Introduction
Enumerations (enums) in C# provide a convenient way to define a set of named values. However, there are scenarios where we must represent multiple values or combinations of values using a single enum variable. This is where bitwise enums come into play. Bitwise enums in C# allow for efficient and flexible flag-based enumerations, enabling us to store and manipulate multiple values in a compact form. In this article, we will delve into the concept of bitwise enums and explore how to use them effectively in C#.
What is Bitwise Enums?
A bitwise enum is an enum type that uses bitwise operations to combine multiple enum values into a single value. Each enum value is assigned a unique bit flag, represented by a power of 2. By assigning these flags, we can represent multiple enum values by combining or masking them using bitwise operators.
How do we define Bitwise Enums?
To define a bitwise enum, we need to decorate it with the [Flags] attribute, indicating that the enum values can be combined using bitwise operations. Here's an example,
[Flags] enum DaysOfWeek {
None = 0, Monday = 1, Tuesday = 2, Wednesday = 4, Thursday = 8, Friday = 16,
Saturday = 32, Sunday = 64
};
In the above code, we define a DaysOfWeek enum where each day of the week is assigned a unique power of 2. The None value is assigned 0, indicating no days are selected.
Combining Bitwise Enums
To combine multiple enum values, we use the bitwise OR (|) operator. Here's an example,
DaysOfWeek selectedDays = DaysOfWeek.Monday | DaysOfWeek.Wednesday | DaysOfWeek.Friday;
The above code combines the Monday, Wednesday, and Friday enum values using the bitwise OR operator.
Checking for Enum Values
To check if a specific enum value is set in a bitwise enum, we use the bitwise AND (&) operator. Here's an example:
Console.WriteLine("selected Days", selectedDays);
if ((selectedDays & DaysOfWeek.Monday) != 0) {
Console.WriteLine("Monday is selected.");
}
selectedDays ^= DaysOfWeek.Wednesday;
In the above code, we check if the Monday value is set in the selectedDays variable using the bitwise AND operator.
Removing Enum Values
To remove a specific enum value from a bitwise enum, we use the bitwise XOR (^) operator. Here's an example:
selectedDays ^= DaysOfWeek.Wednesday;
Console.WriteLine(selectedDays);
In the above code, we remove the Wednesday value from the selectedDays variable using the bitwise XOR operator.
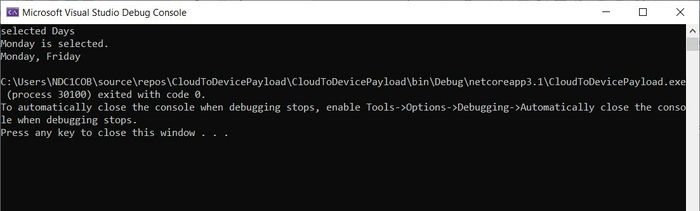
Conclusion
Bitwise enums in C# provide a powerful mechanism for representing and manipulating flag-based enumerations efficiently. By assigning unique bit flags to enum values and utilizing bitwise operations, we can combine, check, and remove specific enum values within a compact representation. Whether representing days of the week, permissions, or other flag-based scenarios, bitwise enums offer flexibility and convenience. With a solid understanding of bitwise enums, you can leverage this feature in your C# projects to handle complex flag-based scenarios effectively.