDI+ api and some of our existing drawing utilities. The end-result looks something like the following image. At runtime, of course, it allows you to drag and drop the filled area, just as with any normal trackbar. The value property is public and can be used to get the current setting at any time.
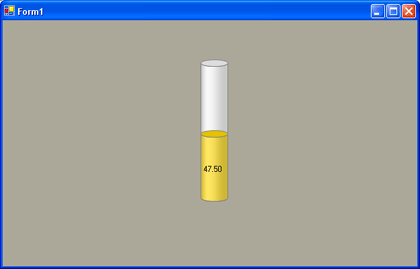
Method #1: DrawBar
This method does the bulk of the heavy lifting with a little help from the DrawingUtils class. Both classes are included in the sample source. The properties that are exposed control through the property grid include control color, anti-aliasing, and max and min values.
private void DrawBar(Graphics g, Color foreColor)
{
bool outLine = this.outLineColor != Color.Transparent;
Rectangle bound = this.ClientRectangle;
bound.Inflate(-20, -20);
Mycos.Glib.DrawingUtils.FillCylinder(g, bound, new Size(4, 10),
Color.FromArgb(this.Alpha, foreColor), this.OutLineColor, outLine);
if ( this.Value > this.Minimum && this.Value <= this.Maximum )
{
float barValue = bound.Height * (( this.Value - this.Minimum )
/ ( this.Maximum - this.Minimum));
RectangleF valueBound = RectangleF.FromLTRB(bound.Left,
bound.Bottom - barValue, bound.Right, bound.Bottom); Mycos.Glib.DrawingUtils.FillCylinder(g, valueBound, new Size(4, 10), Color.FromArgb(this.Alpha, this.BarColor), this.OutLineColor, outLine);
if ( this.showValue && valueBound.Height > 20 )
{
g.SmoothingMode = SmoothingMode.AntiAlias;
StringFormat format = new StringFormat();
format.Alignment = StringAlignment.Far;
format.LineAlignment = StringAlignment.Center;
g.DrawString(this._value.ToString("F2"), this.Font,Brushes.Black, valueBound, format);
format.Dispose();
}//end if Show Value
}//end in Length
}
#endregion
Method #2: FillCylinder
This method fills the cylinder given a handle to its graphics object. It would be quite simple to rework this for other cylinder types. It is found in the class, DrawingUtils.
public
static void FillCylinder(Graphics g, RectangleF front, SizeF depth, Color fillColor, Color borderColor, bool outLine)
{
if ( front.Width <= 0 || front.Height <= 0 )
return;
// Make Back Side Area
RectangleF aback = front;
// Make Depth
aback.X += depth.Width;
aback.Y -= depth.Height;
// Create Top and Bottom Plane.
RectangleF bottomPlane;
RectangleF topPlane;
// Create Graphics Object
GraphicsPath gp = new GraphicsPath();
topPlane = new RectangleF((front.X+aback.X)/2, aback.Location.Y, front.Width, front.Y - aback.Y);
bottomPlane = new RectangleF(new PointF((front.X+aback.X)/2, aback.Bottom), new SizeF(front.Width, front.Y - aback.Y ));
// Brush
SolidBrush brush = new SolidBrush(fillColor);
// Border Pen
Pen borderPen = new Pen(borderColor);
// Make GP On Bottom
gp.AddEllipse(bottomPlane);
// Get Bottom color
brush.Color = GetSideColor(fillColor, WallSide.Bottom);
// Fill Bottom Plane
g.FillPath(brush, gp);
// Check Draw Border
if ( outLine )
g.DrawPath(borderPen, gp);
gp.Reset();
gp.AddArc(topPlane, 0, 180);
gp.AddLine(gp.GetLastPoint(), new PointF((front.X+aback.X)/2, (front.Bottom+aback.Bottom)/2.0f));
gp.AddArc(bottomPlane, 180, -180);
gp.CloseFigure();
// Color For Body is real Fill Color.
brush.Color = fillColor;
// Fill Body
g.FillPath(brush, gp);
// Shadow of the Body
FillCylinderShadow(g, front, gp, false);
// Check Draw Border
if ( outLine )
g.DrawPath(borderPen, gp);
/***************
* Top *
* ************/
gp.Reset();
gp.AddEllipse(topPlane);
// Get Bottom color
brush.Color = GetSideColor(fillColor, WallSide.Top);
// Fill Top Plane
g.FillPath(brush, gp);
// Check Draw Border
if ( outLine )
g.DrawPath(borderPen, gp);
// Dispose
gp.Dispose();
brush.Dispose();
borderPen.Dispose();
}//End Method Fill
Conclusions
It doesn't take too much code to wake up the view of a Windows Forms application and hopefully this will get you started along the right path.
Acknowledgements
Thanks to Jirapong Nanta here at Mycos for implementing the control in under 10 minutes and listening to my crazy ideas all the time.